Magento 2 and Blockchain-Based Supply Chain Tracking
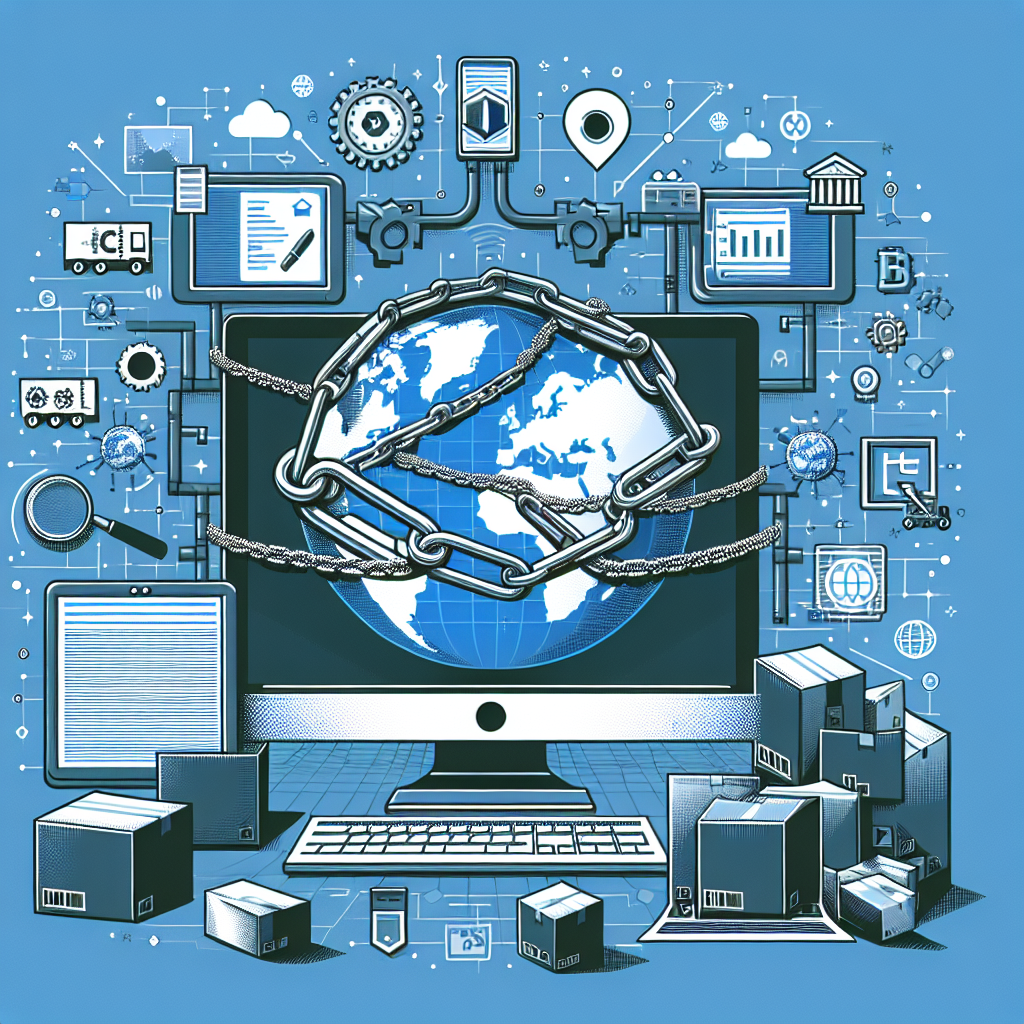
Magento 2 and Blockchain-Based Supply Chain Tracking
Imagine knowing exactly where every product in your Magento store comes from, who handled it, and when – all in real time. That's the power of combining Magento 2 with blockchain technology for supply chain tracking. For store owners who want to build trust with customers and streamline operations, this is a game-changer.
In this post, we'll break down how blockchain works with Magento 2, why it matters for your business, and how to implement it step by step – even if you're new to the concept.
Why Blockchain for Supply Chain?
