Magento 2 Security: Protecting Against SQL Injection and XSS Attacks
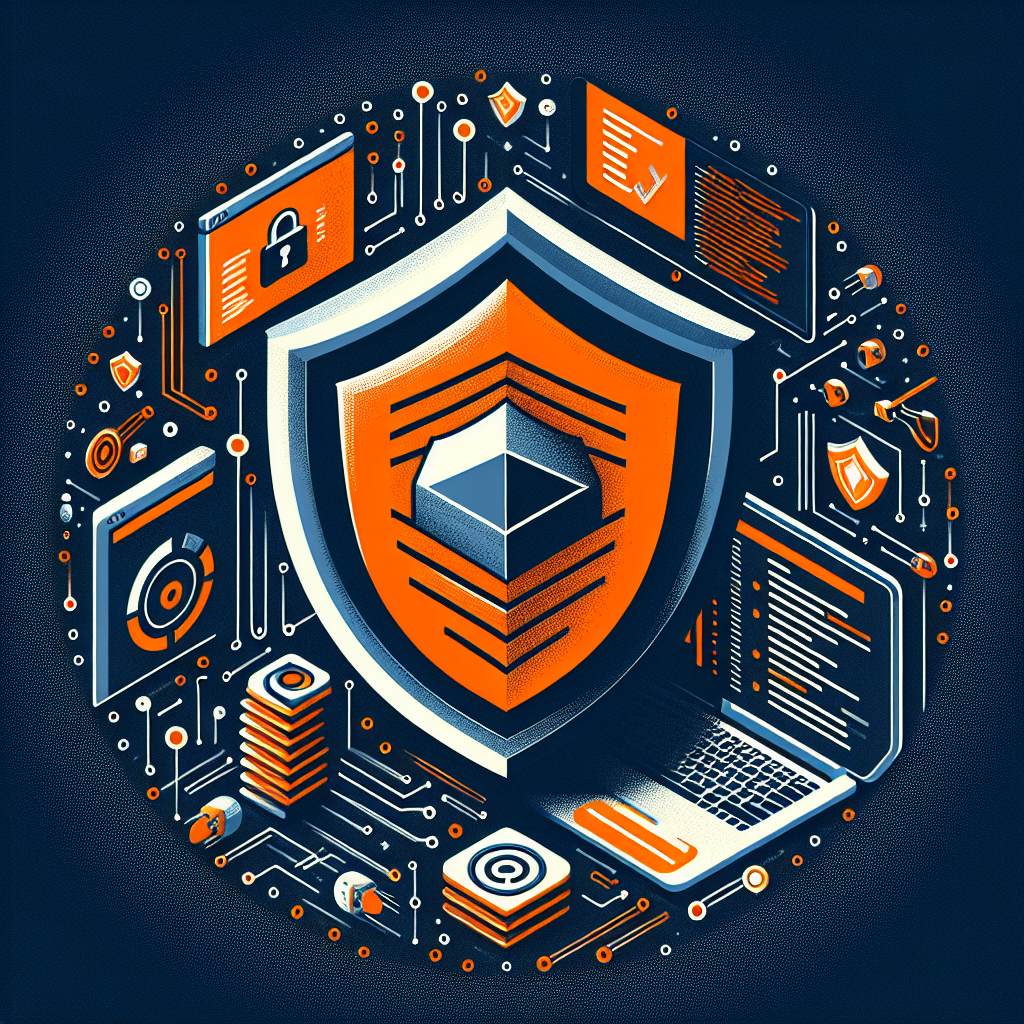
Magento 2 Security: Protecting Against SQL Injection and XSS Attacks
Hey there, fellow Magento enthusiast! If you're running a Magento 2 store, security should be at the top of your priority list. Today, we're diving into two of the most common and dangerous threats to your eCommerce site: SQL Injection (SQLi) and Cross-Site Scripting (XSS) attacks. Don't worry if these terms sound intimidating – by the end of this post, you'll understand exactly what they are and how to protect your store against them.
Why Should You Care About These Attacks?
Before we get into the technical stuff, let's understand why these attacks matter:
- SQL Injection can give attackers access to your entire database (customer info, orders, everything!)
- XSS Attacks can let hackers steal customer data or deface your store
- Both can lead to lost revenue, damaged reputation, and legal trouble
- Magento stores are prime targets because they handle sensitive financial data
Understanding SQL Injection Attacks
SQL Injection occurs when an attacker inserts malicious SQL code into your database queries. Here's a simple example of vulnerable code:
// BAD PRACTICE - Vulnerable to SQL Injection
$userId = $_GET['user_id'];
$sql = "SELECT * FROM users WHERE id = " . $userId;
An attacker could pass something like 1; DROP TABLE users;--
as the user_id parameter, which would delete your entire users table!
