Magento 2 and Third-Party APIs: Integration Best Practices
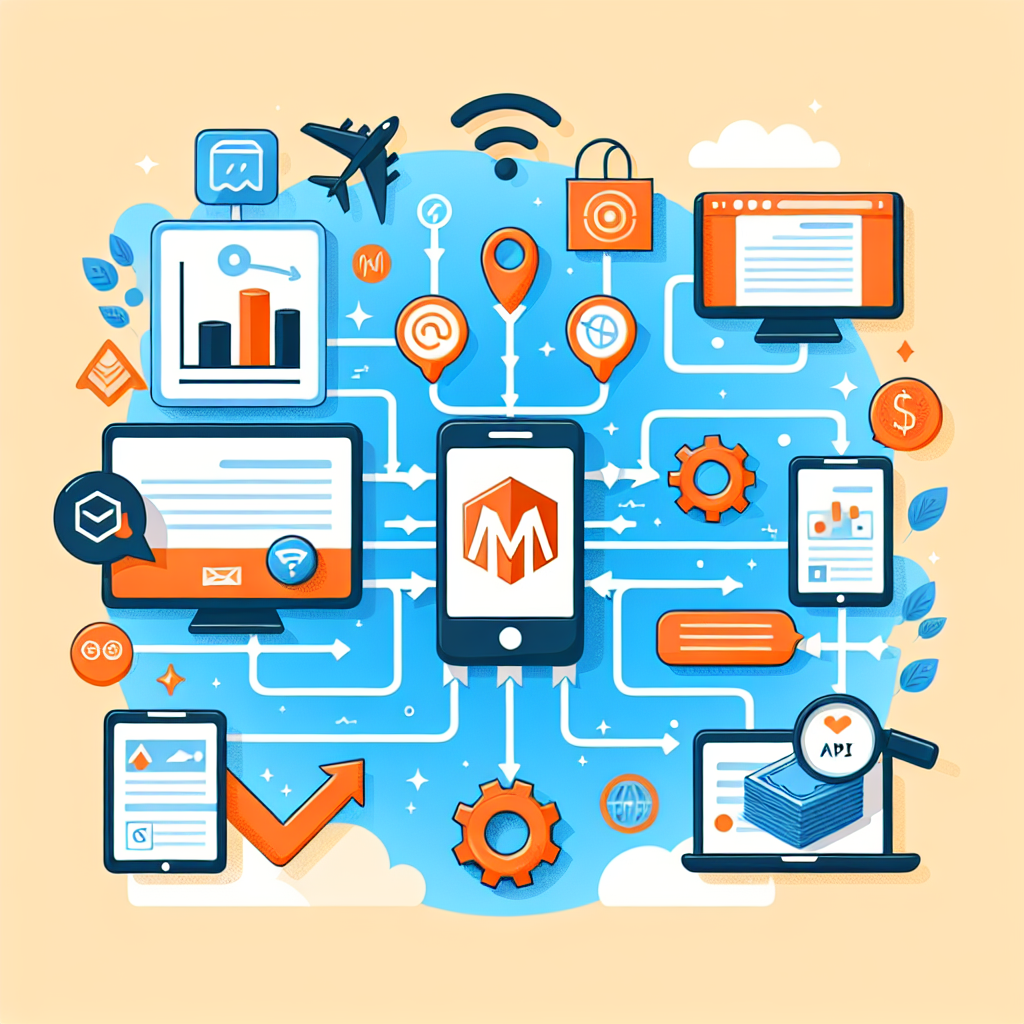
Magento 2 and Third-Party APIs: Integration Best Practices
Integrating third-party APIs with Magento 2 can supercharge your store’s functionality—whether it’s syncing inventory, processing payments, or fetching shipping rates. But let’s be honest: API integrations can be tricky if you don’t follow the right approach.
In this guide, we’ll walk through the best practices for integrating external APIs with Magento 2, ensuring smooth performance, reliability, and maintainability. We’ll also dive into some code examples to make things crystal clear.
Why API Integrations Matter in Magento 2
APIs (Application Programming Interfaces) act as bridges between Magento 2 and external services. They allow your store to:
- Fetch real-time shipping rates from carriers like FedEx or UPS
- Process payments via Stripe, PayPal, or other gateways
- Sync inventory with ERP systems
- Pull product reviews from third-party platforms
But if not handled properly, API integrations can slow down your store, cause errors, or even break during peak traffic. That’s why following best practices is crucial.
