Exploring Class Dependency Injection in Magento 2
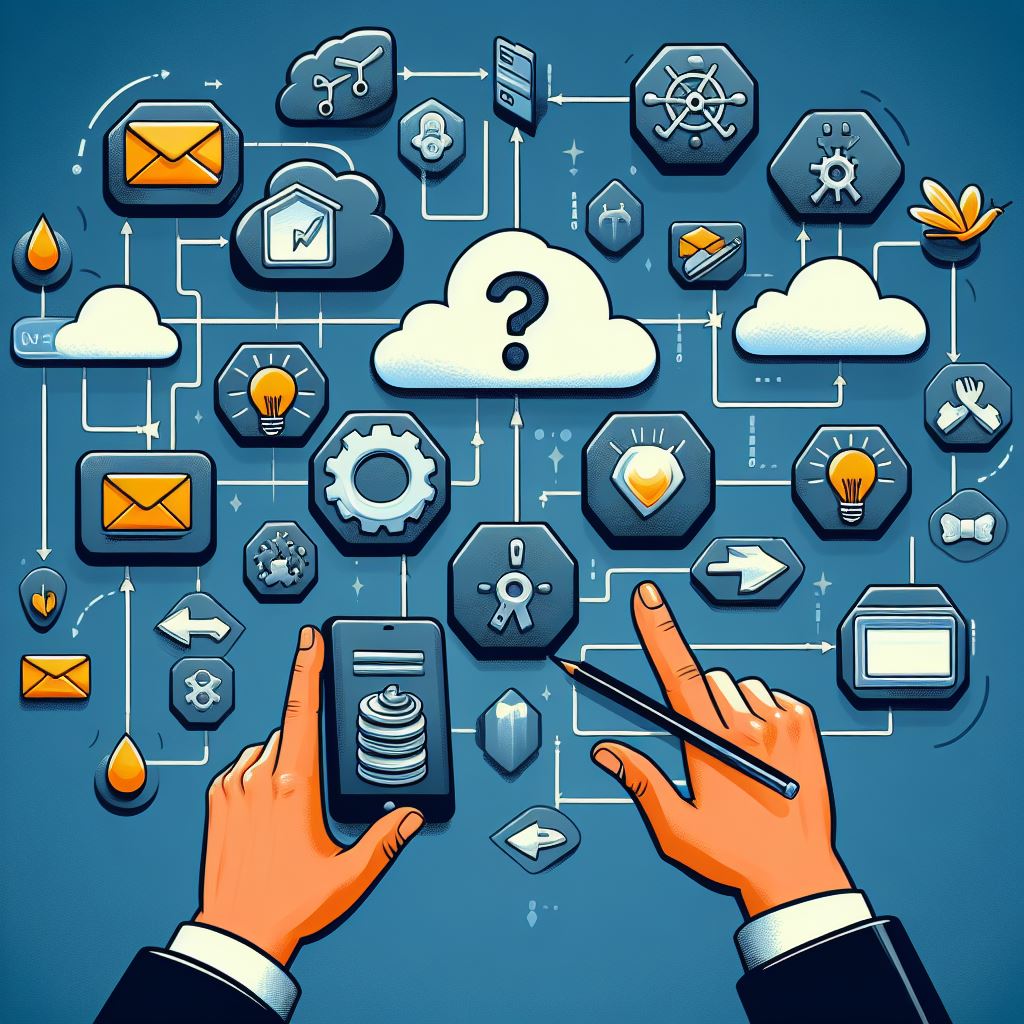
As an experienced e-commerce developer proficient in Magento 2, you understand the importance of clean, maintainable, and efficient code. One of the key design patterns that contribute to achieving these goals is Class Dependency Injection. In this comprehensive guide, we will unravel the concept of Class Dependency Injection in Magento 2, shedding light on what it is and how it operates. Additionally, we will provide code snippets to illustrate its implementation.
What is Class Dependency Injection?
Class Dependency Injection (CDI) is a design pattern utilized extensively in Magento 2 and modern PHP development. At its core, CDI is a technique that allows you to inject dependent objects or services into a class through its constructor. This approach promotes code modularity, reusability, and testability, while also reducing the tight coupling between components.
In Magento 2, Class Dependency Injection plays a pivotal role in structuring and managing object instances, making it an essential concept for extension development and website optimization.
The Significance of Class Dependency Injection in Magento 2
- Code Organization
One of the primary advantages of CDI is improved code organization. By injecting dependencies directly into a class, you clearly define its requirements and reduce the need for complex initialization procedures within the class. This leads to cleaner, more readable code.
- Reusability
CDI facilitates code reusability by allowing you to easily swap out dependencies. You can create interchangeable components that can be reused across different projects or tailored to specific client needs without modifying the core class.
- Testability
In the realm of e-commerce development, testing is paramount. CDI simplifies the process of unit testing by enabling the use of mock objects or stubs to replace real dependencies during testing. This ensures that individual components of your extension or application can be tested in isolation for reliability.
Implementing Class Dependency Injection in Magento 2
Let's dive into the practical implementation of Class Dependency Injection in Magento 2 using PHP code examples. We will primarily focus on constructor injection.
Constructor Injection
In Magento 2, the most common form of CDI is constructor injection. It involves passing dependencies through a class's constructor. Here's an example:
use Magento\Catalog\Api\ProductRepositoryInterface;
class MyExtension
{
protected $productRepository;
public function __construct(ProductRepositoryInterface $productRepository)
{
$this->productRepository = $productRepository;
}
// ...
}
In this example, the ProductRepositoryInterface is injected into the MyExtension class via its constructor. This ensures that the required dependency is available for use within the class.
Conclusion
Class Dependency Injection is a fundamental concept in Magento 2 development, offering numerous benefits such as improved code organization, reusability, and testability. Understanding how to implement CDI in your Magento 2 extensions and websites is essential for building robust, scalable, and maintainable e-commerce solutions.
In this guide, we've explored the significance of Class Dependency Injection in Magento 2 and provided code examples to demonstrate its practical implementation. Armed with this knowledge, you can elevate your e-commerce development expertise and create exceptional online shopping experiences for your clients and customers.
Happy coding!