How to Use Magento 2’s Built-in CSRF Protection for Enhanced Security
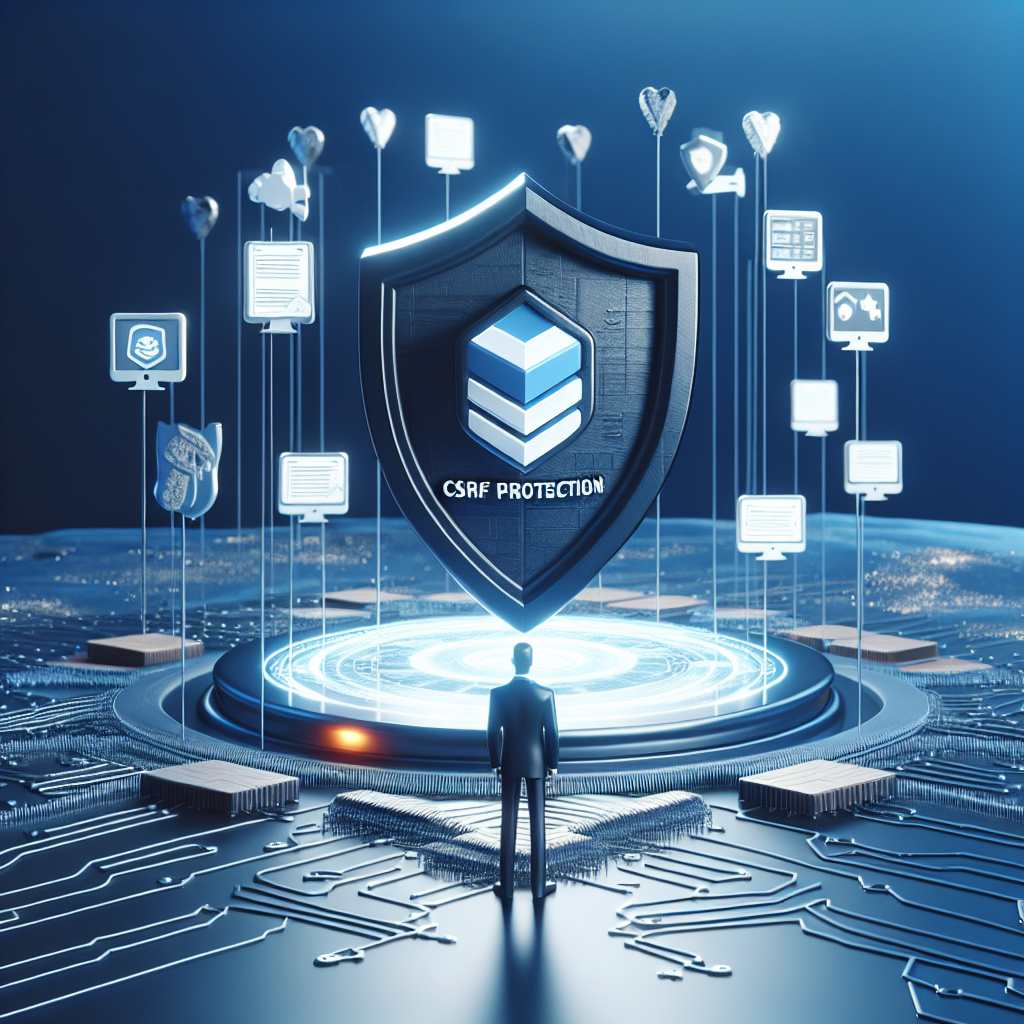
Understanding CSRF Attacks and Why Magento 2’s Protection Matters
Cross-Site Request Forgery (CSRF) attacks are sneaky. Imagine a hacker tricks your browser into performing unwanted actions on a site where you’re logged in—like changing your password or making a purchase without your consent. Scary, right? That’s why Magento 2 comes with built-in CSRF protection to keep your store secure.
Magento 2 implements CSRF protection using form keys—unique tokens generated for each user session. These tokens validate that form submissions come from legitimate sources, not malicious scripts. If you’ve ever seen a Form key is invalid
error, that’s Magento’s CSRF protection doing its job!
How Magento 2’s CSRF Protection Works
Here’s the breakdown:
- Token Generation: When a user loads a page with a form, Magento generates a unique form key tied to their session.
- Token Validation: When the form is submitted, Magento checks if the submitted token matches the one stored in the session.
- Request Blocking: If the tokens don’t match, Magento rejects the request.
This simple but effective mechanism prevents attackers from forging requests on behalf of authenticated users.
Enabling and Customizing CSRF Protection in Magento 2
Magento 2 enables CSRF protection by default, but let’s see how you can customize it for your needs.
1. Adding CSRF Protection to Custom Forms
If you’re creating a custom form in Magento 2, you need to include the form key manually. Here’s how:
<form action="<?php echo $block->getUrl('your/route/action') ?>" method="post">
<input type="hidden" name="form_key" value="<?php echo $block->getFormKey() ?>" />
<!-- Other form fields -->
<button type="submit">Submit</button>
</form>
This ensures your custom form is protected against CSRF attacks.
2. Disabling CSRF Protection for Specific Routes (When Necessary)
Sometimes, you might need to disable CSRF protection for specific API endpoints or AJAX calls. You can do this in your module’s di.xml
:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Framework\App\Request\CsrfValidator">
<arguments>
<argument name="excludedUrls" xsi:type="array">
<item name="your_route" xsi:type="string">/your/route/action</item>
</argument>
</arguments>
</type>
</config>
Warning: Only disable CSRF protection if absolutely necessary, as it weakens security.
3. Handling CSRF in AJAX Requests
For AJAX requests, you need to pass the form key in the request headers or data payload. Here’s an example using jQuery:
$.ajax({
url: '<?php echo $block->getUrl('your/route/action') ?>',
type: 'POST',
data: {
form_key: '<?php echo $block->getFormKey() ?>',
// Other data fields
},
success: function(response) {
console.log(response);
}
});
Testing Your CSRF Protection
It’s always good to verify that your CSRF protection is working. Here’s a quick test:
- Log in to your Magento admin.
- Open the browser’s Developer Tools (F12).
- Find a form submission request in the Network tab.
- Copy the request as a cURL command.
- Remove or alter the
form_key
parameter. - Execute the modified cURL command.
If Magento returns a 403 Forbidden
or Form key is invalid
error, your CSRF protection is working correctly!
Common Issues and Fixes
1. Form Key Expiration
Magento’s form keys expire when the session ends. If users face frequent Invalid Form Key
errors, check:
- Session timeout settings in
app/etc/env.php
. - Cookie domain and path configurations.
2. Caching Issues with Form Keys
If you’re using Full Page Cache (FPC), dynamic form keys might get cached. To fix this:
- Exclude forms from FPC.
- Use JavaScript to dynamically insert form keys.
3. CSRF Errors in Custom Modules
If your custom module throws CSRF errors, ensure:
- Your forms include
<?php echo $block->getFormKey() ?>
. - AJAX requests send the correct form key.
Best Practices for CSRF Protection in Magento 2
- Always use form keys in admin and frontend forms.
- Avoid disabling CSRF protection unless absolutely necessary.
- Test your forms to ensure they work with CSRF validation.
- Monitor logs for repeated CSRF failures—they might indicate attack attempts.
Final Thoughts
Magento 2’s built-in CSRF protection is a powerful security feature that helps prevent unauthorized actions. By understanding how it works and implementing it correctly in your custom forms and modules, you can keep your store safe from malicious attacks.
Need help securing your Magento store? Check out our Magento security extensions for enhanced protection!