How to Implement a Custom Report in Magento 2 Admin
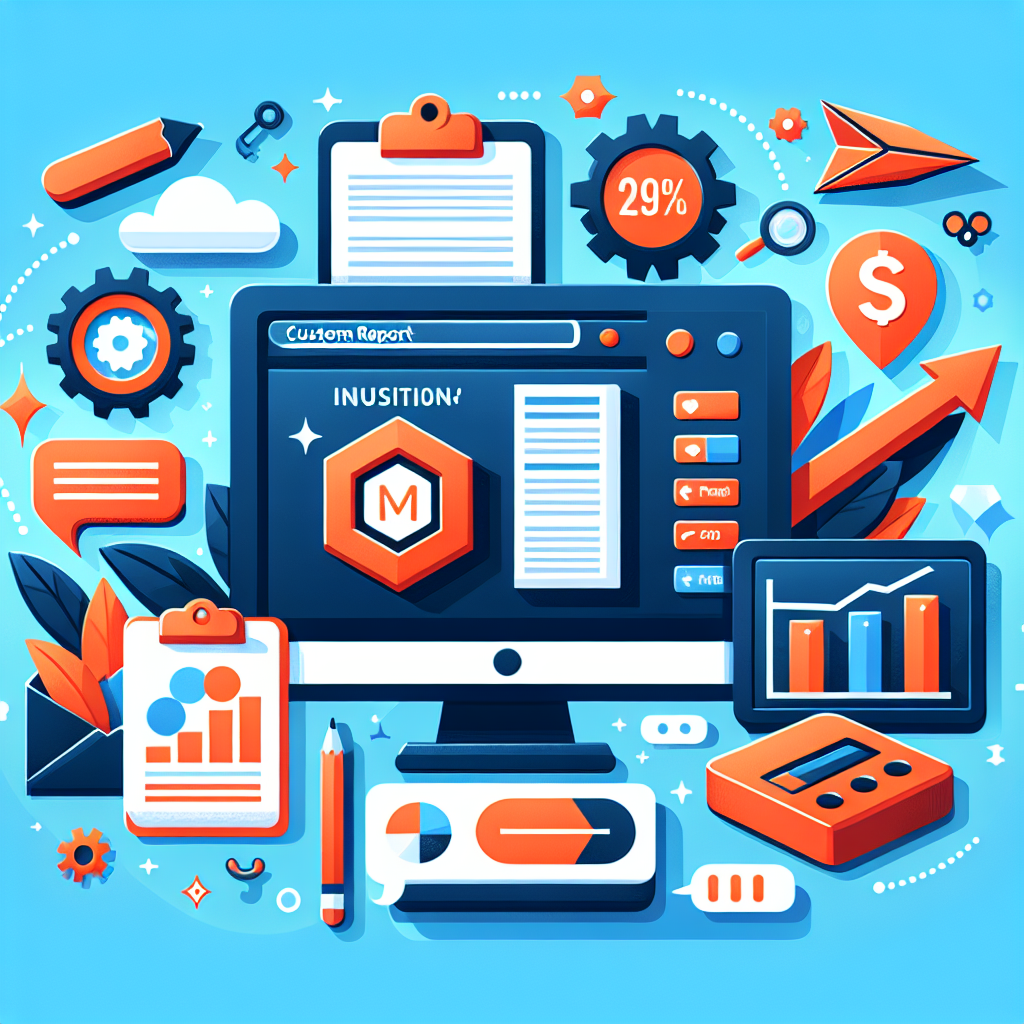
How to Implement a Custom Report in Magento 2 Admin
If you're running a Magento 2 store, you know how important it is to have access to the right data. Magento 2 comes with a variety of built-in reports, but sometimes you need something more specific to your business needs. That's where custom reports come in. In this post, we'll walk you through the process of creating a custom report in Magento 2 Admin. Don't worry if you're new to this; we'll take it step by step.
Why Create a Custom Report?
Custom reports allow you to analyze data that is specific to your business. Whether you need to track sales by a specific category, monitor customer behavior, or analyze product performance, custom reports can provide the insights you need. By creating a custom report, you can tailor the data to your exact requirements, making it easier to make informed business decisions.
