How to Implement Advanced Search Functionality in Magento 2
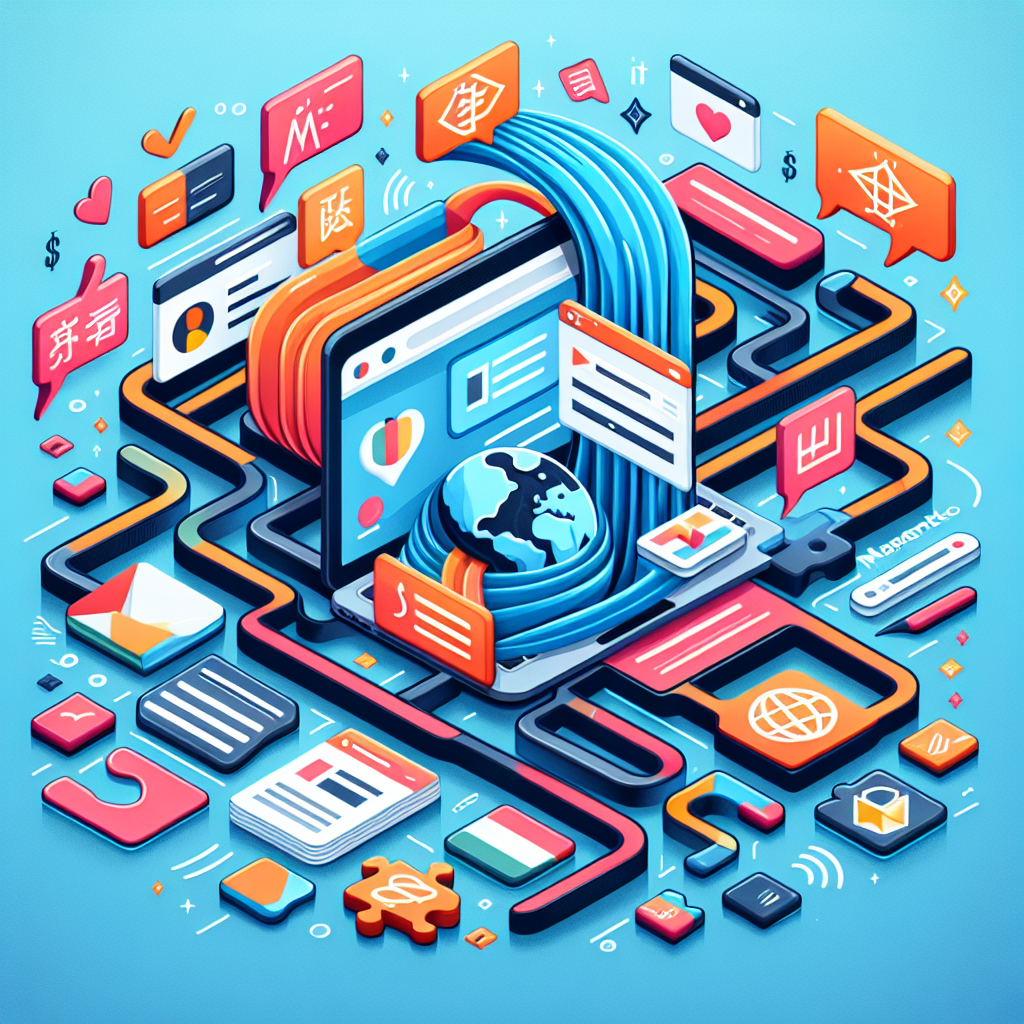
How to Implement Advanced Search Functionality in Magento 2
If you're running a Magento 2 store, you know how crucial it is to provide your customers with a seamless shopping experience. One of the key elements of this experience is the search functionality. A robust search feature can significantly enhance user satisfaction, leading to higher conversion rates. In this post, we'll walk you through the steps to implement advanced search functionality in Magento 2, complete with code examples and best practices.
Why Advanced Search Matters
Before diving into the technical details, let's briefly discuss why advanced search is essential. A basic search function might suffice for small stores with limited products, but as your inventory grows, customers need more sophisticated tools to find what they're looking for. Advanced search allows users to filter results by various attributes, such as price range, category, brand, and more. This not only improves the user experience but also increases the likelihood of a purchase.
