Magento 2 and Gamification: Boosting Engagement with Rewards & Badges
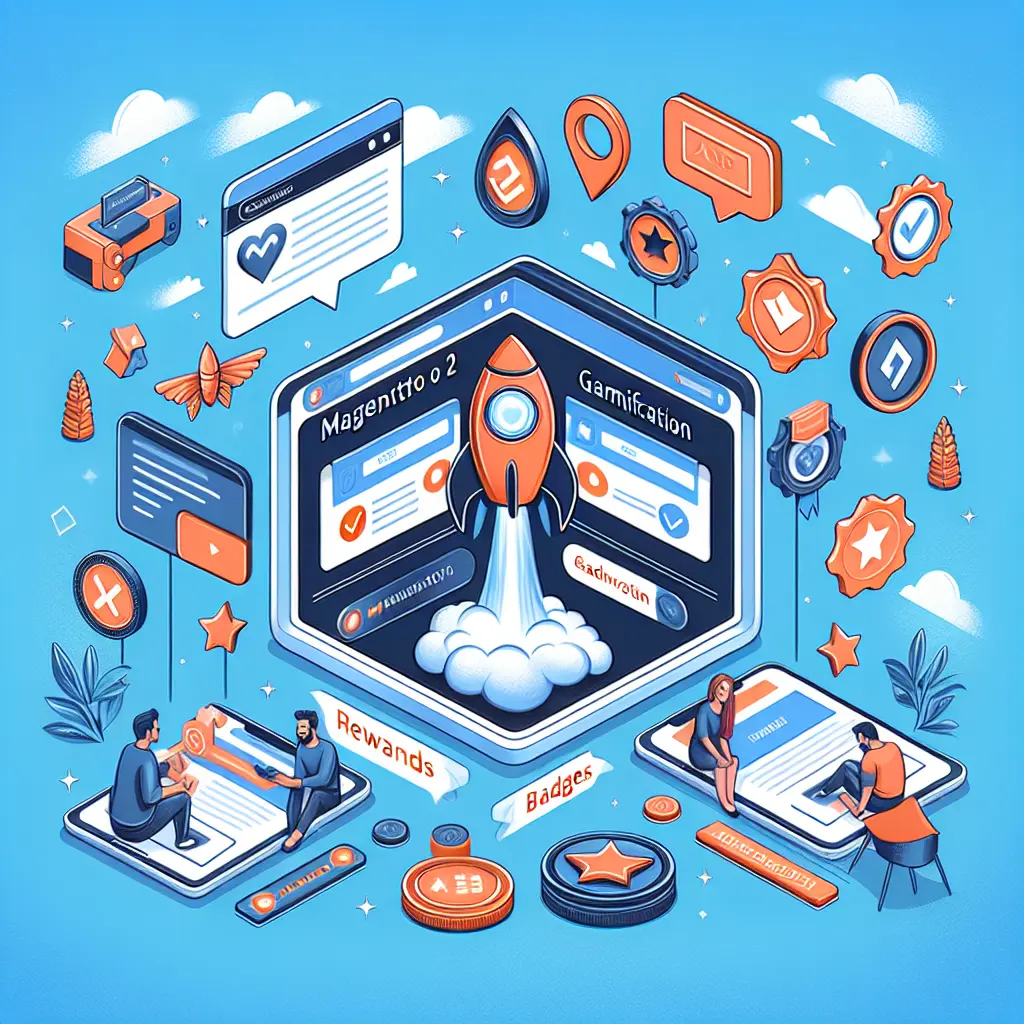
Magento 2 and Gamification: Boosting Engagement with Rewards & Badges
Gamification isn’t just for mobile apps and social networks—it’s a powerful tool for eCommerce too. By integrating game-like elements into your Magento 2 store, you can boost customer engagement, encourage repeat purchases, and foster brand loyalty. Think points, badges, leaderboards, and exclusive rewards that turn shopping into an interactive experience.
In this post, we’ll explore how to implement gamification in Magento 2, from simple reward systems to advanced badge mechanics. Whether you're a developer or a store owner, you’ll find actionable steps (and code snippets!) to get started.
Why Gamification Works in eCommerce
Gamification taps into basic human psychology—competition, achievement, and instant gratification. When customers earn points for purchases, unlock badges for milestones, or see their name on a leaderboard, they’re more likely to return and engage with your store. Here’s what it can do for you:
- Increase repeat purchases – Customers work toward rewards, making them more likely to buy again.
- Encourage social sharing – Badges and achievements can be shared on social media, driving free traffic.
- Improve customer retention – A fun, interactive experience keeps shoppers coming back.
Basic Gamification: Implementing a Points System
Let’s start with a simple points system where customers earn rewards for purchases. We’ll use Magento 2’s built-in features and some custom code.
Step 1: Create a Custom Module for Rewards
First, set up a basic module structure in app/code/Magefine/Rewards
:
// app/code/Magefine/Rewards/registration.php
Next, define the module in app/code/Magefine/Rewards/etc/module.xml
:
Step 2: Add a Customer Attribute for Points
We’ll store points as a custom customer attribute. Create an install script:
// app/code/Magefine/Rewards/Setup/InstallData.php
customerSetupFactory = $customerSetupFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerSetup->addAttribute(
Customer::ENTITY,
'reward_points',
[
'type' => 'int',
'label' => 'Reward Points',
'input' => 'text',
'required' => false,
'visible' => true,
'system' => false,
'default' => 0
]
);
$setup->endSetup();
}
}
Step 3: Award Points on Order Completion
Now, let’s automatically add points when a customer places an order. Create an observer:
// app/code/Magefine/Rewards/Observer/AddRewardPoints.php
customerFactory = $customerFactory;
}
public function execute(Observer $observer)
{
$order = $observer->getEvent()->getOrder();
$customerId = $order->getCustomerId();
if ($customerId) {
$customer = $this->customerFactory->create()->load($customerId);
$currentPoints = $customer->getData('reward_points') ?? 0;
$newPoints = $currentPoints + (int)($order->getGrandTotal()); // 1 point per $1 spent
$customer->setData('reward_points', $newPoints)->save();
}
}
}
Register the observer in app/code/Magefine/Rewards/etc/events.xml
:
Advanced Gamification: Badges & Achievements
Now, let’s level up with badges. Customers can unlock achievements like "First Purchase," "Loyal Customer," or "Big Spender."
