Unveiling the Power of Magento 2 Module Base Classes
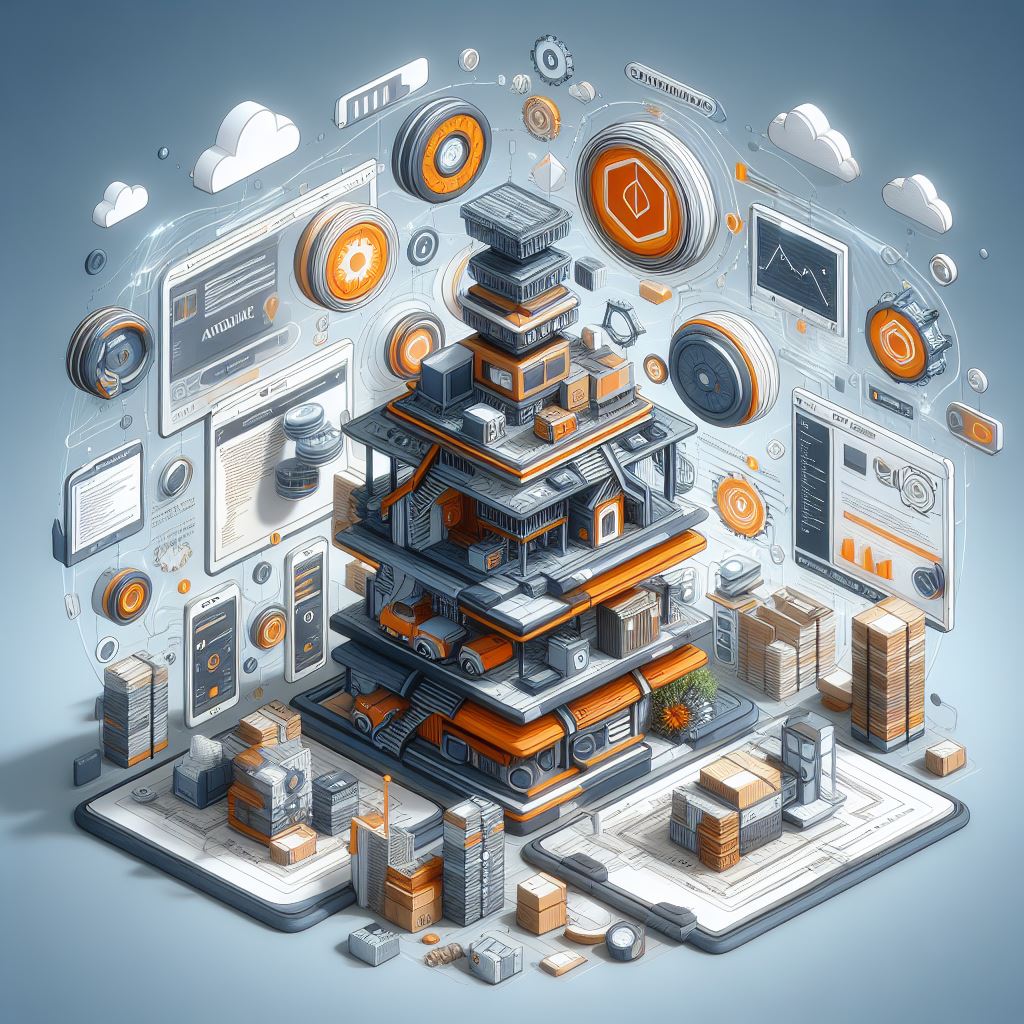
Introduction
In the ever-evolving world of e-commerce, Magento 2 reigns as a prominent and robust platform, empowering online businesses to thrive. At the heart of Magento 2's flexibility and extensibility lies its modular architecture. Modules are the building blocks that make it possible to customize and extend the platform to suit unique business needs. Within these modules, base classes play a pivotal role in defining the structure and functionality of your e-commerce website.
