10 Magento development tips | Episode 3 : Custom caching
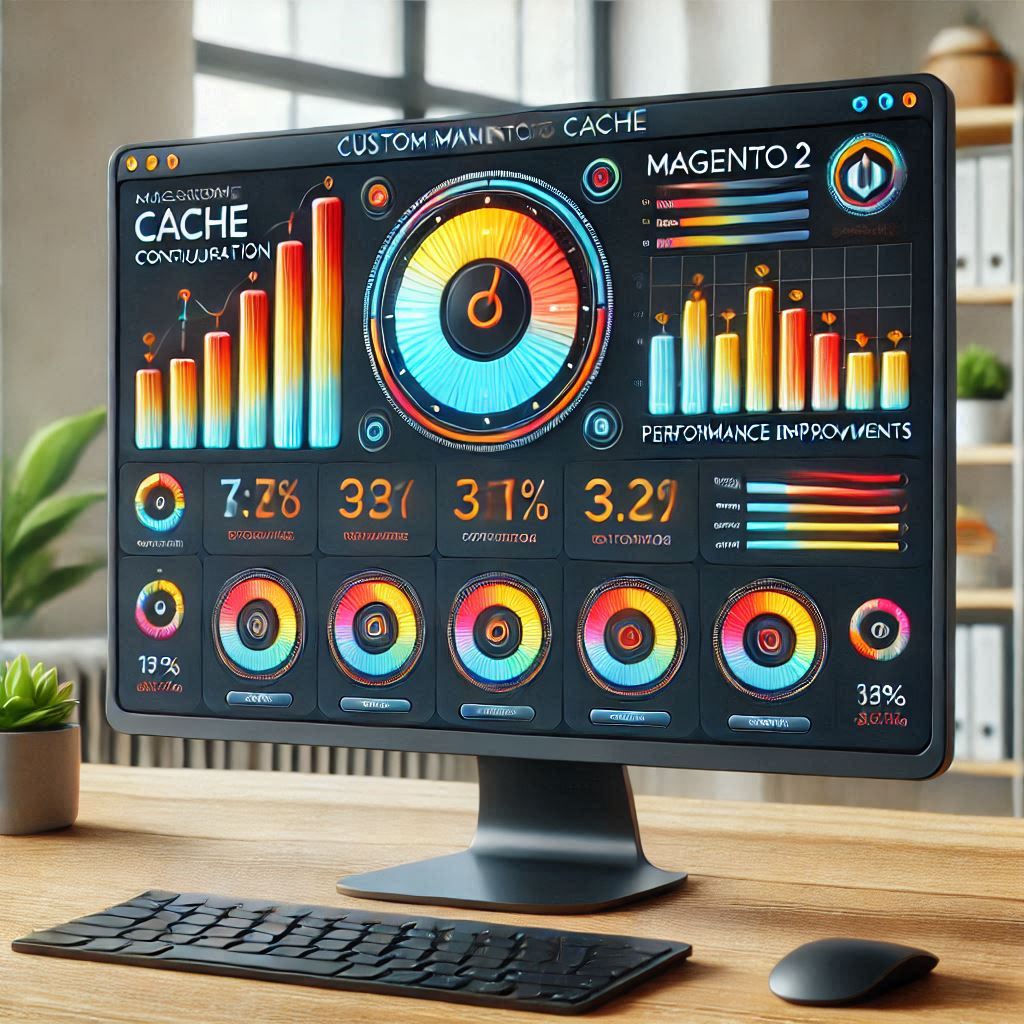
Caching is one of the most powerful tools in a Magento 2 developer's arsenal for enhancing e-commerce site performance. Although Magento 2 provides several default cache types, there are times when application-specific data requires custom handling. Here's how you can effectively implement a custom cache in Magento 2 to store data optimally.
Why Use Custom Cache?
Before diving into implementation, understand why you might need a custom cache:
- Unique Data: If your modules deal with data that doesn't fit into Magento's existing cache types.
- Performance: For data that's frequently accessed but costly to generate.
- Control: To have fine-grained control over when and how data is cached or invalidated.
Step 1: Planning
Identify Data to Cache:
Begin by analyzing which data is most frequently used and consumes significant resources when generated.
Define Cache Lifetime:
Wisely choose the lifetime of cached data. Too short, and you won't reap performance benefits; too long, and you risk showing outdated information.
Step 2: Creating the Cache Type
Magento 2 allows you to create custom cache types through modules. Here's how:
Create a Module:
Set up a Magento 2 module if you don't have one already, located in app/code/YourNamespace/YourModule
.
Define the Cache in cache.xml
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Cache/etc/cache.xsd">
<type name="your_unique_cache" translate="label,description" instance="YourNamespace\YourModule\Model\Cache\Type">
<label>Your Custom Cache</label>
<description>Description of your custom cache.</description>
</type>
</config>
Implement the Cache Class:
<?php
namespace YourNamespace\YourModule\Model\Cache;
use Magento\Framework\App\Cache\Type\FrontendPool;
class Type extends \Magento\Framework\Cache\Frontend\Decorator\TagScope
{
const TYPE_IDENTIFIER = 'your_unique_cache';
const CACHE_TAG = 'YOUR_UNIQUE_CACHE';
public function __construct(FrontendPool $cacheFrontendPool)
{
parent::__construct($cacheFrontendPool->get(self::TYPE_IDENTIFIER), self::CACHE_TAG);
}
}
Step 3: Using the Cache
Dependency Injection:
In classes where you want to use the cache, inject Magento\Framework\App\CacheInterface
through the constructor.
Saving and Loading Data:
<?php
use Magento\Framework\App\CacheInterface;
public function __construct(CacheInterface $cache)
{
$this->cache = $cache;
}
public function getCustomData($identifier)
{
$data = $this->cache->load($identifier);
if ($data === false) {
$data = $this->generateExpensiveData($identifier);
$this->cache->save(serialize($data), $identifier, [Type::CACHE_TAG], 86400); // Cache for 24 hours
}
return unserialize($data);
}
private function generateExpensiveData($identifier)
{
// Logic to generate expensive data
return ['some' => 'data'];
}
Step 4: Cache Management
Cache Invalidation:
public function invalidateCache()
{
$this->cache->clean([Type::CACHE_TAG]);
}
Define when cached data should be invalidated. This could be based on system events or user actions:
Cache Management UI:
Your custom cache will appear in the admin panel under Cache Management, allowing admins to clear it manually.
Step 5: Best Practices
- Use Cache Tags: Tags allow you to clean specific portions of the cache without clearing everything, which is crucial for dynamic data.
- Don't Overuse Caching: Ensure caching is used judiciously. Over-caching can increase memory usage without significant benefits.
- Testing and Monitoring: Test your caching implementation to ensure it works as intended and monitor performance before and after implementation.
- Documentation: Clearly document how your cache is used to help future developers understand and maintain the system.
Conclusion
Implementing a custom cache in Magento 2 can dramatically improve your site's performance, especially for application-specific data. By following these steps, you can ensure your cache is efficient, well-managed, and significantly contributes to the user experience. Remember, optimization is an ongoing process, so take time to evaluate and refine your caching approach over time.