Step 1: Create a Badge Database Table
Set up a table to store badges and customer achievements:
// app/code/Magefine/Rewards/Setup/InstallSchema.php
startSetup();
// Badges table
$setup->getConnection()->createTable(
$setup->getConnection()
->newTable($setup->getTable('magefine_rewards_badges'))
->addColumn(
'badge_id',
Table::TYPE_INTEGER,
null,
['identity' => true, 'unsigned' => true, 'nullable' => false, 'primary' => true],
'Badge ID'
)
->addColumn(
'name',
Table::TYPE_TEXT,
255,
['nullable' => false],
'Badge Name'
)
->addColumn(
'description',
Table::TYPE_TEXT,
'64k',
['nullable' => false],
'Badge Description'
)
->addColumn(
'image_url',
Table::TYPE_TEXT,
255,
['nullable' => false],
'Badge Image URL'
)
);
// Customer badges table
$setup->getConnection()->createTable(
$setup->getConnection()
->newTable($setup->getTable('magefine_rewards_customer_badges'))
->addColumn(
'id',
Table::TYPE_INTEGER,
null,
['identity' => true, 'unsigned' => true, 'nullable' => false, 'primary' => true],
'ID'
)
->addColumn(
'customer_id',
Table::TYPE_INTEGER,
null,
['unsigned' => true, 'nullable' => false],
'Customer ID'
)
->addColumn(
'badge_id',
Table::TYPE_INTEGER,
null,
['unsigned' => true, 'nullable' => false],
'Badge ID'
)
->addColumn(
'earned_at',
Table::TYPE_TIMESTAMP,
null,
['nullable' => false, 'default' => Table::TIMESTAMP_INIT],
'Earned At'
)
->addForeignKey(
$setup->getFkName(
'magefine_rewards_customer_badges',
'customer_id',
'customer_entity',
'entity_id'
),
'customer_id',
$setup->getTable('customer_entity'),
'entity_id',
Table::ACTION_CASCADE
)
->addForeignKey(
$setup->getFkName(
'magefine_rewards_customer_badges',
'badge_id',
'magefine_rewards_badges',
'badge_id'
),
'badge_id',
$setup->getTable('magefine_rewards_badges'),
'badge_id',
Table::ACTION_CASCADE
)
);
$setup->endSetup();
}
}
Step 2: Create a Badge Model & Check Conditions
Now, let’s define a service to check if a customer qualifies for a badge:
// app/code/Magefine/Rewards/Model/BadgeChecker.php
customerFactory = $customerFactory;
$this->orderCollectionFactory = $orderCollectionFactory;
$this->badgeCollectionFactory = $badgeCollectionFactory;
$this->customerBadgeCollectionFactory = $customerBadgeCollectionFactory;
}
public function checkForNewBadges($customerId)
{
$customer = $this->customerFactory->create()->load($customerId);
$badges = $this->badgeCollectionFactory->create();
foreach ($badges as $badge) {
if (!$this->hasBadge($customerId, $badge->getId())) {
if ($this->meetsBadgeCondition($customer, $badge)) {
$this->assignBadge($customerId, $badge->getId());
}
}
}
}
protected function hasBadge($customerId, $badgeId)
{
$collection = $this->customerBadgeCollectionFactory->create()
->addFieldToFilter('customer_id', $customerId)
->addFieldToFilter('badge_id', $badgeId);
return $collection->getSize() > 0;
}
protected function meetsBadgeCondition($customer, $badge)
{
// Example: Check if customer has made 5+ orders
$orderCount = $this->orderCollectionFactory->create()
->addFieldToFilter('customer_id', $customer->getId())
->getSize();
return $orderCount >= 5; // "Loyal Customer" badge
}
protected function assignBadge($customerId, $badgeId)
{
// Logic to save badge assignment
}
}
Displaying Rewards & Badges in the Frontend
Now, let’s show customers their progress. Create a block and template to display points and badges in the customer dashboard.
// app/code/Magefine/Rewards/Block/Customer/Rewards.php
customerSession = $customerSession;
parent::__construct($context, $data);
}
public function getRewardPoints()
{
$customer = $this->customerSession->getCustomer();
return $customer->getData('reward_points') ?? 0;
}
}
Then, create a template at app/code/Magefine/Rewards/view/frontend/templates/customer/rewards.phtml
:
<div class="rewards-dashboard">
<h2>Your Rewards</h2>
<p>You have <strong><?= $block->getRewardPoints() ?></strong> points.</p>
<?php // Add badge display logic here ?>
</div>
Final Thoughts
Gamification in Magento 2 doesn’t have to be complicated. Start with a simple points system, then expand to badges and leaderboards as you see engagement grow. The code examples above should give you a solid foundation—customize them to fit your store’s unique needs!
Want a ready-made solution? Check out Magefine’s extensions for pre-built gamification modules that save development time.
Have you tried gamification in your store? Share your experiences in the comments!