How to Implement a Custom Logging System in Magento 2
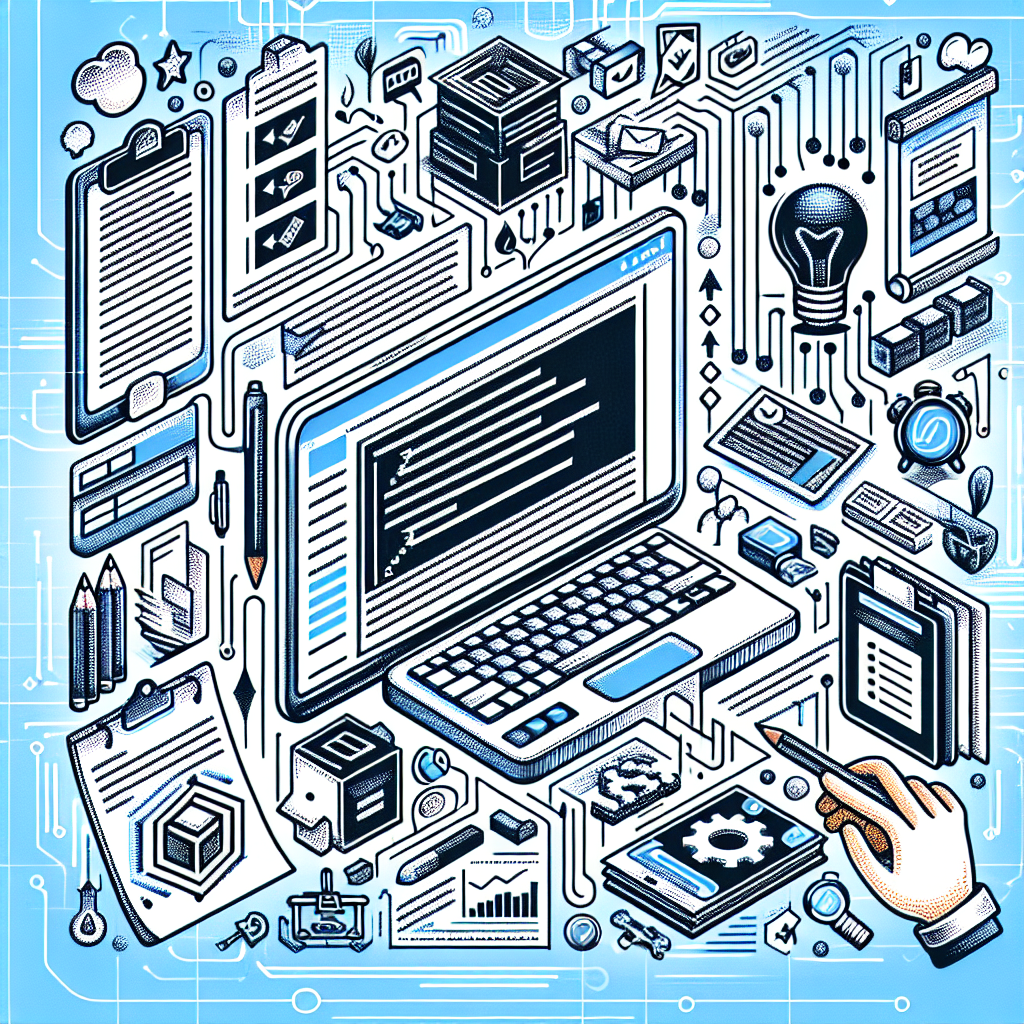
How to Implement a Custom Logging System in Magento 2
Logging is an essential part of any Magento 2 store. It helps you keep track of errors, debug issues, and monitor the performance of your store. While Magento 2 comes with its own logging system, there are times when you might need a custom logging system to meet specific requirements. In this blog post, we'll walk you through the process of implementing a custom logging system in Magento 2.
Why Do You Need a Custom Logging System?
Magento 2's default logging system is robust and covers most of the common use cases. However, there are scenarios where you might need more control over your logs. For example, you might want to:
- Log specific events that are not covered by the default system.
- Store logs in a different format or location.
