Magento 2 and WebSockets: Real-Time Updates for Cart/Inventory Changes
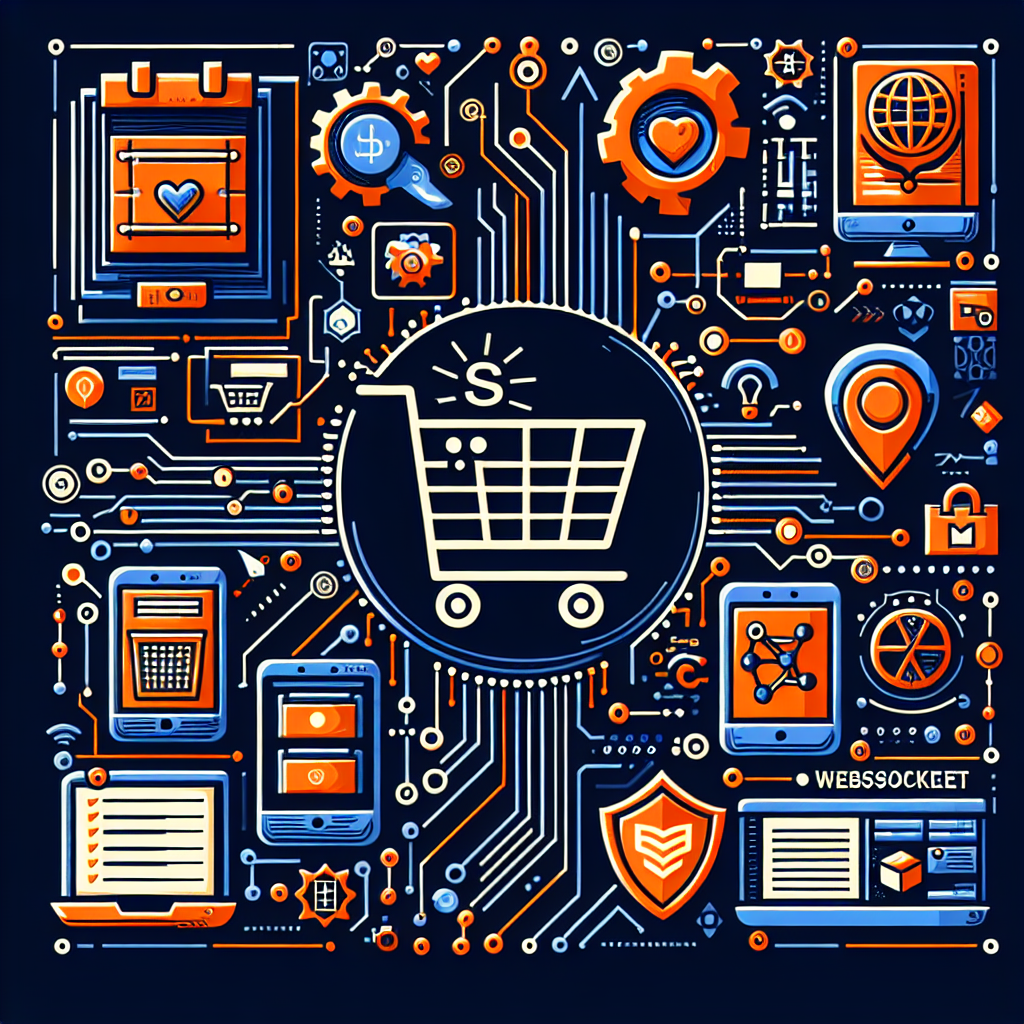
Magento 2 and WebSockets: Real-Time Updates for Cart/Inventory Changes
Ever wondered how some e-commerce stores update cart totals or inventory levels instantly without refreshing the page? That’s WebSockets in action! If you’re running a Magento 2 store and want to give your customers a seamless, real-time experience, WebSockets are your best friend.
In this post, we’ll break down how WebSockets work, why they’re perfect for Magento 2, and how to implement them for cart and inventory updates. No jargon—just practical steps to make your store feel like magic.
What Are WebSockets?
WebSockets are a communication protocol that allows real-time, two-way interaction between a client (like a browser) and a server. Unlike traditional HTTP requests, which require constant polling (asking the server for updates), WebSockets keep a persistent connection open. This means instant updates without delays.
For Magento 2, this is a game-changer for:
- Cart updates – Show changes immediately when a customer adds/removes items.
- Inventory sync – Prevent overselling by updating stock levels in real time.
