Understand the Dependency Injection System in Magento 2
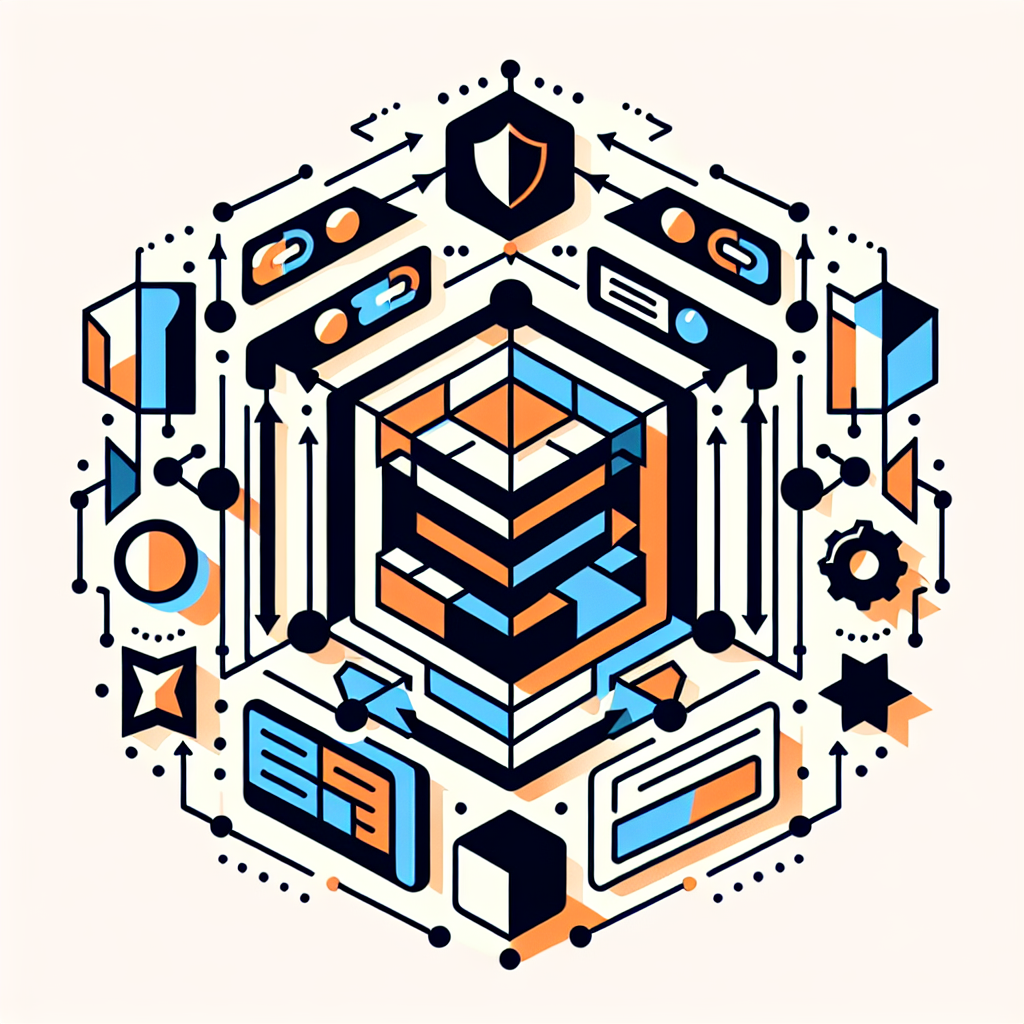
Understand the Dependency Injection System in Magento 2
If you're diving into Magento 2 development, one of the first concepts you'll encounter is Dependency Injection (DI). It’s a core part of how Magento 2 works, and understanding it is essential if you want to build custom modules or modify existing ones. Don’t worry if it sounds intimidating—by the end of this post, you’ll have a solid grasp of what DI is, why it’s important, and how to use it in your Magento 2 projects.
What is Dependency Injection?
Dependency Injection is a design pattern that allows you to inject dependencies (like services, models, or helpers) into a class rather than having the class create them itself. This makes your code more modular, testable, and easier to maintain. In Magento 2, DI is used extensively to manage object creation and dependencies.
Think of it like this: instead of a class saying, "I need this specific tool to work," it says, "I need something that can do this job." The system then provides the appropriate tool (dependency) when the class is instantiated.
Why is Dependency Injection Important in Magento 2?
