How to Implement GraphQL in Magento 2 for Better API Performance
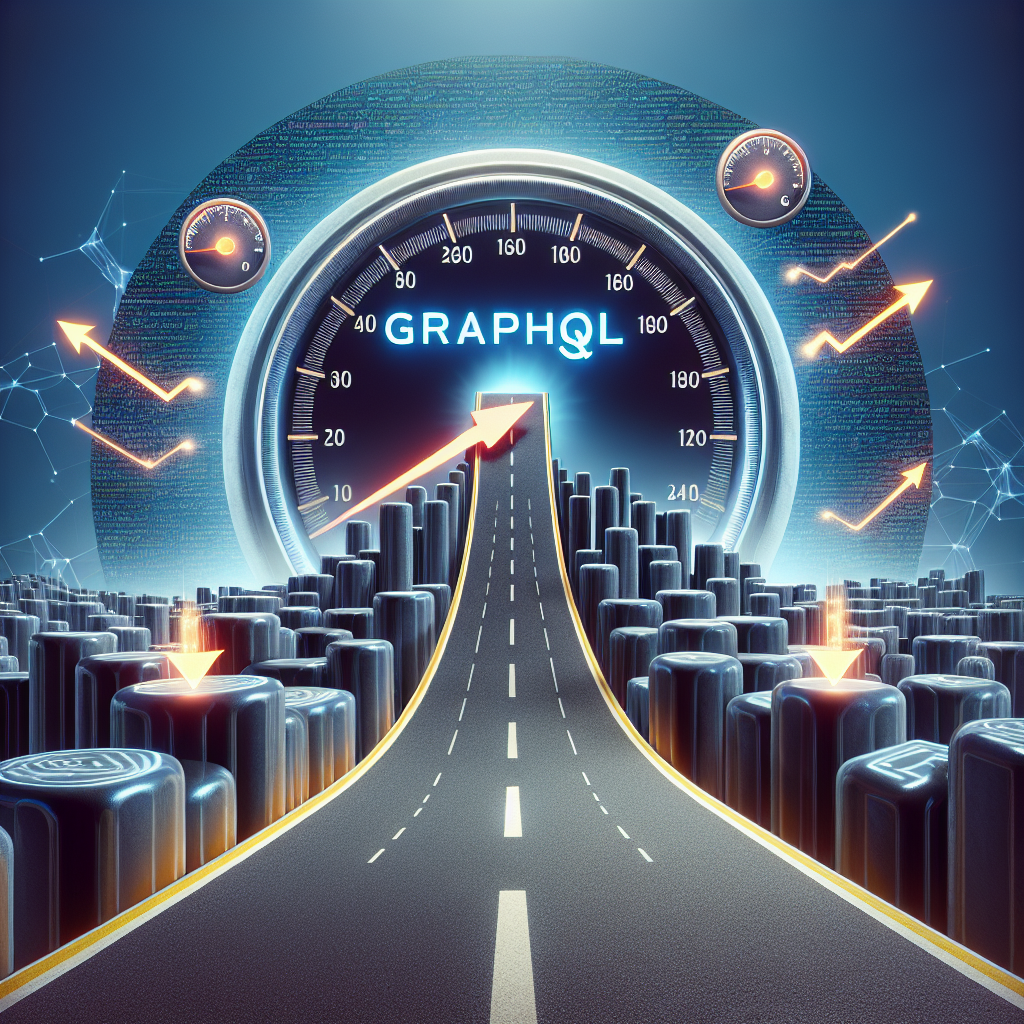
Why GraphQL is a Game-Changer for Magento 2 APIs
If you've worked with Magento 2's REST or SOAP APIs, you know they can be clunky. Over-fetching data, multiple round trips to the server, and rigid response structures are common pain points. GraphQL solves these issues by letting clients request exactly what they need in a single query.
Here's why Magento 2 store owners should care:
- Faster frontend performance - Mobile apps and PWA stores load only necessary data
- Reduced server load - Fewer API calls mean less strain on your hosting
- Future-proof architecture - GraphQL is becoming the standard for eCommerce APIs
Setting Up GraphQL in Magento 2
Magento 2.3+ includes native GraphQL support, but you'll need to enable and configure it properly. Here's how:
1. Verify Your Magento Version
First, check if your store supports GraphQL:
php bin/magento --version
You need at least Magento 2.3.0. If you're running an older version, consider upgrading or using third-party modules.
