The Role of Message Queues in Magento 2: How to Use Them Effectively
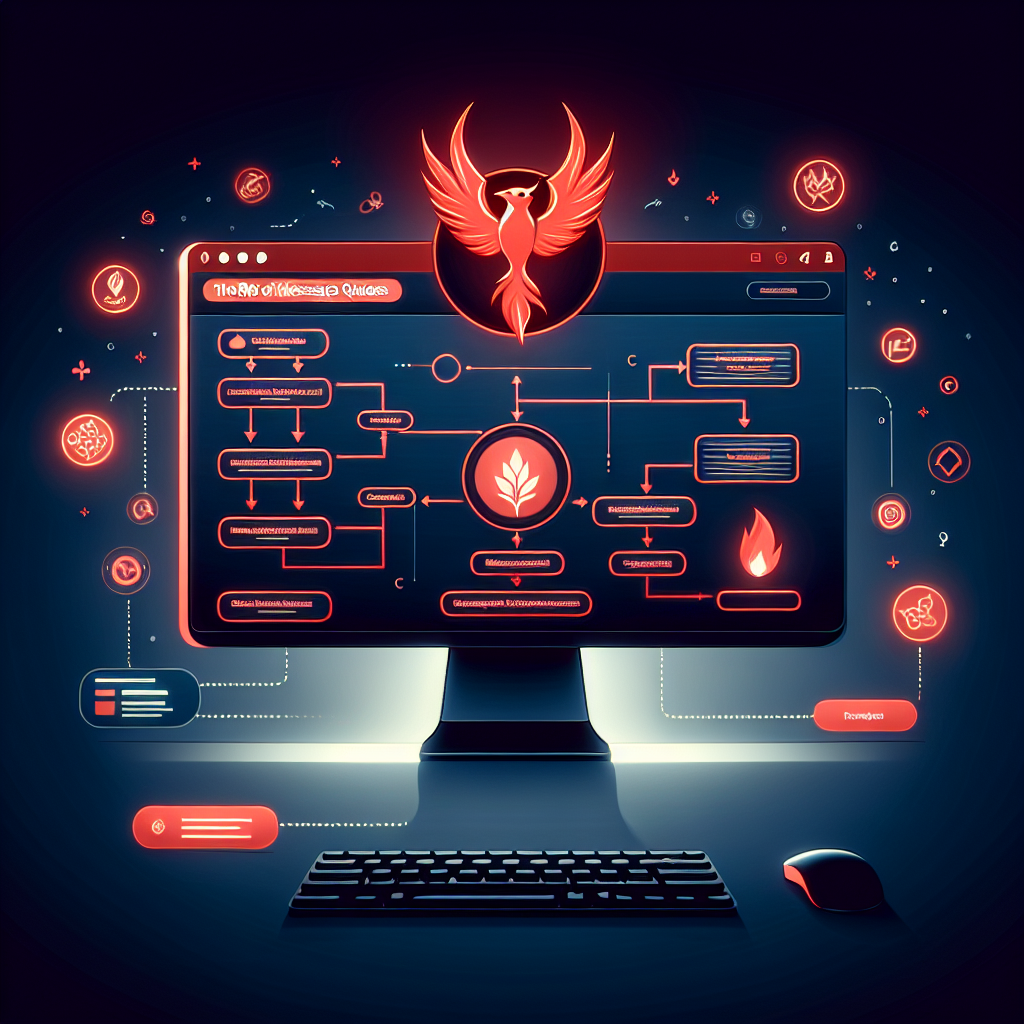
Understanding Message Queues in Magento 2
Message queues in Magento 2 are like a digital waiting line where tasks are processed one by one, ensuring smooth performance even during high traffic. Instead of executing everything at once, Magento queues tasks like order processing, inventory updates, or email notifications, preventing server overload.
Think of it as a restaurant kitchen: instead of preparing all dishes simultaneously (which would overwhelm the chefs), orders are queued and handled efficiently. This improves scalability, reduces errors, and keeps your store running smoothly.
