What is a UI Component in Magento 2?
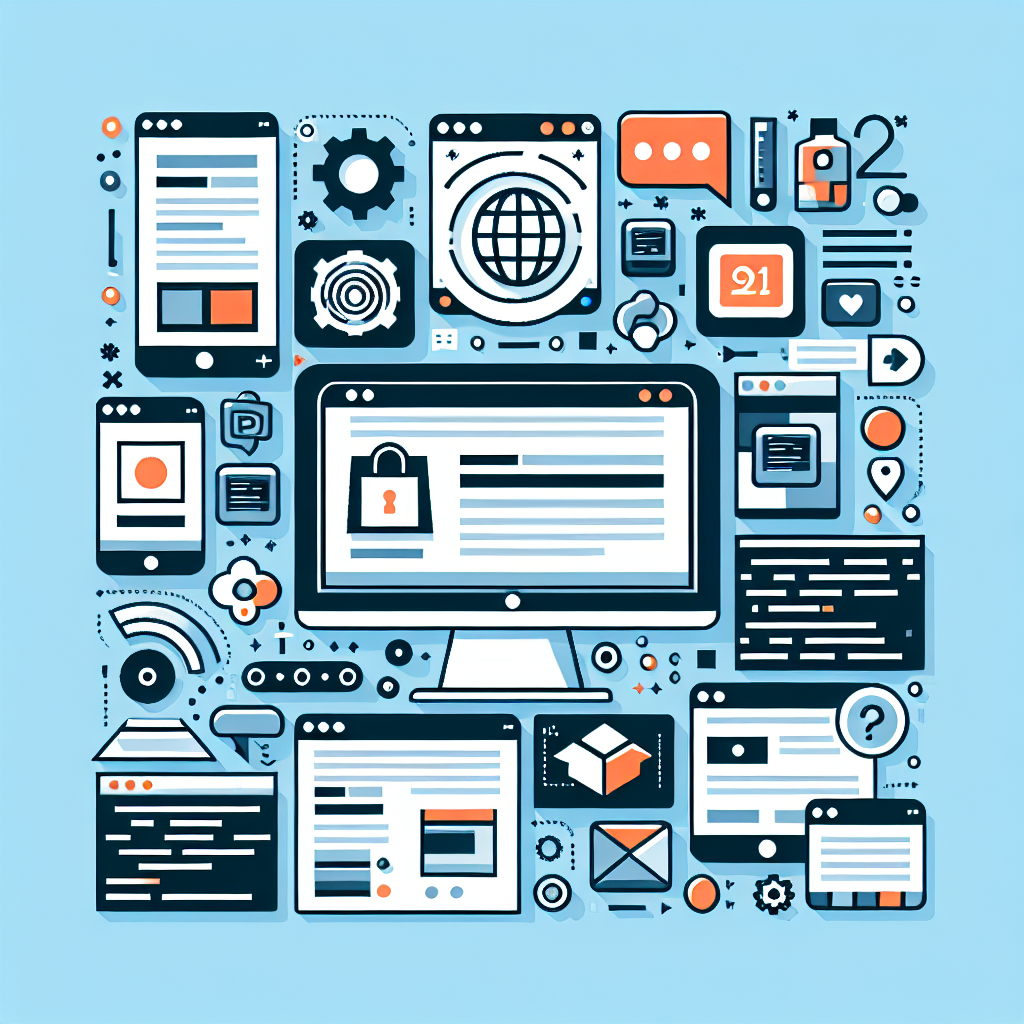
What is a UI Component in Magento 2?
If you're diving into Magento 2 development, you've probably heard the term "UI Component" thrown around. But what exactly is it? Simply put, a UI Component is a reusable piece of code that helps you build and manage the admin and frontend interfaces in Magento 2. Think of it like Lego blocks—each component has a specific function, and you can combine them to create complex pages without reinventing the wheel every time.
Magento 2 uses UI Components extensively in its admin panel—product grids, forms, and even checkout steps are built using them. They make development faster, more organized, and easier to maintain. Plus, they follow Magento’s best practices, so your customizations stay clean and upgrade-friendly.
Why Should You Care About UI Components?
If you're customizing Magento 2, UI Components are your best friends. Here’s why:
- Reusable: Write once, use everywhere.
- Modular: Easy to extend or override.
- Declarative: Define behavior in XML instead of hardcoding PHP.
- Performance-Optimized: Built with Knockout.js for smooth frontend interactions.
Types of UI Components in Magento 2
Magento 2 comes with several built-in UI Components. The most common ones are:
- Listing (Grid): Used for displaying data tables (like product grids).
- Form: Handles input fields, validation, and submissions.
- Buttons: Action buttons like "Save" or "Delete."
- Modal: Pop-up dialogs for confirmations or additional forms.
How to Create a Simple UI Component
Let’s walk through creating a basic UI Component—a custom admin grid.
Step 1: Define the Component in XML
First, create a file at: app/code/Vendor/Module/view/adminhtml/ui_component/custom_grid.xml
<listing xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Ui:etc/ui_configuration.xsd">
<argument name="data" xsi:type="array">
<item name="js_config" xsi:type="array">
<item name="provider" xsi:type="string">custom_grid.custom_grid_data_source</item>
</item>
</argument>
<dataSource name="custom_grid_data_source">
<argument name="dataProvider" xsi:type="configurableObject">
<argument name="class" xsi:type="string">Vendor\Module\Model\DataProvider</argument>
</argument>
</dataSource>
<columns name="custom_grid_columns">
<column name="id">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="filter" xsi:type="string">text</item>
<item name="label" xsi:type="string">ID</item>
</item>
</argument>
</column>
</columns>
</listing>
Step 2: Create a Data Provider
Next, define a data provider at: app/code/Vendor/Module/Model/DataProvider.php
<?php
namespace Vendor\Module\Model;
use Magento\Framework\View\Element\UiComponent\DataProvider\DataProvider;
class DataProvider extends \Magento\Framework\View\Element\UiComponent\DataProvider\DataProvider
{
public function getData()
{
return [
'items' => [
['id' => 1, 'name' => 'Sample Item 1'],
['id' => 2, 'name' => 'Sample Item 2'],
]
];
}
}
Step 3: Display the Grid in the Admin
Finally, create a layout file to render the grid:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<uiComponent name="custom_grid"/>
</referenceContainer>
</body>
</page>
Now, when you navigate to your custom admin route, you’ll see a simple grid displaying the sample data!
Extending UI Components
One of the biggest advantages of UI Components is that you can extend existing ones. For example, if you want to add a custom column to the product grid:
<listing xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Ui:etc/ui_configuration.xsd">
<columns name="product_columns">
<column name="custom_field">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="filter" xsi:type="string">text</item>
<item name="label" xsi:type="string">Custom Field</item>
</item>
</argument>
</column>
</columns>
</listing>
Common Pitfalls & Best Practices
- Don’t Override Core Components Unnecessarily: Use plugins or preferences only when needed.
- Use XML for Configuration: Avoid hardcoding logic in PHP when possible.
- Test Performance: Complex UI Components can slow down the admin—optimize queries.
- Keep It Modular: Break down large components into smaller, reusable pieces.
Final Thoughts
UI Components are a powerful feature in Magento 2 that streamline admin and frontend development. Once you get the hang of them, you’ll be able to build complex interfaces quickly and efficiently. Start small—create a custom grid, tweak an existing form—and gradually explore more advanced use cases.
Need help optimizing your Magento 2 store? Check out Magefine for extensions and hosting solutions!