How to Send Custom Emails in Magento 2 Programmatically
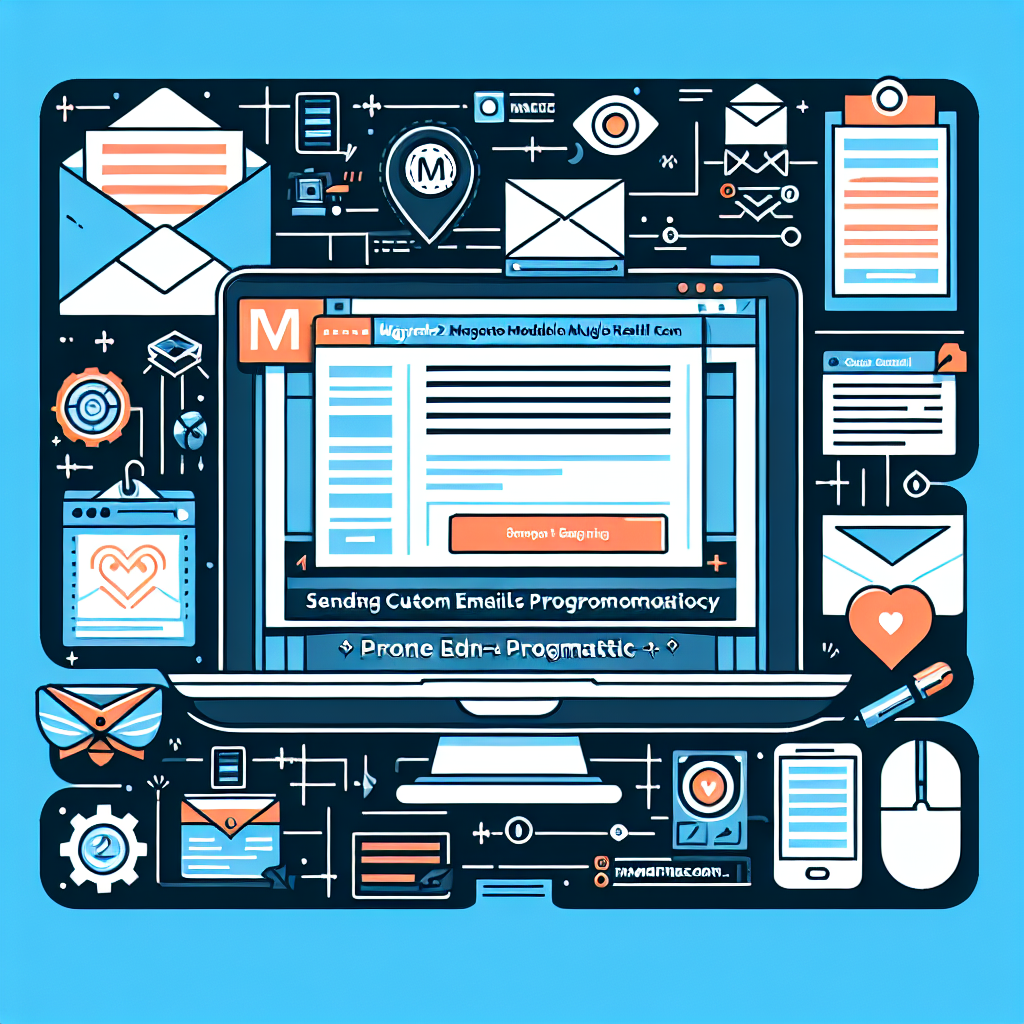
How to Send Custom Emails in Magento 2 Programmatically
If you're running an online store with Magento 2, you know how important it is to communicate with your customers. Whether it's order confirmations, shipping updates, or promotional emails, sending the right message at the right time can make a big difference. But what if you want to send custom emails that aren't covered by Magento's default email templates? That's where programmatic email sending comes in.
In this post, we'll walk you through how to send custom emails in Magento 2 programmatically. We'll cover everything from setting up your email template to writing the code that sends the email. By the end, you'll be able to send custom emails tailored to your specific needs.
Why Send Custom Emails Programmatically?
Magento 2 comes with a variety of built-in email templates for common scenarios like order confirmations, shipment notifications, and password resets. However, there are times when you need to send emails that go beyond these templates. For example, you might want to send a personalized thank-you email after a customer makes their first purchase, or notify your team when a high-value order is placed.
By sending custom emails programmatically, you can:
- Create highly personalized messages.
- Trigger emails based on specific events or conditions.
- Integrate with third-party services or APIs.
- Automate repetitive tasks, saving you time and effort.
Step 1: Create a Custom Email Template
Before you can send a custom email, you need to create an email template. Magento 2 allows you to create and manage email templates from the admin panel. Here's how:
- Log in to your Magento 2 admin panel.
- Navigate to Marketing > Communications > Email Templates.
- Click on Add New Template.
