The Block Directory and Class in Magento 2
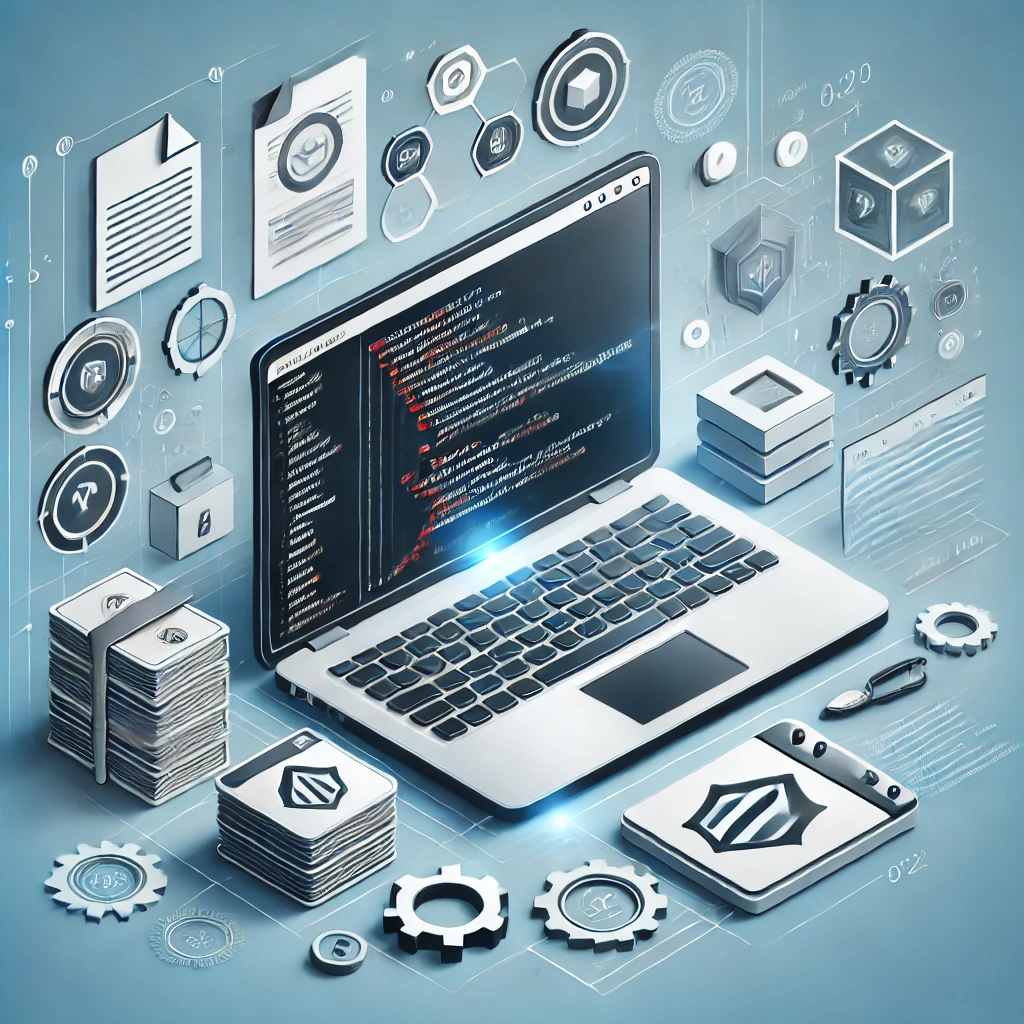
Hello there! Let’s dive into one of the core pillars of Magento 2: the Block directory and class. Whether you’re new to Magento or looking to deepen your understanding, we’ll explore these concepts in a friendly, approachable way. I’ll break everything down so you feel confident navigating and using Blocks in your projects.
1. What Are Blocks in Magento 2?
Let’s start with the basics. Blocks in Magento 2 are a key component of the MVC (Model-View-Controller) architecture. They serve as the middle layer between the business logic (Models) and the presentation layer (Templates).
Key Characteristics of Blocks:
- Purpose: Provide data to templates and manipulate that data as needed.
- Location: Reside in the
Block
directory of a module. - Flexibility: Allow developers to handle dynamic content, fetch data from models, and pass it to templates.
