How to Create Custom REST APIs in Magento 2
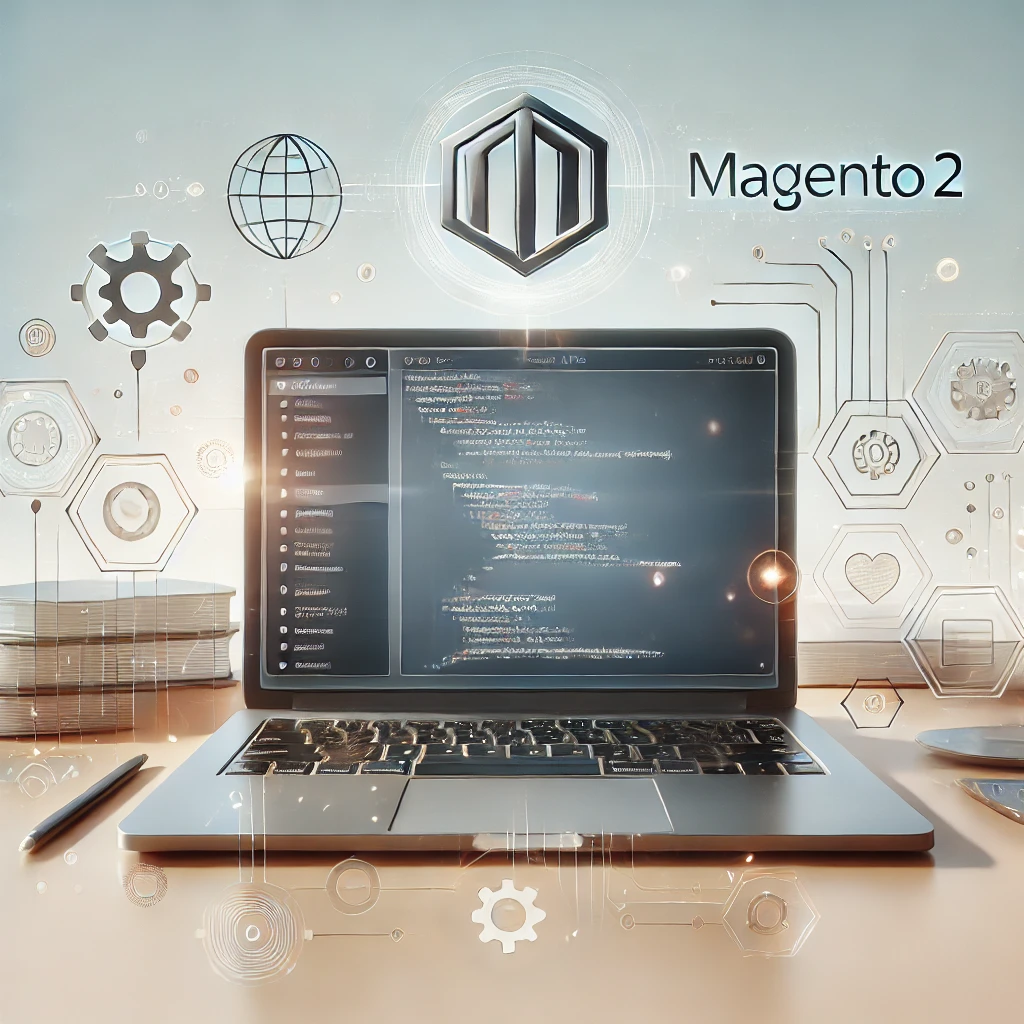
How to Create Custom REST APIs in Magento 2
If you're diving into Magento 2 development, you'll quickly realize that REST APIs are a powerful tool for integrating your store with external systems, mobile apps, or even custom frontends. Creating custom REST APIs in Magento 2 might sound intimidating at first, but once you get the hang of it, it’s pretty straightforward. Let’s break it down step by step, so you can build your own APIs like a pro.
What Are REST APIs in Magento 2?
REST (Representational State Transfer) APIs are a way for different systems to communicate over HTTP. In Magento 2, REST APIs allow you to perform CRUD (Create, Read, Update, Delete) operations on your store’s data, such as products, customers, orders, and more. By creating custom REST APIs, you can extend Magento’s functionality to meet your specific business needs.