Blockchain is essentially a digital ledger that records transactions in a way that's secure, transparent, and tamper-proof. When applied to supply chains, it means:
- ✅ Full traceability – Track products from manufacturer to customer
- ✅ Reduced fraud – Prevent counterfeit goods from entering your supply chain
- ✅ Automated verification – Smart contracts can validate shipments automatically
- ✅ Improved trust – Customers can verify product authenticity themselves
How Magento 2 Integrates with Blockchain
Magento 2 doesn't have built-in blockchain functionality (yet), but with some custom development or the right extensions, you can connect it to blockchain networks. Here's the basic architecture:
- Products are assigned unique identifiers (like QR codes or RFID tags)
- Each movement (manufacturing, shipping, delivery) is recorded on the blockchain
- Magento pulls this data to display to customers
- Smart contracts can trigger actions (like payments) when conditions are met
Setting Up Basic Blockchain Tracking in Magento 2
Let's walk through a simple implementation using Ethereum. You'll need:
- Magento 2 store (2.4.x recommended)
- Access to an Ethereum node (or test network)
- Basic PHP and Solidity knowledge
Step 1: Create a Smart Contract
Here's a simple Solidity contract to track product movements:
pragma solidity ^0.8.0;
contract ProductTracker {
struct Movement {
address handler;
uint256 timestamp;
string location;
string notes;
}
mapping(uint256 => Movement[]) public productHistory;
function addMovement(
uint256 productId,
string memory location,
string memory notes
) public {
productHistory[productId].push(
Movement(msg.sender, block.timestamp, location, notes)
);
}
function getMovementCount(uint256 productId) public view returns (uint) {
return productHistory[productId].length;
}
function getMovement(
uint256 productId,
uint256 index
) public view returns (address, uint256, string memory, string memory) {
Movement memory m = productHistory[productId][index];
return (m.handler, m.timestamp, m.location, m.notes);
}
}
Step 2: Connect Magento to the Blockchain
Create a custom module with this basic setup:
// app/code/Vendor/BlockchainTracker/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_BlockchainTracker">
<sequence>
<module name="Magento_Catalog"/>
</sequence>
</module>
</config>
Then create a helper to interact with Ethereum:
// app/code/Vendor/BlockchainTracker/Helper/Ethereum.php
<?php
namespace Vendor\BlockchainTracker\Helper;
use Web3\Web3;
use Web3\Contract;
class Ethereum
{
protected $web3;
protected $contract;
public function __construct()
{
$this->web3 = new Web3('http://localhost:8545');
$abi = '[YOUR_CONTRACT_ABI]';
$this->contract = new Contract($this->web3->provider, $abi);
$this->contract->at('YOUR_CONTRACT_ADDRESS');
}
public function recordMovement($productId, $location, $notes)
{
$this->contract->send('addMovement', $productId, $location, $notes);
}
public function getHistory($productId)
{
$history = [];
$this->contract->call('getMovementCount', $productId, function($err, $count) use (&$history, $productId) {
for ($i = 0; $i < $count; $i++) {
$this->contract->call('getMovement', $productId, $i, function($err, $result) use (&$history) {
$history[] = [
'handler' => $result[0],
'timestamp' => $result[1],
'location' => $result[2],
'notes' => $result[3]
];
});
}
});
return $history;
}
}
Step 3: Display Tracking Information
Add a block to your product page:
// app/code/Vendor/BlockchainTracker/view/frontend/layout/catalog_product_view.xml
<referenceContainer name="product.info.main">
<block class="Magento\Framework\View\Element\Template" name="product.blockchain.tracker" template="Vendor_BlockchainTracker::product/view/tracker.phtml"/>
</referenceContainer>
And the template:
<!-- app/code/Vendor/BlockchainTracker/view/frontend/templates/product/view/tracker.phtml -->
<?php
$helper = $this->helper('Vendor\BlockchainTracker\Helper\Ethereum');
$history = $helper->getHistory($block->getProduct()->getId());
?>
<div class="blockchain-tracker">
<h3>Product Journey</h3>
<div class="tracker-timeline">
<?php foreach ($history as $event): ?>
<div class="tracker-event">
<div class="event-time"><?= date('Y-m-d H:i', $event['timestamp']) ?></div>
<div class="event-location"><?= $event['location'] ?></div>
<div class="event-notes"><?= $event['notes'] ?></div>
</div>
<?php endforeach; ?>
</div>
</div>
Real-World Use Cases
Several industries are already benefiting from blockchain supply chain tracking:
- Luxury goods: Verify authenticity of high-end products
- Food industry: Track farm-to-table journey for freshness
- Pharmaceuticals: Prevent counterfeit medicines
- Electronics: Ensure conflict-free minerals
Challenges to Consider
While promising, blockchain integration isn't without hurdles: