How to Implement a Custom Customer Attribute in Magento 2
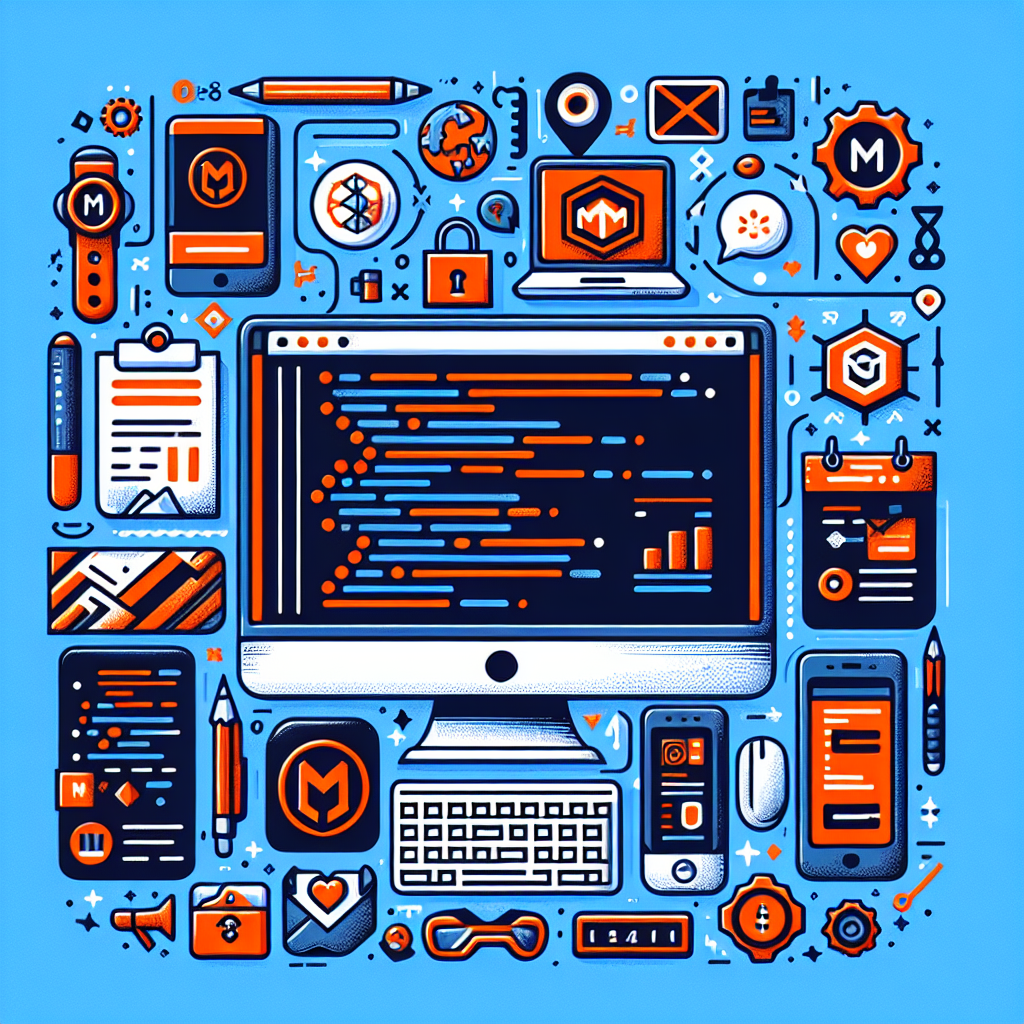
How to Implement a Custom Customer Attribute in Magento 2
Hey there! So, you’ve decided to dive into the world of Magento 2 customization, and you’re looking to add a custom customer attribute. Whether it’s to store additional customer information or to enhance your store’s functionality, creating custom attributes is a powerful way to tailor Magento 2 to your specific needs. Don’t worry if you’re new to this—I’ll walk you through the process step by step, and by the end, you’ll have a solid understanding of how to implement a custom customer attribute in Magento 2.
What is a Custom Customer Attribute?
Before we jump into the code, let’s quickly define what a custom customer attribute is. In Magento 2, customer attributes are pieces of information that you collect and store about your customers. By default, Magento comes with a set of predefined attributes like first name, last name, email, and so on. However, there might be cases where you need to store additional information, such as a customer’s favorite color, preferred payment method, or even their social media handle. That’s where custom customer attributes come into play.
Why Would You Need a Custom Customer Attribute?
Custom customer attributes can be incredibly useful for a variety of reasons:
- Personalization: You can use custom attributes to personalize the shopping experience for your customers. For example, if you know a customer’s favorite color, you can recommend products that match their preference.