Why Create Custom REST APIs?
Custom REST APIs are useful when:
- You need to integrate Magento with third-party systems like ERPs or CRMs.
- You’re building a mobile app that interacts with your Magento store.
- You want to expose specific data or functionality to external applications.
Now, let’s get into the nitty-gritty of creating a custom REST API in Magento 2.
Step 1: Set Up Your Magento 2 Module
First, you’ll need to create a custom module. If you’re not familiar with Magento 2 module structure, don’t worry—it’s simpler than it sounds. Here’s how to set it up:
- Create the module directory structure:
- Add the
registration.php
file: - Add the
module.xml
file in theetc
directory:
app/code/Vendor/ModuleName
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_ModuleName',
__DIR__
);
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_ModuleName" setup_version="1.0.0"/>
</config>
Once your module is set up, enable it by running:
php bin/magento setup:upgrade
Step 2: Define Your API Interface
Next, you’ll define the interface for your API. This interface will specify the methods that your API will expose. Create a file called Api/YourServiceInterface.php
in your module:
<?php
namespace Vendor\ModuleName\Api;
interface YourServiceInterface
{
/**
* Get custom data
*
* @param int $id
* @return string
*/
public function getCustomData($id);
/**
* Save custom data
*
* @param string $data
* @return bool
*/
public function saveCustomData($data);
}
Step 3: Implement the API Interface
Now, create a class that implements the interface. This class will contain the actual logic for your API. Create a file called Model/YourService.php
:
<?php
namespace Vendor\ModuleName\Model;
use Vendor\ModuleName\Api\YourServiceInterface;
class YourService implements YourServiceInterface
{
/**
* {@inheritdoc}
*/
public function getCustomData($id)
{
return "Custom data for ID: " . $id;
}
/**
* {@inheritdoc}
*/
public function saveCustomData($data)
{
// Logic to save data
return true;
}
}
Step 4: Configure the API in webapi.xml
To expose your API endpoints, you need to define them in the webapi.xml
file. Create this file in the etc
directory of your module:
<?xml version="1.0"?>
<routes xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Webapi:etc/webapi.xsd">
<route url="/V1/custom-data/:id" method="GET">
<service class="Vendor\ModuleName\Api\YourServiceInterface" method="getCustomData"/>
<resources>
<resource ref="anonymous"/>
</resources>
</route>
<route url="/V1/custom-data" method="POST">
<service class="Vendor\ModuleName\Api\YourServiceInterface" method="saveCustomData"/>
<resources>
<resource ref="anonymous"/>
</resources>
</route>
</routes>
This configuration defines two endpoints: one for retrieving data and one for saving data.
Step 5: Test Your API
Once everything is set up, you can test your API using tools like Postman or cURL. For example, to test the GET endpoint, you can use:
curl -X GET "http://your-magento-site.com/rest/V1/custom-data/1"
And to test the POST endpoint:
curl -X POST "http://your-magento-site.com/rest/V1/custom-data" -H "Content-Type: application/json" -d '{"data": "example"}'
Step 6: Secure Your API
By default, the above configuration allows anonymous access. For production, you’ll want to secure your API by restricting access to authenticated users. Update the <resource>
tag in webapi.xml
to use a specific resource:
<resource ref="Magento_Backend::admin"/>
This ensures that only users with admin privileges can access the API.
Step 7: Extend Your API
Now that you’ve built a basic API, you can extend it to include more complex functionality. For example, you can add endpoints for updating or deleting data, or integrate with other Magento services like product management or order processing.
Final Thoughts
Creating custom REST APIs in Magento 2 is a powerful way to extend your store’s functionality and integrate with external systems. By following the steps above, you can build APIs tailored to your specific needs. Whether you’re integrating with a third-party system or building a custom frontend, REST APIs are an essential tool in your Magento 2 development toolkit.
If you’re looking for more advanced features or need help optimizing your Magento store, check out the extensions and hosting solutions available at magefine.com. Happy coding!