How to Implement a Custom Widget in Magento 2
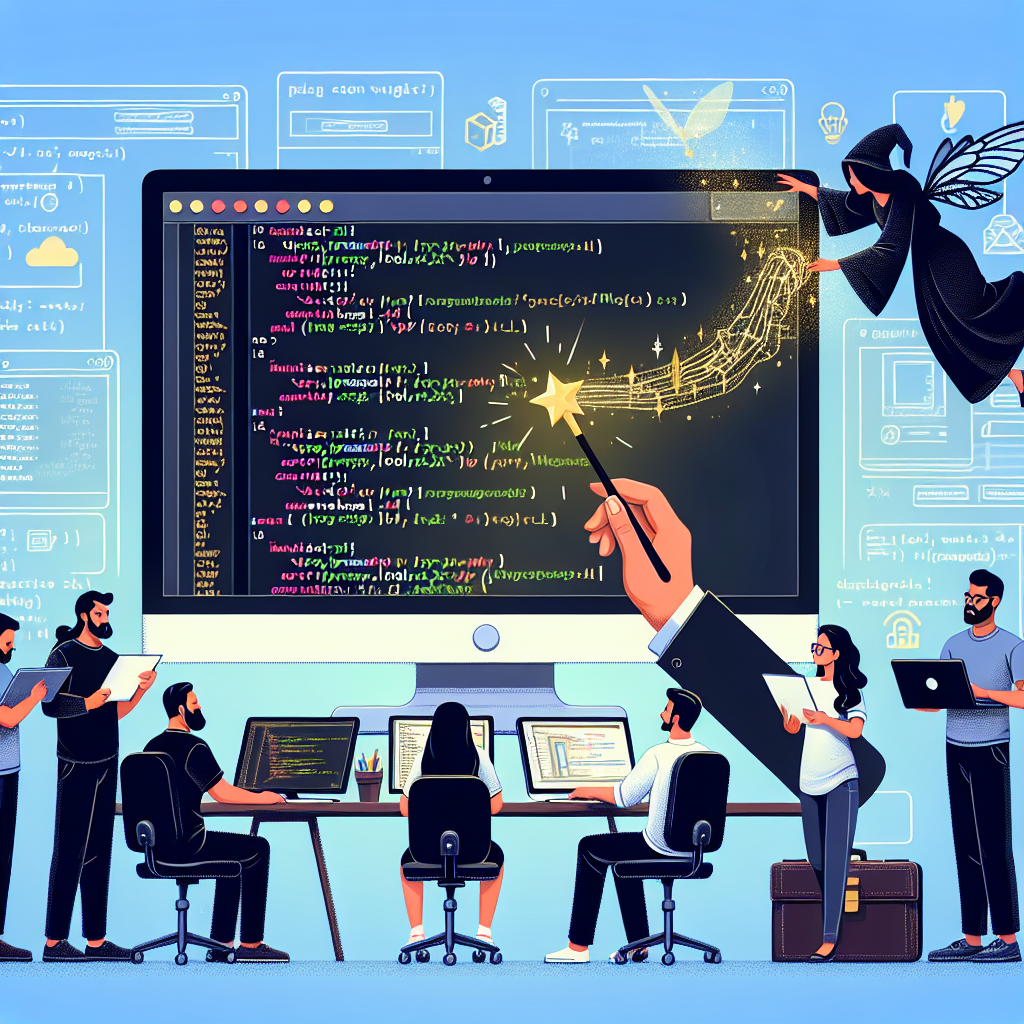
How to Implement a Custom Widget in Magento 2
If you're diving into Magento 2 development, you'll quickly realize that widgets are one of the most powerful tools at your disposal. They allow you to create reusable blocks of content that can be easily managed from the Magento admin panel. Whether you're looking to display a custom banner, a product slider, or any other dynamic content, widgets can save you a ton of time and effort.
In this post, we'll walk through the process of creating a custom widget in Magento 2. By the end, you'll have a solid understanding of how to implement your own widgets and integrate them into your store.
What is a Widget in Magento 2?
Before we jump into the code, let's clarify what a widget is in the context of Magento 2. A widget is essentially a reusable block of content that can be inserted into various parts of your store, such as the homepage, category pages, or even individual product pages. Widgets are managed through the Magento admin panel, making it easy for non-technical users to update content without touching the code.
