How to Implement a Custom Product Attribute in Magento 2
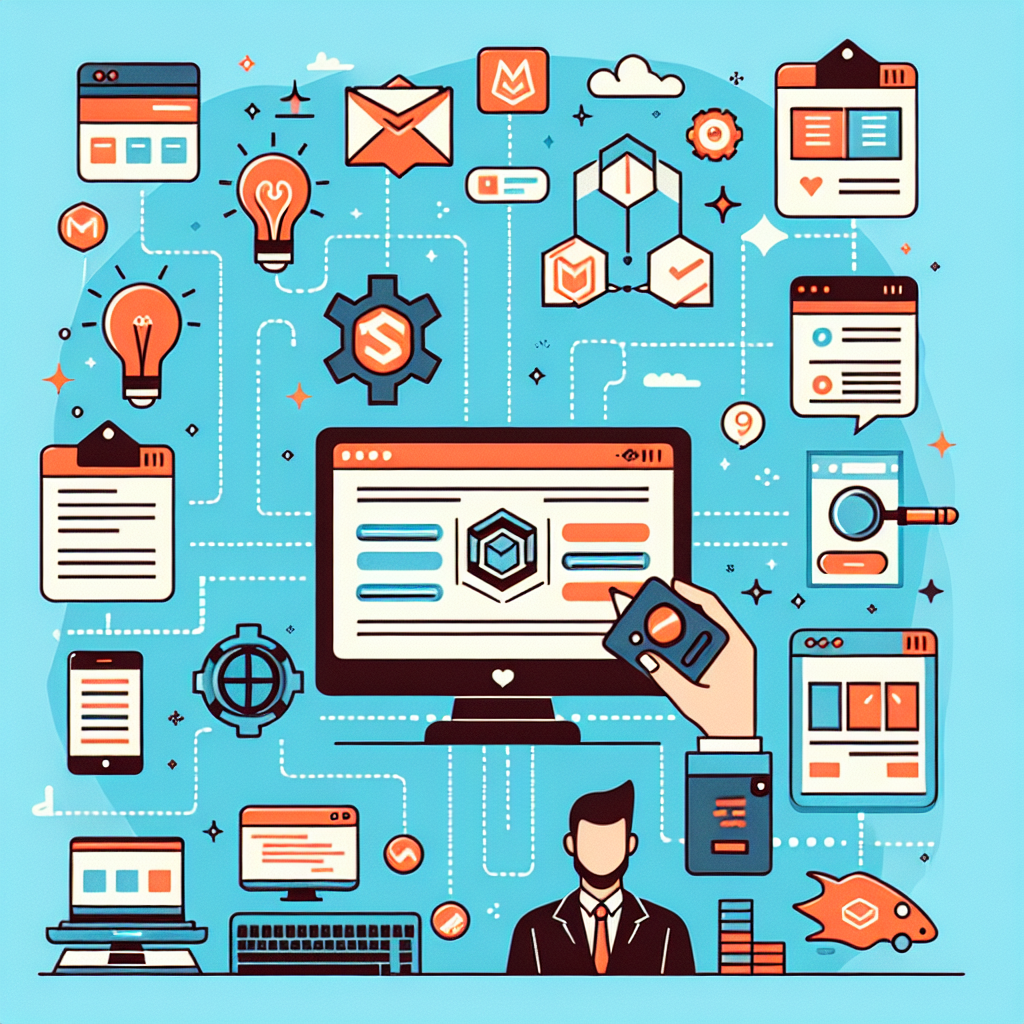
How to Implement a Custom Product Attribute in Magento 2
Hey there! So, you’re diving into Magento 2 and want to add a custom product attribute? Great choice! Custom product attributes are super useful for adding extra details to your products, like material type, warranty info, or even a custom SKU format. In this guide, I’ll walk you through the process step by step, so even if you’re new to Magento, you’ll be able to follow along easily.
Why Add a Custom Product Attribute?
Before we jump into the code, let’s talk about why you might want to add a custom product attribute. Custom attributes allow you to store additional information about your products that aren’t covered by the default Magento attributes. This can be super helpful for:
- Adding unique product details (e.g., "Made in Italy").
- Enhancing search and filtering options.