- Select a template to start from (or choose "Custom Template" if you want to start from scratch).
- Fill in the template details, including the template name, subject, and content.
- Use Magento's template variables to dynamically insert data like customer names, order numbers, or product details.
- Save the template.
For example, here's a simple email template:
<p>Hello {{var customer_name}},</p>
<p>Thank you for your recent purchase! Your order number is {{var order_number}}.</p>
<p>We hope you enjoy your new items.</p>
<p>Best regards,</p>
<p>The {{var store_name}} Team</p>
Once you've created your template, note down its Template Identifier. You'll need this in the next step.
Step 2: Write the Code to Send the Email
Now that you have your email template, it's time to write the code that sends the email. Magento 2 provides a robust email framework that you can use to send emails programmatically. Here's how to do it:
- Create a custom module (if you don't already have one).
- In your module, create a new PHP file to handle the email sending logic.
- Use Magento's
TransportBuilder
class to build and send the email.
Here's an example of how to send a custom email:
<?php
namespace Vendor\Module\Controller\Index;
use Magento\Framework\App\Action\Action;
use Magento\Framework\App\Action\Context;
use Magento\Framework\Mail\Template\TransportBuilder;
use Magento\Framework\Translate\Inline\StateInterface;
use Magento\Store\Model\StoreManagerInterface;
class SendEmail extends Action
{
protected $transportBuilder;
protected $inlineTranslation;
protected $storeManager;
public function __construct(
Context $context,
TransportBuilder $transportBuilder,
StateInterface $inlineTranslation,
StoreManagerInterface $storeManager
) {
$this->transportBuilder = $transportBuilder;
$this->inlineTranslation = $inlineTranslation;
$this->storeManager = $storeManager;
parent::__construct($context);
}
public function execute()
{
// Disable inline translation to avoid issues with email templates
$this->inlineTranslation->suspend();
try {
// Set the template variables
$templateVars = [
'customer_name' => 'John Doe',
'order_number' => '123456',
'store_name' => 'My Store'
];
// Set the sender and recipient
$sender = [
'name' => 'My Store',
'email' => 'noreply@mystore.com'
];
$recipient = 'customer@example.com';
// Build the email
$transport = $this->transportBuilder
->setTemplateIdentifier('your_template_identifier') // Template ID
->setTemplateOptions([
'area' => \Magento\Framework\App\Area::AREA_FRONTEND,
'store' => $this->storeManager->getStore()->getId()
])
->setTemplateVars($templateVars)
->setFrom($sender)
->addTo($recipient)
->getTransport();
// Send the email
$transport->sendMessage();
// Re-enable inline translation
$this->inlineTranslation->resume();
echo 'Email sent successfully!';
} catch (\Exception $e) {
echo 'Error: ' . $e->getMessage();
}
}
}
In this example, we're using the TransportBuilder
class to build and send the email. The setTemplateIdentifier
method specifies the email template to use, while setTemplateVars
sets the variables that will be replaced in the template.
Step 3: Trigger the Email
Now that you have the code to send the email, you need to decide when and where to trigger it. Here are a few common scenarios:
- After a customer places an order: You can use an observer to trigger the email after the
checkout_submit_all_after
event. - When a new customer registers: Use the
customer_register_success
event to send a welcome email. - When a product goes on sale: Create a cron job to check for price changes and send an email to interested customers.
Here's an example of how to trigger the email using an observer:
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
use Vendor\Module\Controller\Index\SendEmail;
class SendCustomEmail implements ObserverInterface
{
protected $sendEmail;
public function __construct(SendEmail $sendEmail)
{
$this->sendEmail = $sendEmail;
}
public function execute(Observer $observer)
{
// Trigger the email sending logic
$this->sendEmail->execute();
}
}
Don't forget to register your observer in your module's events.xml
file:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="checkout_submit_all_after">
<observer name="send_custom_email" instance="Vendor\Module\Observer\SendCustomEmail"/>
</event>
</config>
Step 4: Test Your Custom Email
Before deploying your custom email functionality, it's important to test it thoroughly. Here's how:
- Place a test order or trigger the event that should send the email.
- Check your email inbox (or the recipient's inbox) to ensure the email is sent correctly.
- Verify that all template variables are replaced with the correct data.
- Test different scenarios, such as invalid email addresses or missing template variables, to ensure your code handles errors gracefully.
Conclusion
Sending custom emails in Magento 2 programmatically is a powerful way to enhance your store's communication capabilities. By creating custom email templates and using Magento's email framework, you can send personalized, event-driven emails that meet your specific needs. Whether you're thanking customers for their orders, notifying your team about important events, or promoting new products, custom emails can help you build stronger relationships with your audience.
If you're looking for a reliable hosting solution to support your Magento 2 store, check out Magefine. We offer optimized Magento hosting plans that ensure your store runs smoothly and efficiently.
Happy coding!