Understanding Helpers in Magento 2
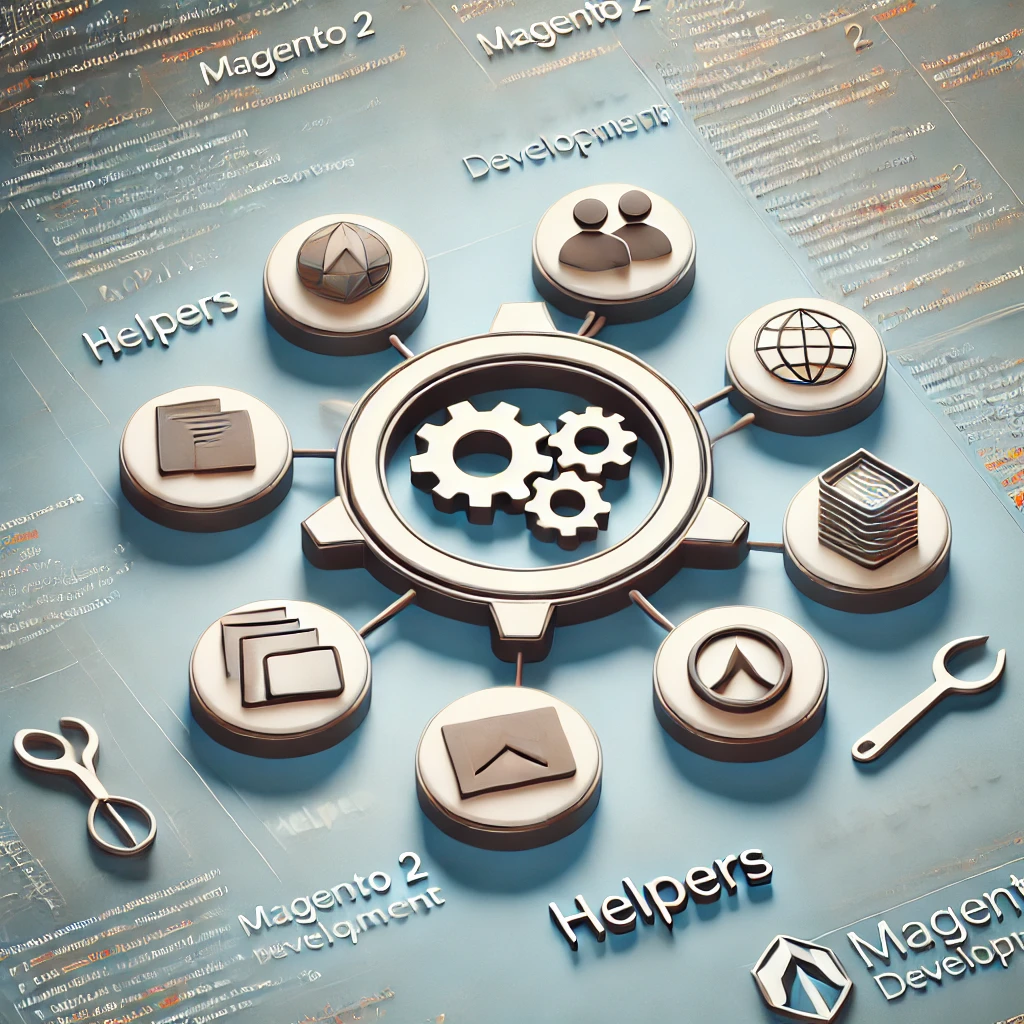
1. What Are Helpers in Magento 2?
Helpers are PHP classes in Magento 2 that provide utility functions for repetitive or complex operations. These classes are not tied to any specific part of the application (e.g., Models, Controllers, etc.) and can be used globally within your module.
Key Characteristics:
- Reusable: Helpers reduce code duplication by centralizing common functions.
- Accessible: You can use helpers in Blocks, Controllers, Models, and Templates.
- Lightweight: Helpers are designed to be small, utility-focused classes, without holding state or handling large operations.
Think of helpers as your "Swiss Army Knife" for simplifying tasks that don’t belong to any specific layer of the Magento 2 architecture.
2. The Role of Helpers
Why Use Helpers?
- Code Efficiency: By encapsulating repetitive logic into helpers, you streamline your codebase.
- Centralized Logic: Changes made in a helper are reflected wherever it’s used, ensuring maintainability.
- Simplified Data Handling: Helpers can process data before sending it to templates or other application layers.
When to Use Helpers:
- To format prices, dates, or other data for consistent output.
- To retrieve Magento configuration values.
- To perform calculations or conversions.
- To create utility functions for specific module features.
3. Default Magento 2 Helpers
Magento 2 comes with several built-in helpers that you can use right away. These helpers are located in the Magento\Framework
namespace.
Commonly Used Magento 2 Helpers:
-
Data Helper (
\Magento\Framework\App\Helper\AbstractHelper
)- Provides core utility functions like fetching configurations and URLs.
- Example: Retrieve a configuration value:
-
Price Helper (
\Magento\Framework\Pricing\Helper\Data
)- Formats prices for display.
- Example:
-
Url Helper (
\Magento\Framework\Url\Helper\Data
)- Generates or parses URLs.
-
File Helper (
\Magento\Framework\File\Uploader
)- Assists with file uploads.
Pro Tip:
Before creating a custom helper, explore the default helpers provided by Magento. They often meet common requirements out of the box.
4. Creating Custom Helpers
Creating a custom helper in Magento 2 involves defining a class in the Helper
directory of your module.
Step 1: Create the Helper Class
In app/code/Vendor/Module/Helper
, create a file CustomHelper.php
:
Step 2: Use the Helper in Your Code
Inject the helper in any class where it’s needed:
Step 3: Test the Helper
Use the methods defined in your custom helper to simplify operations in your module.
5. Using Helpers in Blocks, Controllers, and Templates
Using Helpers in Blocks
Blocks are a common place to use helpers for preparing data for templates.
Using Helpers in Controllers
Helpers can simplify logic in Controllers by abstracting utility functions.
Using Helpers in Templates
To use a helper in .phtml
files, access it via the Block:
6. Real-World Examples of Helpers
1. Formatting Data for Templates
Imagine you’re working on an order module and need to format the delivery date:
2. Fetching Configuration Values
Suppose you have a configuration field in the admin panel. Use a helper to retrieve it:
3. Generating URLs
For custom URL generation, use Magento’s URL helper or extend it in your custom helper.
7. Best Practices for Working with Helpers
1. Limit Helper Scope
Avoid putting too much logic into helpers. Helpers should remain utility-focused and lightweight.
2. Leverage Dependency Injection
Always use Dependency Injection to access helpers instead of instantiating them directly.
3. Avoid Overusing Helpers
If the logic requires multiple dependencies or is too complex, consider using a service class instead of a helper.
4. Document Helper Methods
Provide clear comments and method descriptions to ensure other developers understand their purpose.
8. Conclusion
Helpers in Magento 2 are invaluable for simplifying repetitive tasks, maintaining cleaner code, and centralizing logic that doesn’t belong in other parts of the MVC structure. By leveraging both default and custom helpers effectively, you can significantly enhance your development workflow.
Whether you’re formatting data, fetching configuration values, or simplifying template logic, helpers are there to make your life easier. Just remember to keep them focused, lightweight, and reusable!