Demystifying EAV Structure in Magento 2: A Comprehensive Guide
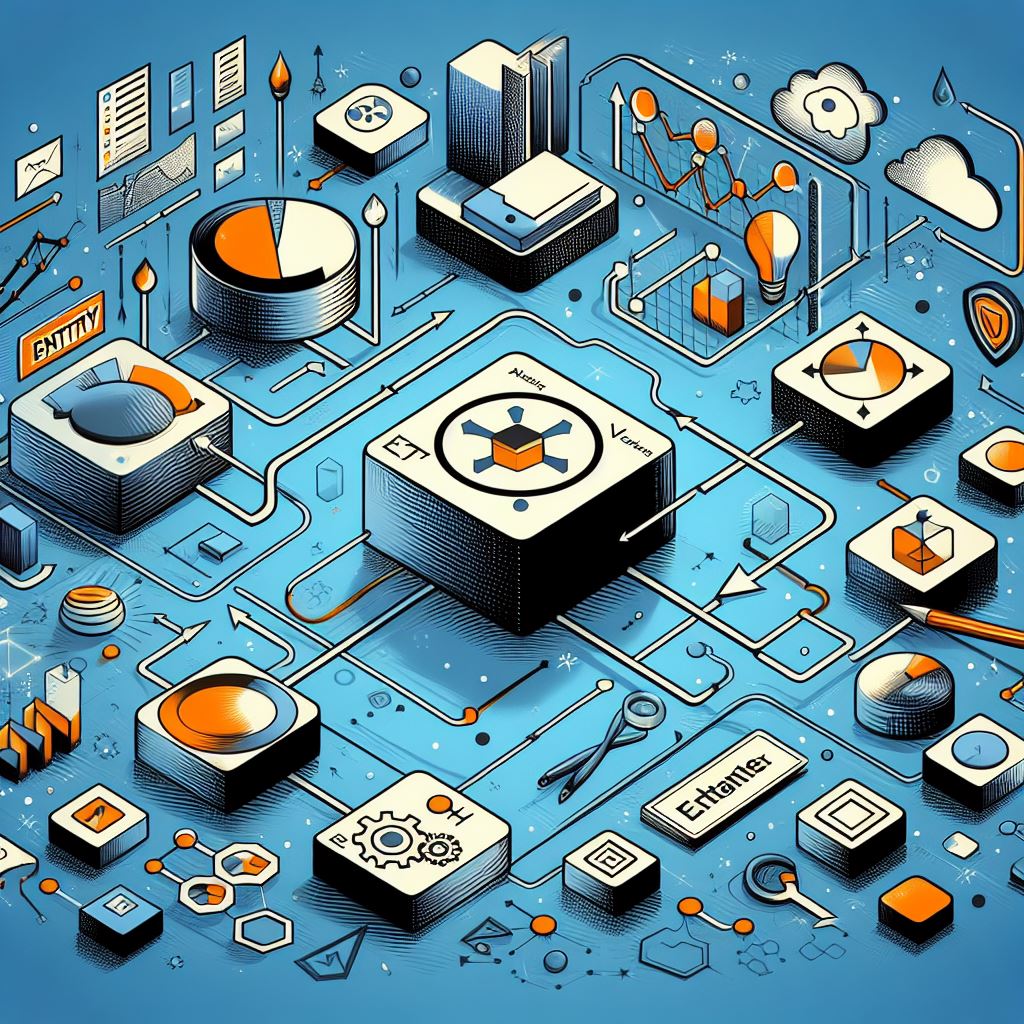
As a seasoned e-commerce developer with expertise in Magento 2, you are no stranger to the complexity of managing and organizing data for your online store. One of the key architectural concepts that sets Magento apart is its Entity-Attribute-Value (EAV) structure. In this comprehensive guide, we will explore what the EAV structure is, why it's crucial for Magento 2, and how it functions. We'll also provide code snippets to illustrate its implementation.
What is the EAV Structure?
The Entity-Attribute-Value (EAV) structure is a flexible and dynamic way to manage and store data in a relational database. In the context of Magento 2, it is the underlying architecture for handling complex and highly customizable data such as product attributes, customer information, and more.
Entities
In the EAV structure, entities represent the core data elements you want to store. In Magento 2, common entities include products, customers, categories, and orders. Each entity is uniquely identified by an entity type.
Attributes
Attributes define the properties or characteristics of an entity. In the EAV model, attributes are categorized as either static or dynamic. Static attributes, such as SKU or price, are shared among all entities of the same type. Dynamic attributes, on the other hand, can vary from one entity to another, making the EAV structure ideal for handling diverse product attributes.
Values
Values, as the name suggests, store the actual data for each attribute associated with an entity. The EAV structure allows multiple values for a single attribute, accommodating various data types and values for different entities.
Why EAV Structure in Magento 2?
The EAV structure is particularly advantageous for e-commerce platforms like Magento 2 for several reasons:
1. Flexibility
E-commerce websites often require a wide range of customizable attributes for products and customer profiles. The EAV structure enables this flexibility by allowing you to define and manage countless attributes without altering the database schema.
2. Scalability
As your online store grows, so does the volume and complexity of data. The EAV structure scales effortlessly, ensuring efficient data storage and retrieval even with a vast product catalog or extensive customer data.
3. Localization
With global e-commerce operations, localization is vital. EAV supports localization by allowing attribute values to vary based on store views, languages, or regions.
How EAV Structure Functions in Magento 2
Let's explore how the EAV structure operates in Magento 2 using PHP code examples.
Retrieving Product Attributes
To retrieve product attributes using the EAV structure, you can use the following code snippet:
phpCopy code
use Magento\Catalog\Api\ProductAttributeRepositoryInterface;
class AttributeExample
{
protected $attributeRepository;
public function __construct(ProductAttributeRepositoryInterface $attributeRepository)
{
$this->attributeRepository = $attributeRepository;
}
public function getAttributeLabel($attributeCode)
{
$attribute = $this->attributeRepository->get($attributeCode);
return $attribute->getDefaultFrontendLabel();
}
}
In this example, we inject the ProductAttributeRepositoryInterface to retrieve product attributes by their code.
Managing Customer Attributes
For managing customer attributes, you can use similar principles. Here's an example:
use Magento\Customer\Api\AddressMetadataInterface;
class CustomerAttributeExample
{
protected $addressMetadata;
public function __construct(AddressMetadataInterface $addressMetadata)
{
$this->addressMetadata = $addressMetadata;
}
public function getAttributeLabel($attributeCode)
{
$attributeMetadata = $this->addressMetadata->getAttributeMetadata($attributeCode);
return $attributeMetadata->getLabel();
}
}
In this case, we use the AddressMetadataInterface to manage customer attributes.
Conclusion
The Entity-Attribute-Value (EAV) structure is the backbone of Magento 2's dynamic and customizable data management. It empowers e-commerce developers like you to handle complex product attributes, customer information, and more with flexibility and scalability.
In this guide, we've delved into the significance of the EAV structure in Magento 2, explained its core components, and provided code examples to illustrate its implementation. Armed with this knowledge, you can optimize your e-commerce website's data management, ensuring a seamless shopping experience for your customers.
Happy coding!