- Integrate with third-party logging services like Loggly or Splunk.
- Filter logs based on specific criteria.
In such cases, implementing a custom logging system can be beneficial.
Step 1: Create a Custom Logger Class
The first step in creating a custom logging system is to create a custom logger class. This class will handle the actual logging logic. Here's an example of how you can create a custom logger class:
namespace Magefine\CustomLogger\Logger;
class Logger extends \Monolog\Logger
{
public function __construct($name, array $handlers = [], array $processors = [])
{
parent::__construct($name, $handlers, $processors);
}
public function customLog($message, array $context = [])
{
$this->info($message, $context);
}
}
In this example, we're extending the `Monolog\Logger` class, which is the default logger used by Magento 2. We've added a custom method `customLog` that logs messages with the `info` level.
Step 2: Register the Custom Logger in di.xml
Next, you need to register your custom logger in the `di.xml` file. This will allow Magento 2 to recognize your custom logger and use it when needed. Here's how you can do it:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magefine\CustomLogger\Logger\Logger">
<arguments>
<argument name="name" xsi:type="string">custom_logger</argument>
<argument name="handlers" xsi:type="array">
<item name="system" xsi:type="object">Magento\Framework\Logger\Handler\System</item>
</argument>
</arguments>
</type>
</config>
In this example, we're registering our custom logger with the name `custom_logger` and specifying that it should use the `System` handler for logging.
Step 3: Use the Custom Logger in Your Code
Now that your custom logger is registered, you can use it in your code. Here's an example of how you can use the custom logger in a Magento 2 controller:
namespace Magefine\CustomLogger\Controller\Index;
use Magento\Framework\App\Action\Action;
use Magento\Framework\App\Action\Context;
use Magefine\CustomLogger\Logger\Logger;
class Index extends Action
{
protected $logger;
public function __construct(Context $context, Logger $logger)
{
$this->logger = $logger;
parent::__construct($context);
}
public function execute()
{
$this->logger->customLog('This is a custom log message.');
return $this->resultFactory->create(\Magento\Framework\Controller\ResultFactory::TYPE_PAGE);
}
}
In this example, we're injecting our custom logger into the controller and using it to log a custom message.
Step 4: Customize the Logging Handler
If you want to customize where and how your logs are stored, you can create a custom logging handler. Here's an example of how you can create a custom handler that logs messages to a custom file:
namespace Magefine\CustomLogger\Logger\Handler;
use Monolog\Logger;
use Magento\Framework\Logger\Handler\Base;
class CustomFileHandler extends Base
{
protected $fileName = '/var/log/custom.log';
protected $loggerType = Logger::INFO;
}
In this example, we're creating a custom handler that logs messages to a file named `custom.log` in the `var/log` directory. You can then register this handler in your `di.xml` file:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magefine\CustomLogger\Logger\Logger">
<arguments>
<argument name="name" xsi:type="string">custom_logger</argument>
<argument name="handlers" xsi:type="array">
<item name="custom_file" xsi:type="object">Magefine\CustomLogger\Logger\Handler\CustomFileHandler</item>
</argument>
</arguments>
</type>
</config>
Now, your custom logger will use the custom file handler to log messages.
Step 5: Test Your Custom Logging System
Once you've implemented your custom logging system, it's important to test it to ensure that it's working as expected. You can do this by triggering the logging logic in your code and checking the log files to see if the messages are being logged correctly.
For example, if you've implemented the custom logger in a controller, you can visit the corresponding URL in your browser to trigger the logging logic. Then, check the log file specified in your custom handler to see if the message has been logged.
Conclusion
Implementing a custom logging system in Magento 2 can give you more control over how and where your logs are stored. By following the steps outlined in this blog post, you can create a custom logger, register it in Magento 2, and use it in your code. Whether you need to log specific events, store logs in a different format, or integrate with third-party logging services, a custom logging system can help you achieve your goals.
If you're looking for more advanced logging solutions or need help with Magento 2 hosting, be sure to check out magefine.com. We offer a wide range of Magento 2 extensions and hosting services to help you get the most out of your Magento store.