Why Use Message Queues?
Here’s why message queues are a game-changer for Magento 2 stores:
- Better Performance: Heavy tasks (like sending bulk emails) don’t slow down your store.
- Scalability: Handles spikes in traffic without crashing.
- Reliability: If a task fails, it retries automatically.
- Asynchronous Processing: Customers don’t wait for backend tasks to finish.
How Magento 2 Implements Message Queues
Magento uses RabbitMQ (or MySQL as a fallback) to manage queues. Here’s how it works:
- A publisher (like an order placement) adds a task to the queue.
- The consumer processes tasks in the background.
- Tasks are retried if they fail.
Setting Up RabbitMQ for Magento 2
Before diving into code, ensure RabbitMQ is installed and configured:
# Install RabbitMQ (Ubuntu example)
sudo apt-get install rabbitmq-server
sudo systemctl enable rabbitmq-server
sudo systemctl start rabbitmq-server
Next, update your env.php
to enable RabbitMQ:
'queue' => [
'amqp' => [
'host' => 'localhost',
'port' => '5672',
'user' => 'guest',
'password' => 'guest',
'virtualhost' => '/'
]
]
Creating a Custom Message Queue
Let’s build a queue to process product updates. Here’s a step-by-step guide:
Step 1: Define the Queue in queue.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework-message-queue:etc/queue.xsd">
<broker topic="magefine.product.update" exchange="magento">
<queue name="magefine_product_update" consumer="magefineProductUpdateConsumer"/>
</broker>
</config>
Step 2: Create a Publisher
Add this to your Observer or Service class to publish tasks:
use Magento\Framework\MessageQueue\PublisherInterface;
class ProductUpdater
{
private $publisher;
public function __construct(PublisherInterface $publisher)
{
$this->publisher = $publisher;
}
public function publishUpdate($productId)
{
$this->publisher->publish('magefine.product.update', $productId);
}
}
Step 3: Build the Consumer
Create a consumer class to process queued tasks:
use Magento\Framework\MessageQueue\ConsumerInterface;
class ProductUpdateConsumer implements ConsumerInterface
{
public function process($productId)
{
// Logic to update the product (e.g., stock sync)
echo "Processing product ID: " . $productId;
}
}
Step 4: Register the Consumer
Add this to your di.xml
:
<type name="Magento\Framework\MessageQueue\ConsumerInterface">
<arguments>
<argument name="handlers" xsi:type="array">
<item name="magefineProductUpdateConsumer" xsi:type="object">Vendor\Module\Model\ProductUpdateConsumer</item>
</argument>
</arguments>
</type>
Running Consumers
Start the consumer via CLI:
php bin/magento queue:consumers:start magefine_product_update
For production, use Supervisor to keep consumers running:
[program:magento_consumer]
command=php /var/www/html/bin/magento queue:consumers:start magefine_product_update
user=www-data
numprocs=2
autostart=true
autorestart=true
Debugging Message Queues
Common issues and fixes:
- Consumer not running: Check RabbitMQ logs (
sudo tail -f /var/log/rabbitmq/rabbitmq.log
). - Tasks stuck: Clear the queue:
php bin/magento queue:consumers:list
+ restart. - Performance lag: Tune RabbitMQ’s memory limits.
Best Practices
- Limit queue size: Avoid overloading with too many tasks.
- Monitor queues: Use tools like
rabbitmqctl list_queues
. - Prioritize critical tasks: Split queues (e.g.,
order_processing
vs.newsletter
).
Final Thoughts
Message queues are Magento 2’s unsung heroes—keeping your store fast and reliable. Whether you’re syncing inventory or sending emails, queues ensure tasks run smoothly without blocking customers. Ready to optimize? Start with RabbitMQ and let your store handle traffic like a pro!
Need help? Magefine offers hosting and extensions to supercharge your Magento 2 performance.