Creating a New Model with a Database Table in Magento 2
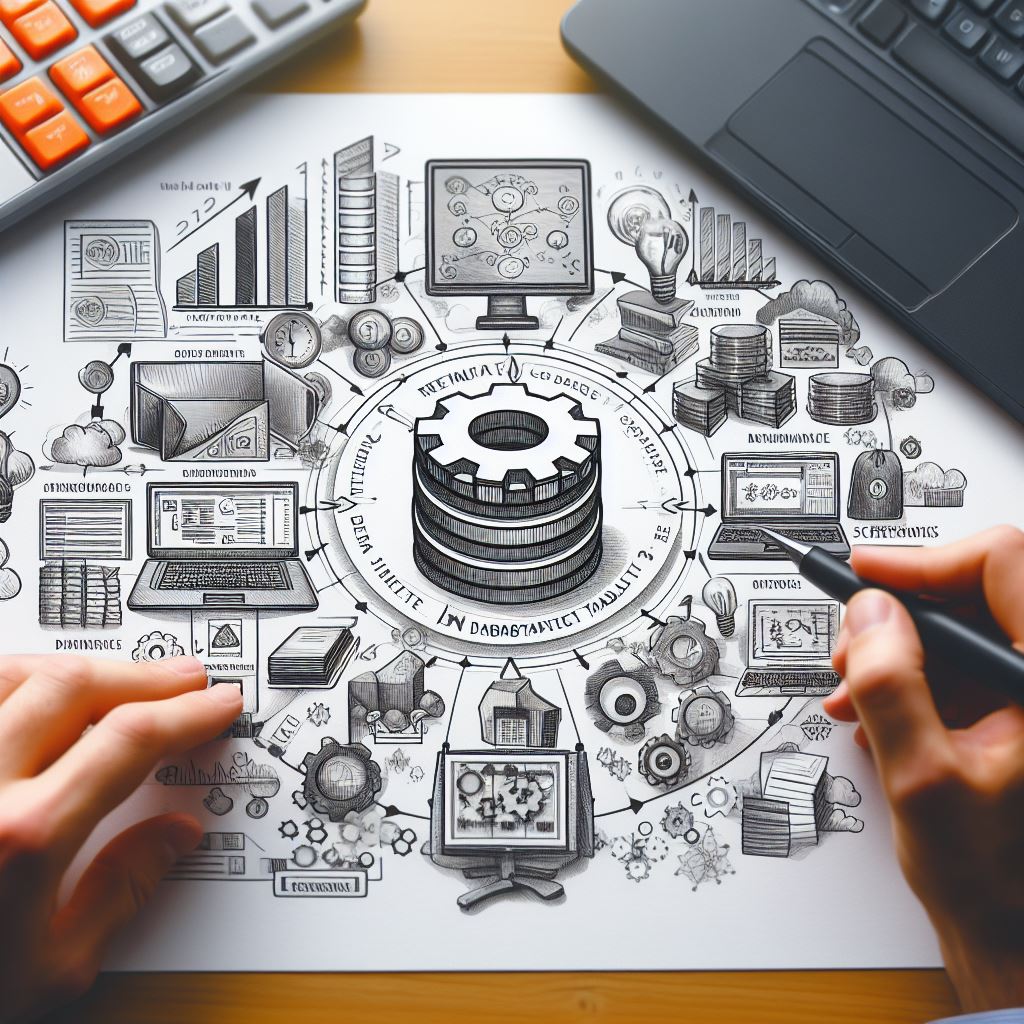
In the world of Magento 2 e-commerce development, there comes a time when you need to extend your store's functionality by creating a new model with its associated database table. This is a crucial skill for developers and e-commerce experts looking to customize and enhance their Magento 2 websites. In this comprehensive guide, we'll walk you through the process of creating a new model and database table within Magento 2.
Why Create a New Model and Table?
Creating a custom model and database table is essential when you need to store specific data for your extension or customization. It's a fundamental part of Magento 2 development and allows you to manage and retrieve data in an organized manner.
Steps to Create a Model and Database Table
- Step 1: Create a Custom Module
In your Magento 2 project root directory, navigate to the app/code directory.
Create a directory for your custom module, following the naming convention VendorName_ModuleName. For example, if your company name is "MyCompany" and your module is called "CustomModule," the directory structure would be app/code/MyCompany/CustomModule.
- Step 2: Define Your Model
Inside your custom module directory, create a new directory called Model. This is where your model class will reside. The path should look like this: app/code/MyCompany/CustomModule/Model.
Now, create your model class in this directory. The class should extend the Magento\Framework\Model\AbstractModel class:
// app/code/MyCompany/CustomModule/Model/MyModel.php
namespace MyCompany\CustomModule\Model;
use Magento\Framework\Model\AbstractModel;
class MyModel extends AbstractModel
{
protected function _construct()
{
$this->_init('MyCompany\CustomModule\Model\ResourceModel\MyModel');
}
}
- Step 3: Create db_schema.xml
Inside your custom module directory, create a new directory called etc. This is where you'll define the db_schema.xml file. The path should be: app/code/MyCompany/CustomModule/etc.
Now, create the db_schema.xml file inside the etc directory. This file will define the structure of your database table. Here's an example:
<!-- app/code/MyCompany/CustomModule/etc/db_schema.xml -->
<?xml version="1.0" ?>
<schema xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Setup/Declaration/Schema/etc/schema.xsd">
<table name="my_table_name" comment="My Custom Table">
<column xsi:type="int" name="entity_id" padding="10" unsigned="true" nullable="false" identity="true" comment="Entity ID"/>
<column xsi:type="varchar" name="name" nullable="false" length="255" comment="Name"/>
<column xsi:type="text" name="description" nullable="true" comment="Description"/>
<constraint xsi:type="primary" referenceId="PRIMARY">
<column name="entity_id"/>
</constraint>
</table>
</schema>
Replace 'my_table_name' with the actual name you want to use for your table.
- Step 4: Run the Database Upgrade
Now that you've defined your database schema using db_schema.xml, you need to run the setup upgrade to create the table in the database. Run the following command from your Magento 2 project root directory:
php bin/magento setup:upgrade
This command will execute the database schema changes defined in your db_schema.xml file.
- Step 5: Interact with Your Model and Table
With your model and table in place, you can now interact with them in your custom extension. You can use dependency injection to inject your model into other parts of your extension and perform CRUD (Create, Read, Update, Delete) operations on your custom table.
Conclusion
Creating a new model with its associated database table in Magento 2 is a valuable skill for e-commerce developers and experts. It allows you to manage and retrieve data efficiently while adhering to best practices in Magento 2 development.
With this knowledge, you can take your Magento 2 customization and extension development to the next level, enhancing the capabilities of your online store.
Happy coding in Magento 2!