Magento 2 and Serverless Architecture: Is It a Good Fit?
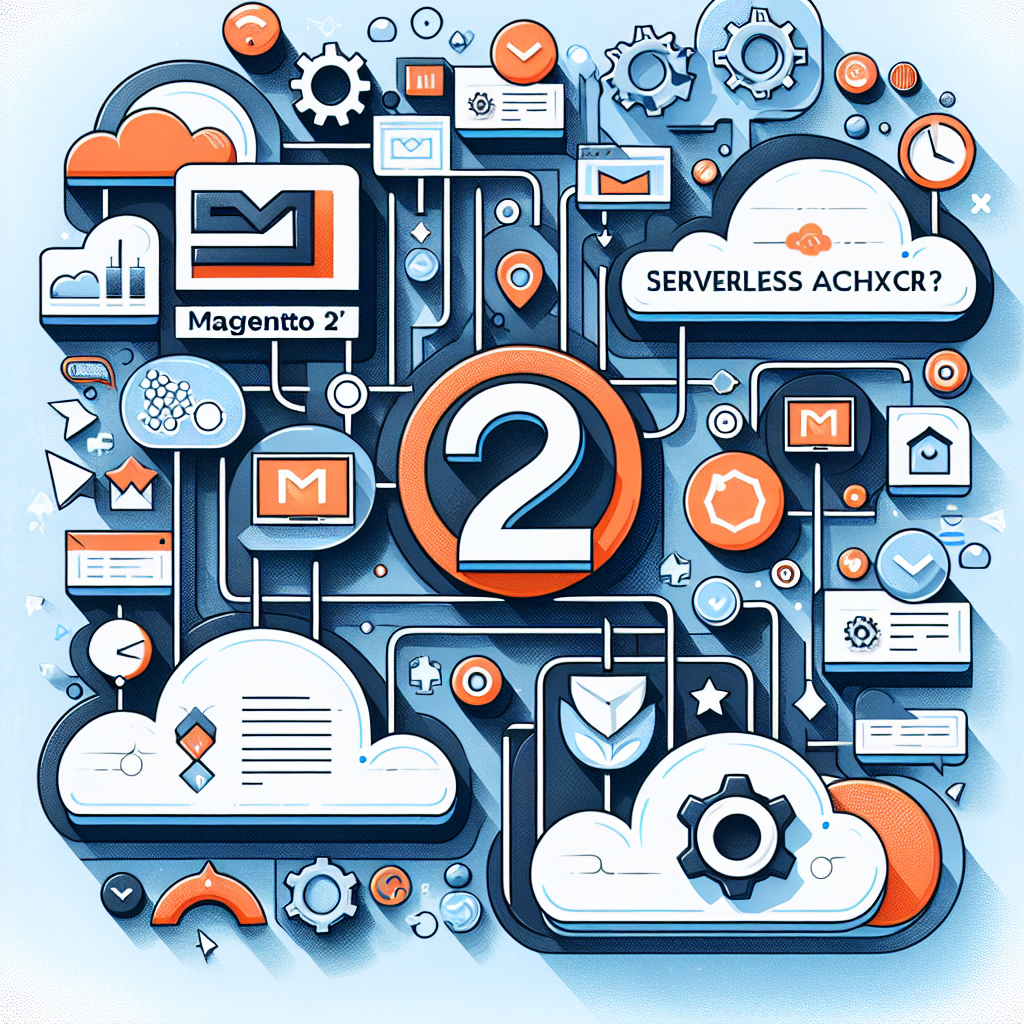
What is Serverless Architecture?
Before diving into whether Magento 2 and serverless architecture are a good match, let’s break down what serverless actually means. Despite its name, serverless doesn’t mean there are no servers involved—it just means you don’t have to manage them yourself. Instead, cloud providers like AWS Lambda, Google Cloud Functions, or Azure Functions handle the infrastructure, scaling, and maintenance for you.
Serverless computing allows you to run code in response to events (like an HTTP request, database change, or file upload) without provisioning or managing servers. You only pay for the compute time you consume, making it cost-effective for certain workloads.
Why Consider Serverless for Magento 2?
Magento 2 is a powerful but resource-intensive eCommerce platform. Traditional hosting setups require dedicated servers, load balancers, and constant scaling adjustments—especially during traffic spikes like Black Friday. Serverless architecture offers some compelling benefits:
- Automatic Scaling: No need to manually adjust server capacity—serverless functions scale instantly.
- Reduced Costs: Pay only for the compute time used, rather than maintaining idle servers.
- Simplified Maintenance: No server patching, updates, or security hardening required.
- Faster Development: Focus on writing business logic instead of managing infrastructure.
But is it a good fit for Magento 2? Let’s explore.
Where Serverless Fits in Magento 2
Magento 2 is a monolithic PHP application, meaning it’s not natively designed for serverless execution. However, certain parts of your Magento store can benefit from serverless architecture:
1. Offloading Heavy Backend Tasks
Tasks like order processing, inventory sync, or generating reports can be moved to serverless functions. For example, instead of running a cron job on your Magento server, you can trigger a Lambda function when an order is placed.
