The Controller Directory in Magento 2
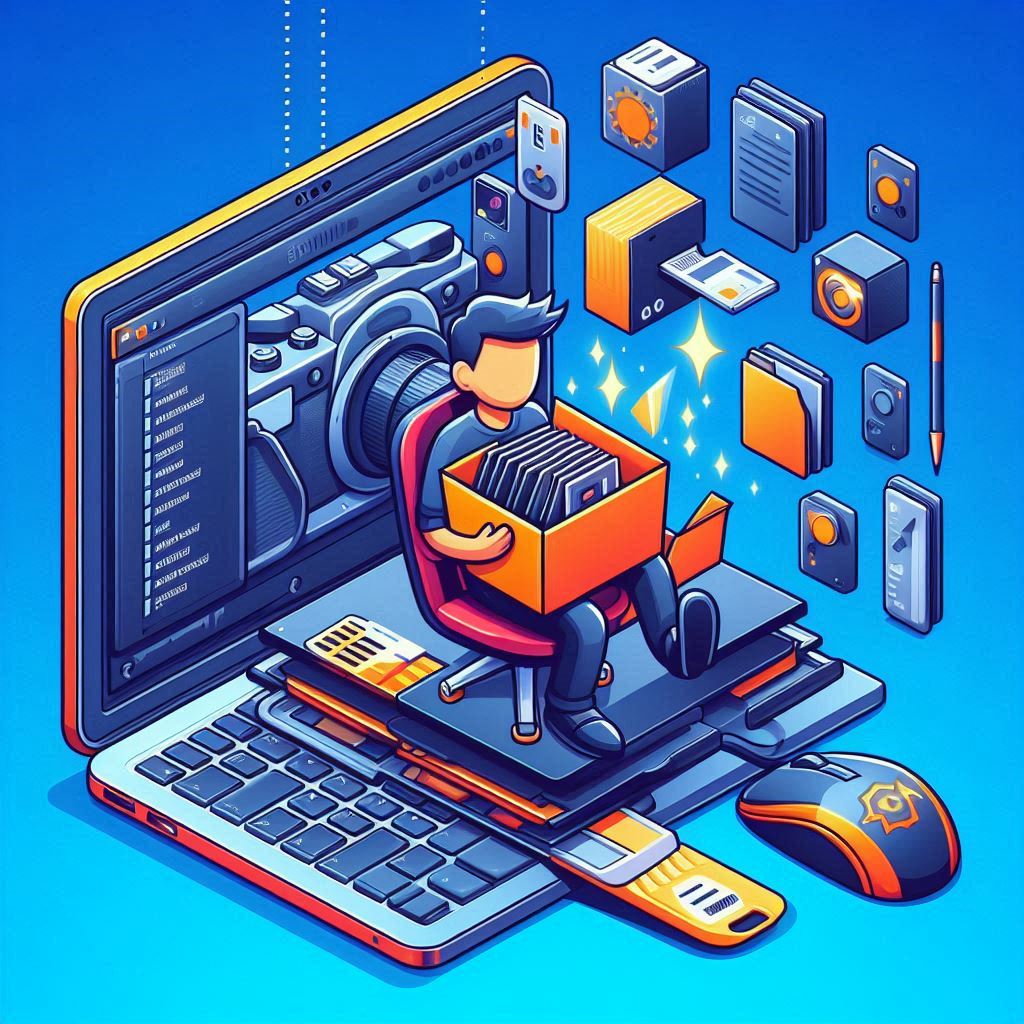
What is the "Controller" Directory in Magento 2?
In Magento 2, the "Controller" directory plays an important role in handling requests and rendering responses for the application. You can think of it as a bridge between the requests users make and the behind-the-scenes logic that processes those requests. It helps determine how the application responds to different types of HTTP requests.
In this article, we'll break down the structure and function of the "Controller" directory, with examples to show how it works in practice.
What Does the Controller Directory Contain?
The Controller directory is located in Magento 2 modules at app/code/{Vendor}/{Module}/Controller
. It holds PHP classes that act as controllers. These controllers are responsible for processing incoming HTTP requests and deciding how the application should respond.
Generally, controllers handle two types of requests:
- Frontend Requests – These are requests coming from the public-facing side of your Magento store, where customers browse products.
- Adminhtml Requests – These are requests from the backend, where store administrators manage products, orders, customers, etc.
Frontend vs. Adminhtml Controllers
Magento 2 divides controllers into two main types based on where they are used:
- Frontend Controllers: These controllers handle requests related to the customer-facing pages. You can find them in
app/code/{Vendor}/{Module}/Controller/Index/
.
namespace Vendor\Module\Controller\Index; use Magento\Framework\App\Action\Action; use Magento\Framework\App\Action\Context; class Example extends Action { public function __construct(Context $context) { parent::__construct($context); } public function execute() { // Logic here echo 'This is a front-end controller!'; } }
- Adminhtml Controllers: These handle admin-side requests and can be found in
app/code/{Vendor}/{Module}/Controller/Adminhtml/
.
namespace Vendor\Module\Controller\Adminhtml\Index; use Magento\Backend\App\Action; use Magento\Backend\App\Action\Context; class Example extends Action { public function __construct(Context $context) { parent::__construct($context); } public function execute() { // Admin-side logic echo 'This is an admin controller!'; } }
Both frontend and adminhtml controllers extend different base classes:
- Frontend controllers extend
\Magento\Framework\App\Action\Action
, while - Adminhtml controllers extend
\Magento\Backend\App\Action
.
How Do Controllers Work?
Each controller class has an execute()
method that defines the logic to be performed when a request hits that controller. The flow typically works like this:
- A user requests a URL.
- Magento routes the request to the appropriate module and controller.
- The controller's
execute()
method is triggered. - The response is generated and displayed to the user.
For example, if a user visits the URL http://yourstore.com/yourmodule/index/example
, Magento will:
- Use the router configuration (defined in the module’s
routes.xml
file) to locate theIndex/Example
controller. - Call the
execute()
method in that controller. - Return the result (a rendered page, a redirect, JSON data, etc.).
Adding Logic to Your Controller
You can add any custom logic inside the execute()
method, such as fetching data from the database, modifying session variables, or rendering custom views.
Here’s an example of a controller that redirects to a custom URL:
public function execute() {
return $this->_redirect('custom/url');
}
Or, here’s one that returns a JSON response:
use Magento\Framework\Controller\Result\JsonFactory;
class Example extends \Magento\Framework\App\Action\Action
{
protected $resultJsonFactory;
public function __construct(Context $context, JsonFactory $resultJsonFactory) {
$this->resultJsonFactory = $resultJsonFactory;
parent::__construct($context);
}
public function execute() {
$data = ['message' => 'Hello, World!'];
$result = $this->resultJsonFactory->create();
return $result->setData($data);
}
}
Controller URL Structure
Magento 2 uses a convention-based URL structure to route requests to the appropriate controller. The general URL structure is:
{base_url}/{frontname}/{controller}/{action}/{params}
For example, http://yourstore.com/yourmodule/index/example
maps to the Index/Example.php
controller in the Vendor_Module
module.
Conclusion
Controllers are a key part of developing modules in Magento 2. By managing how HTTP requests are handled, they give developers a flexible and powerful way to create custom functionality on both the frontend and backend of Magento stores. Understanding the Controller directory is crucial for creating robust and scalable Magento 2 extensions.