Step 1: Create a Module
First things first, we need to create a custom module for our widget. If you're not familiar with creating modules in Magento 2, don't worry—it's straightforward. Here's how you can do it:
- Create the module directory structure:
- Create the
registration.php
file: - Create the
module.xml
file in theetc
directory:
app/code/Magefine/CustomWidget
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Magefine_CustomWidget',
__DIR__
);
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Magefine_CustomWidget" setup_version="1.0.0"/>
</config>
With these files in place, your module is ready to go. Run the following command to enable it:
php bin/magento setup:upgrade
Step 2: Define the Widget
Now that our module is set up, we need to define the widget itself. This involves creating a widget XML file that Magento will use to recognize and display our widget in the admin panel.
- Create the
widget.xml
file in theetc
directory:
<?xml version="1.0"?>
<widgets xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Widget:etc/widget.xsd">
<widget id="magefine_customwidget" class="Magefine\CustomWidget\Block\Widget\CustomWidget">
<label>Custom Widget</label>
<description>This is a custom widget created by Magefine.</description>
<parameters>
<parameter name="title" xsi:type="text" visible="true" required="true">
<label>Title</label>
</parameter>
<parameter name="content" xsi:type="textarea" visible="true" required="true">
<label>Content</label>
</parameter>
</parameters>
</widget>
</widgets>
In this XML file, we've defined a widget with two parameters: title
and content
. These parameters will be editable from the Magento admin panel when you add the widget to a page.
Step 3: Create the Widget Block
Next, we need to create a block class that will handle the rendering of our widget. This block will use the parameters defined in the widget.xml
file to generate the widget's output.
- Create the block class in
app/code/Magefine/CustomWidget/Block/Widget/CustomWidget.php
:
<?php
namespace Magefine\CustomWidget\Block\Widget;
use Magento\Framework\View\Element\Template;
use Magento\Widget\Block\BlockInterface;
class CustomWidget extends Template implements BlockInterface
{
protected $_template = "widget/custom_widget.phtml";
public function getTitle()
{
return $this->getData('title');
}
public function getContent()
{
return $this->getData('content');
}
}
This block class extends Magento's Template
class and implements the BlockInterface
. It also defines two methods, getTitle()
and getContent()
, which will be used to retrieve the widget's parameters.
Step 4: Create the Template File
Now that we have our block class, we need to create a template file that will define the HTML structure of our widget.
- Create the template file in
app/code/Magefine/CustomWidget/view/frontend/templates/widget/custom_widget.phtml
:
<div class="custom-widget">
<h2><?= $block->getTitle() ?></h2>
<p><?= $block->getContent() ?></p>
</div>
This template file simply outputs the title and content of the widget within a div
element. You can customize this HTML structure to fit your specific needs.
Step 5: Add the Widget to a Page
With everything in place, it's time to add our custom widget to a page in Magento. Here's how you can do it:
- Log in to the Magento admin panel.
- Navigate to Content > Pages.
- Edit or create a new page.
- In the content editor, click on the Insert Widget button.
- Select your custom widget from the list.
- Fill in the title and content fields.
- Save the page and preview it to see your custom widget in action.
Step 6: Customize and Extend
Now that you have a basic custom widget, you can start customizing and extending it to fit your specific needs. For example, you could add more parameters, such as an image upload field, or integrate with other Magento features like product listings or customer data.
Here's an example of how you could add an image parameter to your widget:
- Update the
widget.xml
file to include an image parameter: - Update the block class to include a method for retrieving the image URL:
- Update the template file to display the image:
<parameter name="image" xsi:type="image" visible="true" required="false">
<label>Image</label>
</parameter>
public function getImageUrl()
{
return $this->getData('image');
}
<div class="custom-widget">
<h2><?= $block->getTitle() ?></h2>
<?php if ($block->getImageUrl()): ?>
<img src="<?= $block->getImageUrl() ?>" alt="<?= $block->getTitle() ?>" />
<?php endif; ?>
<p><?= $block->getContent() ?></p>
</div>
With these changes, your widget will now support an optional image, which can be uploaded and managed through the Magento admin panel.
Conclusion
Creating a custom widget in Magento 2 is a powerful way to add dynamic, reusable content to your store. By following the steps outlined in this post, you can create a basic widget and extend it to meet your specific needs. Whether you're looking to display custom banners, product sliders, or any other type of content, widgets provide a flexible and user-friendly solution.
If you're looking for more advanced features or need help with your Magento 2 store, be sure to check out the extensions and hosting solutions available at magefine.com. Our team is dedicated to helping you get the most out of your Magento store.
Happy coding!