Think of Blocks as the glue that binds your PHP logic with your frontend HTML. Without Blocks, templates would lack a mechanism to retrieve dynamic data.
2. The Role of the Block Directory
Every Magento 2 module has a Block directory, typically located at:
The Block directory holds PHP classes that extend the functionality of Magento’s core AbstractBlock
. These classes are the foundation for linking layout XML configurations and templates.
Why Do We Need a Block Directory?
- Organization: It provides a structured way to manage PHP classes associated with frontend rendering.
- Reusability: Blocks can be used across multiple templates within a module or even across different modules.
- Customization: Developers can override or extend core Block functionality to tailor it to business requirements.
3. Anatomy of a Block Class
At its core, a Block class is a PHP file that extends \Magento\Framework\View\Element\Template
or one of its specialized subclasses. Here’s a typical structure:
Example Block Class:
Key Elements:
- Namespace: Matches the module’s directory structure.
- Class Name: Descriptive and meaningful (e.g.,
ExampleBlock
). - Dependency Injection (DI): Allows us to inject other classes like helpers, services, or repositories.
- Methods: Contain logic to fetch or manipulate data that templates use.
4. How Blocks Interact with Layout XML
Now that we’ve covered what Blocks are, let’s explore their connection to layout XML. This is where the magic happens — tying together the Block, template, and layout.
Typical Layout XML Structure:
- class: Specifies the PHP class to use for the Block.
- name: A unique identifier for the Block within the layout.
- template: Points to the
.phtml
file for rendering.
Workflow:
- The layout XML tells Magento to instantiate the Block class.
- Magento assigns the template specified in the layout.
- The Block class prepares data, which the template then renders.
Where Is Layout XML Defined?
- Module-Specific:
app/code/Vendor/Module/view/frontend/layout/<layout_name>.xml
- Theme-Specific:
app/design/frontend/<Vendor>/<Theme>/Magento_Module/<layout_name>.xml
5. Creating a Custom Block in Magento 2
Let’s walk through a practical example. Imagine you need to display a list of featured products on your homepage.
Step 1: Define the Block Class
Create a new PHP class in app/code/Vendor/Module/Block/FeaturedProducts.php
:
Step 2: Add Layout XML
Define the Block in a layout file, such as cms_index_index.xml
:
Step 3: Create the Template
Add a template file at app/code/Vendor/Module/view/frontend/templates/featured-products.phtml
:
Step 4: Clear Cache and Test
Run the following commands:
Visit your homepage, and voilà! Your featured products are displayed.
6. Real-Life Examples of Block Usage
Here are some common scenarios where Blocks shine in Magento 2:
1. Dynamic Menus
- Blocks can generate menus dynamically by fetching categories and rendering them in the template.
2. Custom Forms
- Use a Block to fetch form data or validation rules and pass them to the template.
3. Product Listings
- Blocks retrieve product collections and customize how products are displayed.
4. Integrations
- Blocks act as the bridge to fetch data from external APIs and render it in templates.
7. Tips and Best Practices
Here are a few tips to keep your Magento 2 Blocks efficient and maintainable:
1. Keep Logic in the Block
Avoid placing business logic in templates. Instead, use Blocks to prepare data and pass it to templates.
2. Use Dependency Injection
Inject dependencies into Blocks rather than instantiating them directly. This ensures better testability and adheres to Magento’s architecture principles.
3. Leverage Core Classes
Before creating a custom Block, check if Magento’s core Blocks meet your needs. Extending or overriding them can save time.
4. Organize Blocks
Group related Block classes in subdirectories within the Block
directory for better maintainability.
5. Comment Your Code
Document your Block methods to help other developers (and future you!) understand their purpose.
8. Conclusion
The Block directory and class are indispensable components of Magento 2, enabling you to connect the backend logic with the frontend presentation. Understanding their structure and role not only enhances your development skills but also ensures cleaner, more efficient code.
Remember, Blocks are like your backstage crew—they ensure the show runs smoothly, even if the audience (your users) never sees them.
I hope this guide has been helpful! If you have any questions or want to share your experiences, feel free to reach out. Happy coding!