Step 1: Enable Elasticsearch
Magento 2 supports Elasticsearch, a powerful search engine that provides fast and accurate search results. To enable Elasticsearch, follow these steps:
- Log in to your Magento 2 admin panel.
- Navigate to Stores > Configuration > Catalog > Catalog > Catalog Search.
- Set Search Engine to Elasticsearch.
- Fill in the Elasticsearch server details, such as Host, Port, and Index Prefix.
- Save the configuration.
Here's a snippet of what the configuration might look like:
<?php
// Example of setting Elasticsearch in Magento 2
$config->saveConfig('catalog/search/engine', 'elasticsearch7', 'default', 0);
$config->saveConfig('catalog/search/elasticsearch7_server_hostname', 'localhost', 'default', 0);
$config->saveConfig('catalog/search/elasticsearch7_server_port', '9200', 'default', 0);
$config->saveConfig('catalog/search/elasticsearch7_index_prefix', 'magento2', 'default', 0);
?>
Step 2: Customize Search Attributes
Magento 2 allows you to specify which product attributes should be searchable. This is particularly useful if you want to enable filtering by specific attributes like color, size, or material. To customize search attributes:
- Go to Stores > Attributes > Product.
- Select the attribute you want to make searchable.
- Under the Storefront Properties tab, set Use in Search to Yes.
- Save the attribute.
Here's an example of how to programmatically set an attribute as searchable:
<?php
// Example of setting an attribute as searchable
$attribute = $this->attributeRepository->get('color');
$attribute->setIsSearchable(1);
$this->attributeRepository->save($attribute);
?>
Step 3: Implement Layered Navigation
Layered navigation is a powerful feature that allows customers to filter search results by various attributes. To implement layered navigation:
- Go to Stores > Attributes > Product.
- Select the attribute you want to use for filtering.
- Under the Storefront Properties tab, set Use in Layered Navigation to Filterable (with results).
- Save the attribute.
Here's a code snippet to enable layered navigation for an attribute:
<?php
// Example of enabling layered navigation for an attribute
$attribute = $this->attributeRepository->get('size');
$attribute->setIsFilterable(1);
$this->attributeRepository->save($attribute);
?>
Step 4: Customize the Search Results Page
To provide a better user experience, you might want to customize the search results page. This can include changing the layout, adding custom filters, or displaying additional product information. To customize the search results page:
- Create a new XML layout file in your theme: app/design/frontend/[Vendor]/[Theme]/Magento_Catalog/layout/catalogsearch_result_index.xml.
- Add your customizations to the XML file.
Here's an example of a custom XML layout for the search results page:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<block class="Magento\CatalogSearch\Block\Result" name="search.result" template="Magento_CatalogSearch::result.phtml">
<block class="Magento\Catalog\Block\Product\ListProduct" name="search_result_list" template="Magento_Catalog::product/list.phtml">
<container name="category.product.list.additional" as="additional" />
<block class="Magento\Framework\View\Element\RendererList" name="category.product.type.details.renderers" as="details.renderers">
<block class="Magento\Framework\View\Element\Template" name="category.product.type.details.renderers.default" as="default"/>
</block>
</block>
</block>
</referenceContainer>
</body>
</page>
Step 5: Optimize Search Performance
Finally, it's essential to optimize the performance of your search functionality. Here are a few tips:
- Use Elasticsearch: As mentioned earlier, Elasticsearch is faster and more efficient than MySQL for search queries.
- Index Management: Regularly reindex your data to ensure that search results are up-to-date.
- Cache Management: Enable caching for search results to reduce server load and improve response times.
To reindex your data, run the following command:
php bin/magento indexer:reindex catalogsearch_fulltext
Conclusion
Implementing advanced search functionality in Magento 2 can significantly enhance the user experience on your store. By enabling Elasticsearch, customizing search attributes, implementing layered navigation, and optimizing performance, you can provide your customers with a powerful and efficient search tool. Follow the steps and code examples provided in this post to get started, and don't hesitate to explore further customizations to meet your specific needs.
If you're looking for more advanced features or need professional assistance, consider checking out the extensions and hosting services available at magefine.com. Happy coding!