Magento 2 is built with modularity and scalability in mind. DI helps achieve this by decoupling classes and their dependencies. This means:
- Easier Testing: You can mock dependencies in unit tests.
- Better Maintainability: Changes in one part of the system don’t ripple through the entire codebase.
- Flexibility: You can swap out dependencies without modifying the class that uses them.
How Does Dependency Injection Work in Magento 2?
Magento 2 uses a combination of DI and a service container (also known as the Object Manager) to manage dependencies. Here’s a step-by-step breakdown of how it works:
- Define Dependencies: You specify the dependencies a class needs in its constructor.
- Configure DI: Magento’s DI configuration files (like
di.xml
) map dependencies to their implementations. - Instantiate Objects: The Object Manager injects the required dependencies when a class is instantiated.
Example: Using Dependency Injection in a Custom Module
Let’s say you’re building a custom module that needs to use Magento’s ProductRepository
to fetch product data. Here’s how you’d do it:
- Create a Class with Dependencies:
<?php
namespace Magefine\CustomModule\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
class ProductData
{
protected $productRepository;
public function __construct(ProductRepositoryInterface $productRepository)
{
$this->productRepository = $productRepository;
}
public function getProductById($productId)
{
return $this->productRepository->getById($productId);
}
}
In this example, the ProductData
class depends on ProductRepositoryInterface
. Magento will automatically inject the correct implementation when the class is instantiated.
- Configure DI in
di.xml
:
If you need to customize how dependencies are injected, you can do so in your module’s di.xml
file. For example:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magefine\CustomModule\Model\ProductData">
<arguments>
<argument name="productRepository" xsi:type="object">Magento\Catalog\Model\ProductRepository</argument>
</arguments>
</type>
</config>
This configuration tells Magento to use the ProductRepository
class when injecting the ProductRepositoryInterface
dependency.
Types of Dependency Injection in Magento 2
Magento 2 supports three types of dependency injection:
- Constructor Injection: Dependencies are passed to the class via its constructor (as shown in the example above).
- Setter Injection: Dependencies are set using setter methods. This is less common in Magento 2.
- Interface Injection: Dependencies are injected based on interfaces. This is the most flexible and widely used approach in Magento 2.
Best Practices for Using Dependency Injection
Here are some tips to make the most of DI in Magento 2:
- Use Constructor Injection: It’s the most straightforward and recommended approach.
- Avoid Using the Object Manager Directly: While Magento’s Object Manager is powerful, it’s best to let the DI system handle object creation.
- Keep Dependencies Minimal: Only inject what you need to keep your classes lightweight and focused.
- Leverage Interfaces: Use interfaces to define dependencies, making your code more flexible and easier to test.
Common Pitfalls to Avoid
While DI is powerful, it’s easy to misuse if you’re not careful. Here are some common mistakes to watch out for:
- Overloading Constructors: Injecting too many dependencies can make your class hard to manage. Consider breaking it into smaller classes if this happens.
- Circular Dependencies: When two classes depend on each other, it can cause issues. Magento’s DI system will throw an error in such cases.
- Ignoring DI Configuration: Always define your dependencies in
di.xml
to avoid unexpected behavior.
Real-World Example: Extending a Core Class
Let’s say you want to extend Magento’s ProductRepository
to add custom logic. Here’s how you’d do it using DI:
- Create a Custom Repository Class:
<?php
namespace Magefine\CustomModule\Model;
use Magento\Catalog\Model\ProductRepository as CoreProductRepository;
class ProductRepository extends CoreProductRepository
{
public function getById($productId, $editMode = false, $storeId = null, $forceReload = false)
{
$product = parent::getById($productId, $editMode, $storeId, $forceReload);
// Add your custom logic here
return $product;
}
}
- Configure DI to Use Your Custom Class:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<preference for="Magento\Catalog\Api\ProductRepositoryInterface" type="Magefine\CustomModule\Model\ProductRepository" />
</config>
This configuration tells Magento to use your custom ProductRepository
class whenever ProductRepositoryInterface
is requested.
Conclusion
Dependency Injection is a fundamental concept in Magento 2 that makes your code more modular, testable, and maintainable. By understanding how it works and following best practices, you can build robust and scalable Magento 2 modules. Whether you’re extending core functionality or creating custom features, DI will be your best friend.
If you’re looking for more Magento 2 tips or need help with hosting and extensions, check out Magefine.com. We’ve got everything you need to take your Magento store to the next level!