How to Create a Custom Product Type in Magento 2
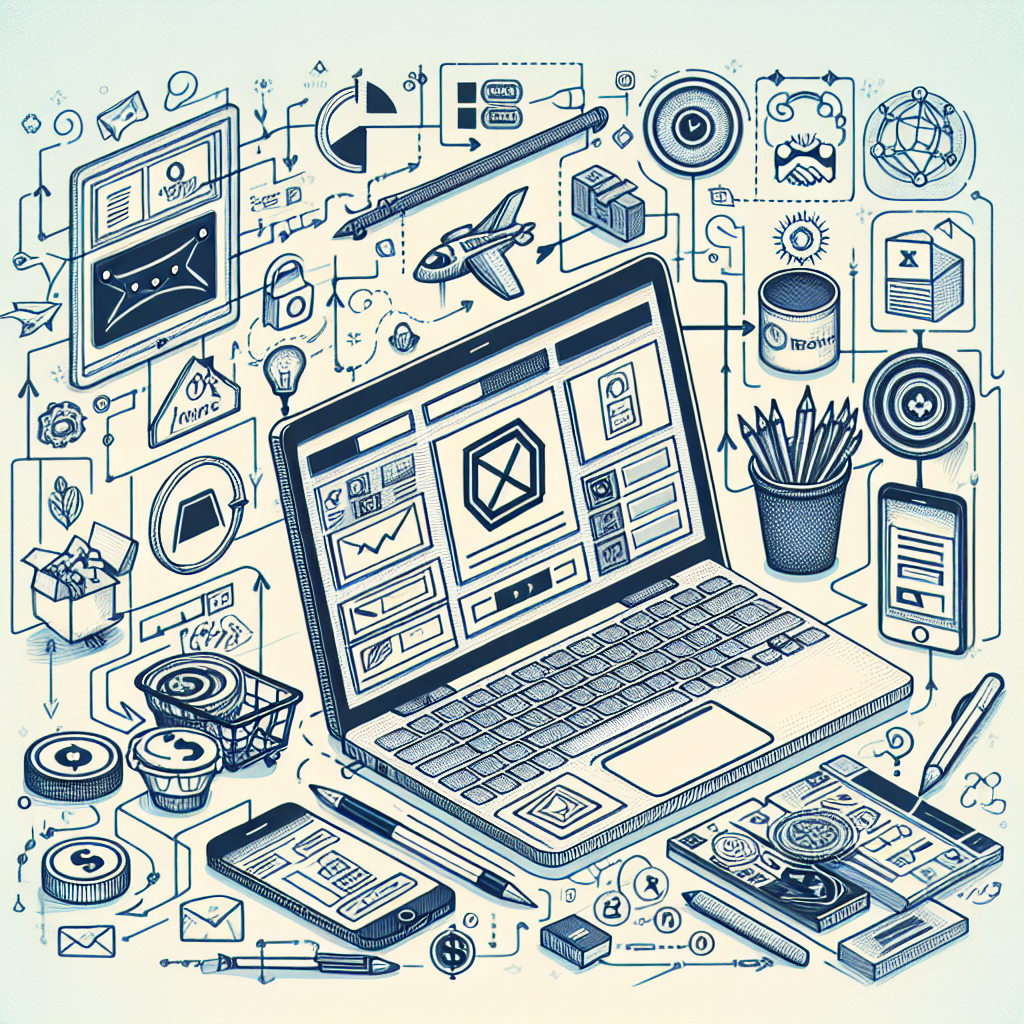
How to Create a Custom Product Type in Magento 2
Magento 2 is a powerful eCommerce platform, but sometimes the default product types just don’t cut it. Maybe you need a subscription-based product, a customizable bundle, or something entirely unique. That’s where custom product types come in!
In this guide, we’ll walk through the process of creating a custom product type in Magento 2 step by step. Whether you're a developer or just curious about how it works, we’ll keep things simple and practical.
Why Create a Custom Product Type?
Magento 2 comes with several built-in product types—Simple, Configurable, Grouped, Virtual, Downloadable, and Bundle. But what if your store sells something more specialized? For example:
- Membership plans
- Rental products
- Custom-made items with unique attributes
A custom product type lets you define exactly how your product behaves in the catalog, cart, and checkout.
Step 1: Set Up the Module Structure
First, we need to create a new Magento 2 module. Here’s the basic structure:
app/code/Vendor/ProductType/
├── etc/
│ ├── module.xml
│ └── product_types.xml
├── Model/
│ └── Product/
│ └── Type/
│ └── Custom.php
└── registration.php
Let’s break this down:
1. registration.php
<?php
use Magento\Framework\Component\ComponentRegistrar;
ComponentRegistrar::register(
ComponentRegistrar::MODULE,
'Vendor_ProductType',
__DIR__
);
2. module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_ProductType" setup_version="1.0.0">
<sequence>
<module name="Magento_Catalog"/>
</sequence>
</module>
</config>
3. product_types.xml
This file defines our new product type:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Catalog:etc/product_types.xsd">
<type name="custom" label="Custom Product" model="Vendor\ProductType\Model\Product\Type\Custom" isQty="true" />
</config>
Step 2: Create the Product Type Model
The real magic happens in the Custom.php file. This class extends Magento\Catalog\Model\Product\Type\AbstractType
and defines how our product behaves.
<?php
namespace Vendor\ProductType\Model\Product\Type;
use Magento\Catalog\Model\Product\Type\AbstractType;
class Custom extends AbstractType
{
const TYPE_CODE = 'custom';
public function deleteTypeSpecificData(\Magento\Catalog\Model\Product $product)
{
// Handle custom product deletion logic here
}
public function isSalable($product)
{
return parent::isSalable($product) && $product->getData('custom_available');
}
}
Step 3: Enable the Product Type in Admin
Now, we need to make sure our product type appears in the Magento admin panel. We’ll use a plugin to modify the product type options.
Create di.xml in app/code/Vendor/ProductType/etc/
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Catalog\Model\Product\Type">
<plugin name="add_custom_product_type" type="Vendor\ProductType\Plugin\Product\Type" />
</type>
</config>
Then, create the plugin class:
<?php
namespace Vendor\ProductType\Plugin\Product;
class Type
{
public function afterGetOptionArray(\Magento\Catalog\Model\Product\Type $subject, array $options)
{
$options['custom'] = __('Custom Product');
return $options;
}
}
Step 4: Test Your Custom Product Type
After running bin/magento setup:upgrade
, go to your Magento admin panel and create a new product. You should now see "Custom Product" as an option in the product type dropdown.
Final Thoughts
Creating a custom product type in Magento 2 might seem complex at first, but once you break it down, it’s totally manageable. With this setup, you can define unique behaviors for your products, making your store more flexible and tailored to your business needs.
Need help with Magento 2 development? Check out our Magento extensions and hosting solutions to optimize your store!