- Price adjustments – Reflect dynamic pricing changes instantly.
Why Use WebSockets Instead of AJAX?
AJAX is great, but it’s not real-time. It requires the browser to repeatedly ask the server: "Hey, any updates?" This creates unnecessary overhead and delays. WebSockets, on the other hand, let the server push updates the moment they happen.
Here’s a quick comparison:
Feature | AJAX | WebSockets |
---|---|---|
Connection | Short-lived (per request) | Persistent |
Latency | High (depends on polling frequency) | Low (instant updates) |
Server Load | Higher (constant requests) | Lower (only updates when needed) |
Setting Up WebSockets in Magento 2
To get started, you’ll need:
- A WebSocket server (we’ll use Ratchet, a PHP WebSocket library).
- A Magento 2 module to handle WebSocket events.
- JavaScript to connect the frontend.
Step 1: Install Ratchet
First, install Ratchet via Composer:
composer require cboden/ratchet
Step 2: Create a WebSocket Server
Create a PHP script (e.g., websocket_server.php
) to run the WebSocket server:
<?php
use Ratchet\Server\IoServer;
use Ratchet\Http\HttpServer;
use Ratchet\WebSocket\WsServer;
use YourNamespace\WebSocketHandler;
require 'vendor/autoload.php';
$server = IoServer::factory(
new HttpServer(
new WsServer(
new WebSocketHandler()
)
),
8080 // Port number
);
$server->run();
Step 3: Create a WebSocket Handler
Next, define a handler class (WebSocketHandler.php
) to manage connections and messages:
<?php
namespace YourNamespace;
use Ratchet\MessageComponentInterface;
use Ratchet\ConnectionInterface;
class WebSocketHandler implements MessageComponentInterface {
protected $clients;
public function __construct() {
$this->clients = new \SplObjectStorage;
}
public function onOpen(ConnectionInterface $conn) {
$this->clients->attach($conn);
echo "New connection! ({$conn->resourceId})\n";
}
public function onMessage(ConnectionInterface $from, $msg) {
// Handle incoming messages (e.g., cart updates)
foreach ($this->clients as $client) {
if ($client !== $from) {
$client->send($msg);
}
}
}
public function onClose(ConnectionInterface $conn) {
$this->clients->detach($conn);
echo "Connection {$conn->resourceId} disconnected\n";
}
public function onError(ConnectionInterface $conn, \Exception $e) {
echo "Error: {$e->getMessage()}\n";
$conn->close();
}
}
Step 4: Integrate with Magento 2
Now, let’s create a Magento 2 module to trigger WebSocket events. Here’s a basic observer for cart updates:
<?php
namespace YourNamespace\WebSocket\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
use Ratchet\Client\Connector;
use React\EventLoop\Factory;
class CartUpdateObserver implements ObserverInterface {
public function execute(Observer $observer) {
$loop = Factory::create();
$connector = new Connector($loop);
$connector('ws://localhost:8080')
->then(function($conn) {
$conn->send(json_encode([
'event' => 'cart_updated',
'data' => 'Cart has been modified'
]));
$conn->close();
}, function ($e) {
echo "Could not connect: {$e->getMessage()}\n";
});
$loop->run();
}
}
Step 5: Frontend JavaScript
Finally, add JavaScript to your Magento 2 frontend to listen for WebSocket updates:
<script>
const socket = new WebSocket('ws://localhost:8080');
socket.onmessage = function(event) {
const data = JSON.parse(event.data);
if (data.event === 'cart_updated') {
// Refresh mini-cart or show notification
require(['Magento_Customer/js/customer-data'], function(customerData) {
customerData.reload(['cart'], true);
});
}
};
</script>
Testing & Debugging
To test:
- Run the WebSocket server:
php websocket_server.php
- Add an item to the cart in your Magento 2 store.
- Check the browser console for real-time updates.
Common issues:
- Connection refused? Ensure the server is running and the port is open.
- No updates? Verify your observer is triggering correctly.
Performance Considerations
WebSockets are efficient, but keep these in mind:
- Scalability: Use a load balancer if handling many connections.
- Fallback: Some networks block WebSockets—have an AJAX fallback.
- Security: Always use
wss://
(secure WebSockets) in production.
Final Thoughts
WebSockets take your Magento 2 store from "good" to "wow" by making interactions instant. Whether it’s cart updates, stock changes, or live notifications, real-time features keep customers engaged and reduce frustration.
Ready to implement this? Check out Magefine’s hosting solutions for optimized Magento 2 performance!