- Marketing: Custom attributes can help you segment your customer base for targeted marketing campaigns. For instance, you could create a custom attribute to track which customers are interested in a particular product category.
- Data Collection: If you need to collect specific information from your customers that isn’t covered by the default attributes, custom attributes are the way to go.
Step 1: Create a Module
In Magento 2, all customizations are done through modules. So, the first step is to create a new module. Don’t worry—it’s not as complicated as it sounds. Here’s how you can do it:
- Navigate to your Magento 2 installation directory.
- Create a new folder under
app/code
. For this example, let’s call itMagefine/CustomAttribute
. - Inside the
Magefine/CustomAttribute
folder, create aregistration.php
file with the following content:
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Magefine_CustomAttribute',
__DIR__
);
This file registers your module with Magento.
- Next, create a
etc/module.xml
file in the same folder with the following content:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Magefine_CustomAttribute" setup_version="1.0.0"/>
</config>
This file defines the basic information about your module, such as its name and version.
Step 2: Create the InstallData Script
Now that your module is set up, it’s time to create the custom customer attribute. In Magento 2, this is done using an InstallData
script. Here’s how you can create one:
- Create a new folder called
Setup
inside your module’s directory (app/code/Magefine/CustomAttribute/Setup
). - Inside the
Setup
folder, create a file namedInstallData.php
with the following content:
<?php
namespace Magefine\CustomAttribute\Setup;
use Magento\Customer\Model\Customer;
use Magento\Customer\Setup\CustomerSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $customerSetupFactory;
public function __construct(CustomerSetupFactory $customerSetupFactory)
{
$this->customerSetupFactory = $customerSetupFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerSetup->addAttribute(
Customer::ENTITY,
'favorite_color',
[
'type' => 'varchar',
'label' => 'Favorite Color',
'input' => 'text',
'required' => false,
'visible' => true,
'user_defined' => true,
'position' => 100,
'system' => 0,
]
);
$attribute = $customerSetup->getEavConfig()->getAttribute(Customer::ENTITY, 'favorite_color');
$attribute->setData('used_in_forms', ['adminhtml_customer', 'customer_account_create', 'customer_account_edit']);
$attribute->save();
$setup->endSetup();
}
}
Let’s break down what’s happening here:
- We’re using the
CustomerSetupFactory
to create a new customer attribute. - The
addAttribute
method is used to define the attribute. In this case, we’re creating afavorite_color
attribute of typevarchar
(a string). - We’re setting various options for the attribute, such as whether it’s required, visible, and user-defined.
- Finally, we’re specifying which forms the attribute should appear in, such as the customer registration form and the admin customer edit form.
Step 3: Run the Setup Upgrade
Now that your InstallData
script is ready, you need to run the setup upgrade to apply the changes. Here’s how you can do it:
- Open your terminal and navigate to your Magento 2 root directory.
- Run the following command:
php bin/magento setup:upgrade
This command will execute the InstallData
script and create the custom customer attribute in your Magento 2 database.
Step 4: Verify the Custom Attribute
Once the setup upgrade is complete, you should be able to see your new custom attribute in the Magento admin panel. Here’s how you can verify it:
- Log in to your Magento admin panel.
- Navigate to Customers > All Customers.
- Edit any customer or create a new one.
- You should see the Favorite Color field in the customer form.
Congratulations! You’ve successfully created a custom customer attribute in Magento 2.
Step 5: Using the Custom Attribute in Your Code
Now that your custom attribute is in place, you might want to use it in your custom code. For example, you might want to display the customer’s favorite color on their account page. Here’s how you can retrieve the value of the custom attribute:
<?php
namespace Magefine\CustomAttribute\Block;
use Magento\Framework\View\Element\Template;
use Magento\Customer\Model\Session;
class FavoriteColor extends Template
{
protected $customerSession;
public function __construct(
Template\Context $context,
Session $customerSession,
array $data = []
) {
$this->customerSession = $customerSession;
parent::__construct($context, $data);
}
public function getFavoriteColor()
{
$customer = $this->customerSession->getCustomer();
return $customer->getData('favorite_color');
}
}
In this example, we’re creating a block that retrieves the customer’s favorite color from the session. You can then use this block in your template to display the value:
<?php if ($block->getFavoriteColor()): ?>
<p>Your favorite color is: <?= $block->getFavoriteColor() ?></p>
<?php endif; ?>
Conclusion
Adding a custom customer attribute in Magento 2 is a straightforward process once you understand the basics. By following the steps outlined in this guide, you can easily create and use custom attributes to enhance your store’s functionality. Whether you’re looking to personalize the shopping experience, segment your customer base, or collect additional information, custom attributes are a powerful tool in your Magento 2 arsenal.
If you have any questions or run into any issues, feel free to reach out. Happy coding!