Protecting Against SQL Injection in Magento 2
Magento 2 provides several built-in protections, but you need to use them correctly:
1. Use Prepared Statements
Magento's database abstraction layer makes this easy:
// GOOD PRACTICE - Using prepared statements
$userId = $this->getRequest()->getParam('user_id');
$connection = $this->resourceConnection->getConnection();
$select = $connection->select()
->from('users')
->where('id = ?', $userId);
$result = $connection->fetchAll($select);
2. Always Use Magento's Data Filtering Methods
For direct queries, always escape values:
$escapedValue = $connection->quote($untrustedInput);
3. Validate All User Input
Use Magento's validation framework:
use Magento\Framework\Validator\EmailAddress;
$validator = new EmailAddress();
if (!$validator->isValid($email)) {
// Handle invalid input
}
Understanding XSS Attacks
Cross-Site Scripting occurs when attackers inject malicious JavaScript into your pages. This can:
- Steal customer session cookies
- Redirect users to phishing sites
- Modify page content
Here's an example of vulnerable code:
// BAD PRACTICE - Vulnerable to XSS
echo "Welcome back, " . $_GET['name'];
An attacker could craft a URL with name=<script>maliciousCode()</script>
to execute their script in your users' browsers.
Protecting Against XSS in Magento 2
1. Always Escape Output
Magento provides several escaping methods:
// In .phtml templates:
echo $block->escapeHtml($untrustedContent);
echo $block->escapeUrl($untrustedUrl);
echo $block->escapeJs($untrustedJs);
// In PHP classes:
use Magento\Framework\Escaper;
$this->escaper->escapeHtml($content);
2. Use Content Security Policy (CSP)
Magento 2.4+ includes CSP support. Configure it in app/etc/config.php
:
'csp' => [
'mode' => 'report-only',
'report_uri' => 'https://yourdomain.com/csp-report',
'policies' => [
'default-src' => ["'self'"],
'script-src' => ["'self'", "'unsafe-inline'", "trusted.cdn.com"],
// Other policies...
]
]
3. Sanitize User-Generated Content
For areas like product descriptions or CMS pages:
use Magento\Framework\Filter\Template\Tokenizer\Parameter;
$sanitizer = $this->templateTokenizerParameter;
$cleanContent = $sanitizer->filter($userContent);
Additional Security Best Practices
1. Keep Magento Updated
Always run the latest version. Check for updates with:
composer show magento/product-community-edition
2. Use Secure Extensions
Only install extensions from trusted sources like Magefine's marketplace. Check for:
- Regular updates
- Good reviews
- Secure coding practices
3. Implement Web Application Firewall (WAF)
Consider using:
- Magento's built-in security features
- Cloud-based WAF services
- Server-level protections like ModSecurity
4. Regular Security Audits
Use tools like:
- Magento Security Scan Tool
- OWASP ZAP
- Burp Suite
Real-World Example: Securing a Contact Form
Let's walk through securing a custom contact form:
// In your controller
public function execute()
{
$post = $this->getRequest()->getPostValue();
// Validate email
if (!\Zend_Validate::is($post['email'], 'EmailAddress')) {
$this->messageManager->addErrorMessage(__('Invalid email'));
return $this->resultRedirectFactory->create()->setPath('*/*/');
}
// Sanitize message
$message = $this->escaper->escapeHtml($post['message']);
// Use prepared statement to save to DB
$connection = $this->resourceConnection->getConnection();
$connection->insert(
'contact_requests',
[
'email' => $post['email'],
'message' => $message,
'created_at' => date('Y-m-d H:i:s')
]
);
// Send email with escaped content
$this->sendEmail(
$this->escaper->escapeHtml($post['email']),
$this->escaper->escapeHtml($post['name']),
$message
);
$this->messageManager->addSuccessMessage(__('Message sent!'));
return $this->resultRedirectFactory->create()->setPath('*/*/');
}
Monitoring and Incident Response
Even with protections, you should monitor for attacks:
- Set up Magento's built-in logging
- Monitor for unusual database activity
- Have a response plan for suspected breaches
- Keep regular backups (test restoring them!)
Final Thoughts
Securing your Magento 2 store against SQL Injection and XSS attacks isn't just about adding a few filters – it's about adopting a security mindset in everything you do. Remember:
- Never trust user input
- Always escape output
- Keep your system updated
- Use Magento's built-in security features
- Regularly audit your security
By implementing these practices, you'll significantly reduce your risk of these common but dangerous attacks. Your customers (and your peace of mind) will thank you!
For more Magento security solutions, check out Magefine's security extensions and secure hosting options designed specifically for Magento 2 stores.