Example: AWS Lambda for Order Processing
const AWS = require('aws-sdk');
const magentoApi = require('magento2-rest-client').default;
exports.handler = async (event) => {
const orderData = JSON.parse(event.Records[0].body);
const client = magentoApi({
url: 'https://your-magento-store.com',
consumerKey: 'your_consumer_key',
consumerSecret: 'your_consumer_secret',
accessToken: 'your_access_token',
accessTokenSecret: 'your_access_token_secret'
});
try {
await client.post('/V1/orders/process', orderData);
return { status: 'Order processed successfully' };
} catch (error) {
console.error('Error processing order:', error);
throw error;
}
};
2. Handling API Integrations
Third-party integrations (payment gateways, shipping providers, CRM systems) can be managed via serverless functions, reducing load on your main Magento instance.
3. Image Processing & CDN Optimization
Instead of using Magento’s built-in image resizing, you can offload this to a serverless function triggered when new product images are uploaded.
4. Real-time Notifications
Push notifications, SMS alerts, or email campaigns can be handled serverlessly, improving responsiveness.
Where Serverless Doesn’t Fit
While serverless has advantages, it’s not a silver bullet for Magento 2. Here’s where it falls short:
- Core Magento Execution: Magento’s PHP-based architecture isn’t optimized for serverless runtimes like AWS Lambda.
- Database Dependencies: Serverless functions have cold starts, making them unsuitable for high-frequency database queries.
- Session Management: Magento relies on PHP sessions, which don’t play well with stateless serverless functions.
Hybrid Approach: Best of Both Worlds
The most practical solution is a hybrid setup:
- Host Magento traditionally (on a cloud VM, Kubernetes, or managed hosting).
- Offload specific tasks to serverless functions.
Example: Serverless Checkout Flow
Instead of handling the entire checkout process in Magento, you can:
- Use Magento for product browsing and cart management.
- Trigger a serverless function when the user proceeds to checkout.
- Process payment and inventory updates serverlessly.
- Return the result to Magento for order confirmation.
Step-by-Step: Integrating AWS Lambda with Magento 2
Let’s walk through a real-world example of using AWS Lambda to process abandoned cart emails.
Step 1: Set Up an SQS Queue
First, create an Amazon SQS queue to handle abandoned cart events:
aws sqs create-queue --queue-name magento-abandoned-carts
Step 2: Configure Magento to Send Events
In Magento, create an observer that sends cart data to SQS when a cart is abandoned:
<?php
use Aws\Sqs\SqsClient;
class AbandonedCartObserver implements \Magento\Framework\Event\ObserverInterface
{
public function execute(\Magento\Framework\Event\Observer $observer)
{
$cart = $observer->getEvent()->getCart();
$client = new SqsClient([
'region' => 'us-east-1',
'version' => 'latest'
]);
$client->sendMessage([
'QueueUrl' => 'https://sqs.us-east-1.amazonaws.com/your-queue-url',
'MessageBody' => json_encode([
'cart_id' => $cart->getId(),
'customer_id' => $cart->getCustomerId(),
'items' => $cart->getAllVisibleItems()
])
]);
}
}
Step 3: Create the Lambda Function
Write a Lambda function (Node.js) to process these events and send emails:
const AWS = require('aws-sdk');
const ses = new AWS.SES();
exports.handler = async (event) => {
for (const record of event.Records) {
const cart = JSON.parse(record.body);
const emailParams = {
Destination: { ToAddresses: [cart.customer_email] },
Message: {
Body: { Text: { Data: `You left items in your cart! View them here: https://yourstore.com/cart` } },
Subject: { Data: 'Complete Your Purchase' }
},
Source: 'noreply@yourstore.com'
};
await ses.sendEmail(emailParams).promise();
}
};
Step 4: Connect SQS to Lambda
Finally, configure the SQS queue as a trigger for your Lambda function in the AWS Console.
Performance Considerations
While serverless can improve scalability, be aware of:
- Cold Starts: The first invocation of a Lambda function may take longer (up to a few seconds).
- Execution Limits: AWS Lambda has a 15-minute maximum runtime per invocation.
- Concurrency Limits: By default, AWS allows 1,000 concurrent Lambda executions per account.
Cost Analysis
Serverless can be cost-effective for sporadic workloads but may become expensive for high-traffic stores. Here’s a rough comparison:
Scenario | Traditional Hosting | Serverless |
---|---|---|
Low Traffic (100 orders/day) | $50/month (fixed) | $5-$10/month |
High Traffic (10,000 orders/day) | $300/month | $200-$400/month |
Final Verdict: Is Serverless Right for Your Magento Store?
Yes, if:
- You want to offload specific tasks (like image processing or notifications).
- Your store has unpredictable traffic spikes.
- You’re comfortable with a hybrid architecture.
No, if:
- You expect to run Magento’s core entirely serverlessly.
- Your store requires persistent PHP sessions.
- You need ultra-low latency for all operations.
For most Magento 2 stores, a balanced approach—using serverless for background tasks while keeping the core on traditional hosting—offers the best mix of performance, scalability, and cost-efficiency.
Ready to experiment? Start by moving one non-critical function (like abandoned cart emails) to serverless and measure the impact!