- Customizing product displays on the frontend.
Now that we’ve covered the "why," let’s get into the "how."
Step 1: Create a Module
First things first, we need to create a custom module. Don’t worry, it’s easier than it sounds! Here’s how you do it:
- Navigate to your Magento 2 root directory.
- Create the following folder structure:
app/code/YourVendor/YourModule
. - Inside the
YourModule
folder, create two files:registration.php
andetc/module.xml
.
Here’s what the registration.php
file should look like:
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'YourVendor_YourModule',
__DIR__
);
And here’s the module.xml
file:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="YourVendor_YourModule" setup_version="1.0.0"/>
</config>
Once you’ve created these files, run the following command to enable your module:
php bin/magento setup:upgrade
Step 2: Create the InstallData Script
Next, we’ll create an InstallData
script to add our custom attribute. This script will run when the module is installed. Here’s how to do it:
- Create a new folder:
app/code/YourVendor/YourModule/Setup
. - Inside the
Setup
folder, create a file namedInstallData.php
.
Here’s the code for InstallData.php
:
<?php
namespace YourVendor\YourModule\Setup;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
public function __construct(EavSetupFactory $eavSetupFactory)
{
$this->eavSetupFactory = $eavSetupFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->addAttribute(
\Magento\Catalog\Model\Product::ENTITY,
'custom_attribute_code',
[
'type' => 'varchar',
'label' => 'Custom Attribute',
'input' => 'text',
'required' => false,
'sort_order' => 100,
'global' => \Magento\Eav\Model\Entity\Attribute\ScopedAttributeInterface::SCOPE_GLOBAL,
'visible' => true,
'user_defined' => true,
'default' => '',
'group' => 'General',
]
);
}
}
In this script, we’re adding a custom attribute called custom_attribute_code
with a text input field. You can customize the options as needed.
Step 3: Run the Setup Upgrade Command
After creating the InstallData
script, run the following command to apply the changes:
php bin/magento setup:upgrade
This will install your module and add the custom attribute to your products.
Step 4: Verify the Attribute in the Admin Panel
Now that the attribute is added, let’s make sure it’s working correctly. Here’s how to check:
- Log in to your Magento 2 admin panel.
- Go to Stores > Attributes > Product.
- Look for your custom attribute (
custom_attribute_code
) in the list.
If you see it there, congratulations! You’ve successfully added a custom product attribute.
Step 5: Display the Attribute on the Frontend
Now that the attribute is added, you might want to display it on the product page. Here’s how to do that:
- Create a new file:
app/code/YourVendor/YourModule/view/frontend/templates/product/view/custom_attribute.phtml
. - Add the following code to the file:
<?php
$product = $block->getProduct();
$customAttribute = $product->getData('custom_attribute_code');
if ($customAttribute) {
echo '<div class="custom-attribute">' . $customAttribute . '</div>';
}
?>
Next, update your product view layout file to include this template. Create or edit the file at app/code/YourVendor/YourModule/view/frontend/layout/catalog_product_view.xml
:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="product.info.main">
<block class="Magento\Framework\View\Element\Template" name="custom.attribute" template="YourVendor_YourModule::product/view/custom_attribute.phtml"/>
</referenceContainer>
</body>
</page>
This will display your custom attribute on the product page under the "Product Info" section.
Step 6: Use the Attribute in Filters and Search
If you want your custom attribute to be searchable or filterable, you’ll need to update its properties. Go back to your InstallData.php
file and modify the attribute options like this:
'searchable' => true,
'filterable' => true,
'visible_in_advanced_search' => true,
Then, run the setup upgrade command again:
php bin/magento setup:upgrade
Now, your custom attribute will be available for search and filtering in the layered navigation.
Step 7: Test Everything
Finally, test your custom attribute to make sure everything works as expected. Add a value to the attribute for a product, then check the frontend to see if it displays correctly. Also, try searching and filtering by the attribute to ensure it’s working.
Wrapping Up
And that’s it! You’ve successfully added a custom product attribute in Magento 2. Whether you’re adding a simple text field or something more complex, this process will help you extend your product data to meet your store’s needs. If you have any questions or run into issues, feel free to drop a comment below. Happy coding!
```