Best Practices for Magento 2 API Integrations
1. Use Magento’s Built-in HTTP Clients
Magento 2 provides built-in HTTP clients (Magento\Framework\HTTP\ClientInterface
) to handle API requests. Avoid using raw cURL or file_get_contents()—these can lead to security and performance issues.
<?php
use Magento\Framework\HTTP\ClientInterface;
class MyApiService
{
protected $httpClient;
public function __construct(ClientInterface $httpClient)
{
$this->httpClient = $httpClient;
}
public function fetchDataFromApi($endpoint, $params = [])
{
$this->httpClient->get($endpoint . '?' . http_build_query($params));
return $this->httpClient->getBody();
}
}
2. Implement Proper Error Handling
APIs can fail—timeouts, rate limits, invalid responses. Always handle errors gracefully to avoid breaking the user experience.
public function fetchDataFromApi($endpoint)
{
try {
$this->httpClient->get($endpoint);
if ($this->httpClient->getStatus() !== 200) {
throw new \Exception("API request failed: " . $this->httpClient->getBody());
}
return json_decode($this->httpClient->getBody(), true);
} catch (\Exception $e) {
$this->logger->error($e->getMessage());
return [];
}
}
3. Cache API Responses When Possible
Frequent API calls can slow down your store. Use Magento’s cache system to store responses temporarily.
use Magento\Framework\App\CacheInterface;
public function getCachedApiData($endpoint, $cacheKey, $ttl = 3600)
{
$cachedData = $this->cache->load($cacheKey);
if ($cachedData) {
return json_decode($cachedData, true);
}
$apiData = $this->fetchDataFromApi($endpoint);
$this->cache->save(json_encode($apiData), $cacheKey, [], $ttl);
return $apiData;
}
4. Use Queues for Heavy API Operations
For tasks like bulk order updates or inventory syncs, use Magento’s message queues to avoid blocking the frontend.
use Magento\Framework\MessageQueue\PublisherInterface;
public function scheduleApiSync($data)
{
$this->publisher->publish('my_api_sync_queue', json_encode($data));
}
5. Secure Your API Credentials
Never hardcode API keys in your modules. Store them in app/etc/env.php
or use Magento’s encrypted configuration.
use Magento\Framework\Encryption\EncryptorInterface;
public function __construct(EncryptorInterface $encryptor)
{
$this->encryptor = $encryptor;
}
public function getDecryptedApiKey()
{
$encryptedKey = $this->scopeConfig->getValue('my_module/api/key');
return $this->encryptor->decrypt($encryptedKey);
}
6. Test API Integrations Thoroughly
Write unit and integration tests for your API calls. Magento’s testing framework supports mocking API responses.
public function testApiCall()
{
$mockResponse = ['status' => 'success'];
$this->httpClientMock->method('getBody')->willReturn(json_encode($mockResponse));
$result = $this->apiService->fetchDataFromApi('https://api.example.com');
$this->assertEquals($mockResponse, $result);
}
Common API Integration Scenarios
1. Payment Gateway Integration
When integrating payment processors like Stripe or PayPal:
- Use official SDKs when available
- Implement webhook handling for asynchronous events
- Always validate responses before processing orders
2. Shipping Carrier Integration
For real-time shipping rates:
- Cache rates to avoid excessive API calls
- Implement fallback rates in case of API failure
- Validate address data before making requests
3. ERP/Inventory Sync
For inventory management systems:
- Use batch processing for large updates
- Implement delta syncs to only update changed products
- Queue failed syncs for retry
Monitoring and Maintenance
Once your API integration is live:
- Log all API requests and responses (but redact sensitive data)
- Set up alerts for frequent failures
- Regularly review API usage against rate limits
- Update API versions as providers deprecate old endpoints
Final Thoughts
API integrations can transform your Magento 2 store’s capabilities, but they require careful implementation. By following these best practices—using Magento’s built-in tools, proper error handling, caching, and security measures—you’ll create robust integrations that scale with your business.
Need help with your Magento 2 API integration? Check out our Magento extensions and hosting solutions designed to make integrations seamless.