2. Enable GraphQL Endpoint
GraphQL is enabled by default in recent versions. The endpoint is typically at:
https://yourstore.com/graphql
You can test it with this simple query in Postman or GraphQL IDE:
{
storeConfig {
base_url
store_name
}
}
3. Configure CORS for GraphQL
For security, you'll need to set up CORS headers. Create or modify your .htaccess
file:
<IfModule mod_headers.c>
Header set Access-Control-Allow-Origin "*"
Header set Access-Control-Allow-Methods "POST, OPTIONS"
Header set Access-Control-Allow-Headers "Content-Type, Authorization"
</IfModule>
Writing Your First GraphQL Query
Let's start with a practical example - fetching product data. Compare this to traditional REST endpoints and you'll see the difference immediately.
Basic Product Query
{
products(filter: {sku: {eq: "24-MB01"}}) {
items {
id
name
sku
price {
regularPrice {
amount {
value
currency
}
}
}
}
}
}
This returns only the fields we need - no wasted data transfer.
Complex Query with Related Data
Here's where GraphQL shines. In one request, get a product with its categories, media gallery, and stock status:
{
products(filter: {category_id: {eq: "15"}}) {
items {
name
sku
categories {
name
url_path
}
media_gallery {
url
label
}
stock_status
}
}
}
Performance Optimization Tips
To get the most out of GraphQL in Magento 2:
1. Implement Data Caching
Add Redis caching for GraphQL responses:
# app/etc/env.php
'cache' => [
'graphql' => [
'id_prefix' => 'gql_',
'backend' => 'Magento\\Framework\\Cache\\Backend\\Redis',
'backend_options' => [
'server' => '127.0.0.1',
'port' => '6379',
'database' => '1'
]
]
]
2. Use Persisted Queries
Persisted queries reduce parsing overhead. First, register your query:
mutation {
createPersistedQuery(
query: "query GetProduct($sku: String!) {...}"
hash: "abc123"
) {
status
}
}
Then call it with just the hash:
{
"queryName": "GetProduct",
"variables": {"sku": "24-MB01"},
"extensions": {"persistedQuery": {"version":1,"sha256Hash":"abc123"}}
}
3. Batch Requests
Combine multiple queries into one HTTP request:
[
{"query": "query{products{items{sku}}}"},
{"query": "query{categories{items{name}}}"}
]
Extending GraphQL Schema
Need custom fields? Here's how to extend the schema:
1. Create Schema File
app/code/Vendor/Module/etc/schema.graphqls
:
type ProductInterface {
custom_field: String @resolver(class: "Vendor\\Module\\Model\\Resolver\\CustomField")
}
extend type Query {
customProducts(filter: ProductFilterInput): [ProductInterface]
@resolver(class: "Vendor\\Module\\Model\\Resolver\\CustomProducts")
}
2. Implement Resolver
app/code/Vendor/Module/Model/Resolver/CustomField.php
:
<?php
namespace Vendor\Module\Model\Resolver;
use Magento\Framework\GraphQl\Query\ResolverInterface;
class CustomField implements ResolverInterface
{
public function resolve($field, $context, $info, array $value = null, array $args = null)
{
return $value['sku'] . '_custom'; // Your logic here
}
}
Monitoring GraphQL Performance
Track your API performance with:
- New Relic - Monitor GraphQL transaction times
- Blackfire.io - Profile query execution
- Magento Profiler - Enable with
bin/magento deploy:mode:set developer
Common Pitfalls to Avoid
- N+1 Query Problem - Use data loaders to batch database requests
- Overly Complex Queries - Limit query depth with
graphql.query.max_depth
in config - No Rate Limiting - Implement API throttling to prevent abuse
When to Consider Third-Party Extensions
The native GraphQL implementation covers most needs, but extensions like these can help:
- GraphQL Query Cache - Advanced caching strategies
- GraphQL Logger - Detailed query logging
- GraphQL Security - Additional authentication methods
For most stores, the built-in functionality is sufficient after proper optimization.
Final Thoughts
Implementing GraphQL in Magento 2 can significantly improve your store's API performance, especially for:
- Progressive Web Apps (PWAs)
- Mobile applications
- Headless commerce implementations
- Third-party integrations
The initial setup is straightforward, but the real benefits come from proper optimization. Start with basic queries, monitor performance, and gradually implement more advanced techniques as needed.
Remember - GraphQL isn't just about technology, it's about delivering better experiences to your customers through faster, more efficient data loading.