This comprehensive article will be your guide to understanding the core base classes of a Magento 2 module. We will delve into each of these classes, shedding light on their significance and how they contribute to the development of a successful online store. From Blocks to Repositories, we will explore the purpose and functionality of these essential building blocks.
In the vast landscape of Magento 2 module development, the Block class stands as a fundamental and indispensable component. It serves as the linchpin in shaping the frontend of your e-commerce store, enabling the rendering of HTML content while defining the structure and placement of elements on webpages.
Understanding the Block Class
At its core, the Block class in Magento 2 is the cornerstone of your frontend design. When creating a custom block, developers usually extend the Magento\Framework\View\Element\Template class. This base class equips your block with a robust set of methods and properties, essential for seamless interactions with layouts and templates.
<?php
namespace YourVendor\YourModule\Block;
use Magento\Framework\View\Element\Template;
class CustomBlock extends Template
{
// Your custom block logic here
}
In the example above, CustomBlock extends the Template class, inheriting its foundational functionalities. You can then enhance the block with your custom logic to tailor it precisely to your requirements.
Interactions with Layouts
Magento 2 Blocks and layouts are intricately connected, with their relationship defined through XML layout files. Blocks interact with layouts by specifying their template file and position within the layout structure. Here's how a custom block integrates into a layout XML file:
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<block class="YourVendor\YourModule\Block\CustomBlock" name="custom.block" template="YourVendor_YourModule::custom_template.phtml" />
</referenceContainer>
</body>
</page>
Within this XML snippet, the <block> element assumes vital roles:
class: Specifies the block's class, enabling Magento to identify and instantiate it.
template: Points to the block's associated template file, which dictates its visual appearance.
name: Provides a unique identifier for the block within the layout, simplifying further customization and reference.
In Summary
Magento 2 Blocks are the architects of your e-commerce store's frontend. They hold the power to create visually captivating and functionally dynamic shopping experiences for your customers. Gaining a firm grasp of the Block class and its interplay with layouts is paramount to leveraging the full potential of Magento 2's frontend capabilities.
Understanding the Helper Class
The Helper class in Magento 2 is a utility class that doesn't adhere to any specific area of your codebase. Instead, it acts as a toolbox of functions and methods that can be used throughout your module. Its purpose is to provide a centralized location for commonly used functions, helping you avoid code repetition and maintain a more organized codebase.
To create a custom Helper class in Magento 2, you typically extend the Magento\Framework\App\Helper\AbstractHelper class:
<?php
namespace YourVendor\YourModule\Helper;
use Magento\Framework\App\Helper\AbstractHelper;
class Data extends AbstractHelper
{
// Your custom helper methods here
}
Here, YourVendor\YourModule\Helper\Data becomes your custom Helper class, and you can add your own methods and functions to it.
Versatility of Helper Methods
The beauty of the Helper class lies in its versatility. It can assist with various tasks, such as:
Configuration Retrieval: Helpers are often used to retrieve configuration values, making it easy to access and manage settings specific to your module.
Data Formatting: You can use Helper methods to format data for display, ensuring consistency in your store's appearance.
Common Operations: Helpers are perfect for encapsulating common operations that are used in multiple places within your module. This promotes code reusability and maintainability.
String Manipulation: If your module involves working with strings, Helpers can contain functions for string manipulation, making your code more readable and efficient.
Interaction with the Module
Helpers are not directly linked to layouts like Blocks, but they often work in tandem with Blocks and other classes to provide data and functionality. For instance, a Helper might be responsible for fetching configuration values from the database and passing them to a Block for rendering.
Understanding the Model Class
The Model class in Magento 2 is the backbone of data management. It's responsible for encapsulating business logic and interacting with the database. Models define the structure and behavior of data entities, ensuring data integrity and providing a foundation for your module's functionality.
Creating a custom Model in Magento 2 is a common practice. You typically extend the Magento\Framework\Model\AbstractModel class to build your own:
<?php
namespace YourVendor\YourModule\Model;
use Magento\Framework\Model\AbstractModel;
class CustomModel extends AbstractModel
{
// Your custom Model logic here
}
In this example, CustomModel becomes your custom Model class, and you can add your own methods and functions to handle data manipulation and business logic.
Roles of Models
Data Access: Models are responsible for reading and writing data to and from the database. They abstract the database schema and provide a clear API for other parts of your module to interact with data.
Business Logic: Complex business logic, such as calculating prices, validating data, and enforcing rules, can be encapsulated within Models. This promotes code organization and maintainability.
Data Validation: Models often include data validation methods, ensuring that data entering or leaving your module adheres to specific rules and constraints.
Database Operations: Models facilitate CRUD (Create, Read, Update, Delete) operations on data entities. They simplify database queries and provide an object-oriented approach to data manipulation.
Understanding the Collection Class
The Collection class in Magento 2 is the bridge between Models and the database. It acts as a powerful tool for retrieving and managing sets of database records. Collections are typically used when you need to work with multiple records, such as when querying for products, customers, or orders.
Creating a custom Collection in Magento 2 involves extending the Magento\Framework\Model\ResourceModel\Db\Collection\AbstractCollection class:
<?php
namespace YourVendor\YourModule\Model\ResourceModel\CustomModel;
use Magento\Framework\Model\ResourceModel\Db\Collection\AbstractCollection;
class Collection extends AbstractCollection
{
protected $_idFieldName = 'entity_id';
protected $_eventPrefix = 'yourmodule_custommodel_collection';
protected function _construct()
{
$this->_init(
\YourVendor\YourModule\Model\CustomModel::class,
\YourVendor\YourModule\Model\ResourceModel\CustomModel::class
);
}
// Custom methods and filters can be added here
}
In this example, YourVendor\YourModule\Model\ResourceModel\CustomModel\Collection represents your custom Collection class, associated with a specific data entity.
Roles of Collections
Data Retrieval: Collections allow you to efficiently retrieve data from the database. They abstract complex SQL queries and provide a convenient API for fetching records.
Filtering and Sorting: Collections support filtering and sorting operations, enabling you to retrieve precisely the data you need. For instance, you can filter products by category or sort orders by date.
Data Manipulation: Collections are also handy for mass data updates or deletions. You can iterate over a collection and apply changes to multiple records simultaneously.
Pagination: When dealing with large datasets, Collections support pagination, ensuring efficient data retrieval without overloading server resources.
Interaction with Models
Collections and Models in Magento 2 often work hand in hand:
Data Loading: Models can use Collections to load related data efficiently. For example, when loading a customer's orders, a Model might use a Collection to fetch the order records.
Data Presentation: Collections can provide data to Models, which can then be used to populate Blocks and Views in the frontend.
Understanding the Controller Class
The Controller class in Magento 2 is the gatekeeper that manages incoming HTTP requests and dispatches them to the appropriate actions. Controllers are responsible for handling user interactions, processing form submissions, and rendering views. They act as intermediaries between the frontend and the backend of your e-commerce website.
Creating a custom Controller in Magento 2 involves extending the Magento\Framework\App\Action\Action class:
<?php
namespace YourVendor\YourModule\Controller\Custom;
use Magento\Framework\App\Action\Action;
use Magento\Framework\App\Action\Context;
class Index extends Action
{
public function __construct(Context $context)
{
parent::__construct($context);
}
public function execute()
{
// Your custom Controller logic here
}
}
In this example, YourVendor\YourModule\Controller\Custom\Index represents your custom Controller class. The execute method contains the logic to be executed when a specific URL is accessed.
Roles of Controllers
Request Handling: Controllers are responsible for handling HTTP requests from users. They analyze the request parameters, determine the appropriate action, and execute it.
Action Execution: Controllers dispatch requests to specific actions, which are methods within the Controller class. These actions perform tasks like rendering pages, processing forms, or interacting with Models and data.
View Rendering: Controllers often render views by generating HTML content and passing data to Blocks and Templates. They define the layout and structure of the pages users see.
URL Routing: Controllers map URLs to specific actions, allowing for clean and user-friendly URLs. For example, a Controller might route "yourmodule/custom/index" to the execute method of the Index class.
Understanding the Repository Class
The Repository class in Magento 2 serves as an abstraction layer for database operations related to a specific entity. It encapsulates the complexity of data retrieval, creation, updating, and deletion, offering a clean and consistent API for working with data entities.
Creating a custom Repository in Magento 2 typically involves defining an interface and a concrete implementation:
<?php
namespace YourVendor\YourModule\Api;
interface CustomEntityRepositoryInterface
{
// Define repository methods here
}
<?php
namespace YourVendor\YourModule\Model;
use YourVendor\YourModule\Api\CustomEntityRepositoryInterface;
use YourVendor\YourModule\Model\ResourceModel\CustomEntity as ResourceModel;
use YourVendor\YourModule\Model\ResourceModel\CustomEntity\CollectionFactory;
class CustomEntityRepository implements CustomEntityRepositoryInterface
{
// Implement repository methods here
}
In this example, YourVendor\YourModule\Api\CustomEntityRepositoryInterface defines the interface for your custom Repository, while YourVendor\YourModule\Model\CustomEntityRepository provides the concrete implementation.
Roles of Repositories
Data Access Abstraction: Repositories abstract data access operations, making it easy to work with entities without the need to write complex SQL queries.
Data Retrieval: Repositories provide methods to retrieve data entities based on specific criteria, such as filtering by attribute values or searching by keywords.
Data Creation and Updating: Repositories allow for the creation of new entities and updating existing ones, ensuring data integrity and consistency.
Data Deletion: Repositories facilitate the removal of entities from the database when they are no longer needed.
Validation: Repositories often include data validation to ensure that data entered or modified adheres to specific rules and constraints.
Conclusion
In the intricate world of Magento 2 module development, each class serves as a unique piece of the puzzle, contributing to the creation of robust and feature-rich e-commerce solutions. In this comprehensive exploration, we've delved into the roles and functionalities of essential base classes within Magento 2 modules: Blocks, Helpers, Controllers, Models, Resource Models, Collections, and Repositories.
Blocks emerged as the presentation layer experts, responsible for rendering content and orchestrating the visual aspects of your e-commerce store. They play a crucial role in shaping the frontend by linking with layout XML files and providing a bridge between business logic and user interfaces.
Helpers stood out as the versatile Swiss Army knives, offering a repository of utility functions and methods for common tasks. Their role extends to data formatting, configuration retrieval, and encapsulating common operations, promoting code reusability and maintainability.
Controllers assumed the role of navigators, managing HTTP requests, executing actions, and rendering views. They act as intermediaries between user interactions and the backend, ensuring smooth user experiences and efficient data flow.
Models became the backbone of data management, encapsulating business logic, interacting with the database, and defining the structure and behavior of data entities. They ensure data integrity and provide a foundation for module functionality.
Resource Models and Collections worked hand in hand to facilitate data retrieval, manipulation, and organization. Resource Models abstracted database operations, while Collections offered efficient tools for managing sets of records.
Repositories emerged as the data access facilitators, simplifying complex database operations related to specific entities. They provided a clean and consistent API for working with data, abstracting data access complexity.
The synergy of these classes is the cornerstone of Magento 2's power and flexibility. Each class plays a vital role in simplifying complex tasks, promoting code organization, and ensuring the seamless operation of your e-commerce store.
As you embark on your Magento 2 development journey, a deep understanding of these classes will empower you to create tailored, efficient, and scalable e-commerce solutions that cater to the unique needs of your business. By harnessing the capabilities of Blocks, Helpers, Controllers, Models, Resource Models, Collections, and Repositories, you can unlock the full potential of Magento 2 and deliver exceptional shopping experiences to your customers.
In conclusion, Magento 2's modular architecture and well-defined classes pave the way for innovation and customization, making it a formidable platform for building and expanding e-commerce ventures. Embrace the possibilities offered by these base classes, and you'll be well-equipped to navigate the ever-evolving landscape of online retail, driving success and growth in the competitive e-commerce market.