Step 1: Set Up Your Module
First, you'll need to create a new module for your custom report. If you're not familiar with creating modules in Magento 2, don't worry—it's straightforward. Here's how you can do it:
app/code/YourVendor/YourModule/registration.php
In this file, you'll register your module with Magento:
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'YourVendor_YourModule',
__DIR__
);
Next, create the module.xml
file:
app/code/YourVendor/YourModule/etc/module.xml
And add the following content:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="YourVendor_YourModule" setup_version="1.0.0"/>
</config>
Step 2: Create the Report Grid
Now that your module is set up, it's time to create the report grid. The grid will display the data in the Magento 2 Admin panel. To do this, you'll need to create a few files:
app/code/YourVendor/YourModule/Block/Adminhtml/Report/YourReport.php
In this file, you'll define the grid:
<?php
namespace YourVendor\YourModule\Block\Adminhtml\Report;
class YourReport extends \Magento\Backend\Block\Widget\Grid\Container
{
protected function _construct()
{
$this->_blockGroup = 'YourVendor_YourModule';
$this->_controller = 'adminhtml_report_yourreport';
$this->_headerText = __('Your Custom Report');
parent::_construct();
$this->buttonList->remove('add');
}
}
Next, create the grid file:
app/code/YourVendor/YourModule/Block/Adminhtml/Report/YourReport/Grid.php
And add the following content:
<?php
namespace YourVendor\YourModule\Block\Adminhtml\Report\YourReport;
class Grid extends \Magento\Backend\Block\Widget\Grid\Extended
{
protected $_collectionFactory;
public function __construct(
\Magento\Backend\Block\Template\Context $context,
\Magento\Backend\Helper\Data $backendHelper,
\YourVendor\YourModule\Model\ResourceModel\YourReport\CollectionFactory $collectionFactory,
array $data = []
) {
$this->_collectionFactory = $collectionFactory;
parent::__construct($context, $backendHelper, $data);
}
protected function _prepareCollection()
{
$this->setCollection($this->_collectionFactory->create());
return parent::_prepareCollection();
}
protected function _prepareColumns()
{
$this->addColumn('entity_id', [
'header' => __('ID'),
'index' => 'entity_id',
]);
$this->addColumn('name', [
'header' => __('Name'),
'index' => 'name',
]);
return parent::_prepareColumns();
}
}
Step 3: Create the Collection
The collection is responsible for fetching the data that will be displayed in the grid. Create the collection file:
app/code/YourVendor/YourModule/Model/ResourceModel/YourReport/Collection.php
And add the following content:
<?php
namespace YourVendor\YourModule\Model\ResourceModel\YourReport;
class Collection extends \Magento\Framework\Model\ResourceModel\Db\Collection\AbstractCollection
{
protected function _construct()
{
$this->_init(
'YourVendor\YourModule\Model\YourReport',
'YourVendor\YourModule\Model\ResourceModel\YourReport'
);
}
}
Next, create the collection factory:
app/code/YourVendor/YourModule/Model/ResourceModel/YourReport/CollectionFactory.php
And add the following content:
<?php
namespace YourVendor\YourModule\Model\ResourceModel\YourReport;
class CollectionFactory
{
protected $_objectManager = null;
protected $_instanceName = null;
public function __construct(
\Magento\Framework\ObjectManagerInterface $objectManager,
$instanceName = '\\YourVendor\\YourModule\\Model\\ResourceModel\\YourReport\\Collection'
) {
$this->_objectManager = $objectManager;
$this->_instanceName = $instanceName;
}
public function create(array $data = [])
{
return $this->_objectManager->create($this->_instanceName, $data);
}
}
Step 4: Create the Layout and Menu
Now that your grid and collection are set up, you'll need to create the layout and menu for your custom report. First, create the layout file:
app/code/YourVendor/YourModule/view/adminhtml/layout/yourmodule_report_yourreport_index.xml
And add the following content:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<block class="YourVendor\YourModule\Block\Adminhtml\Report\YourReport" name="yourmodule_report_yourreport"/>
</referenceContainer>
</body>
</page>
Next, create the menu file:
app/code/YourVendor/YourModule/etc/adminhtml/menu.xml
And add the following content:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Backend:etc/menu.xsd">
<menu>
<add id="YourVendor_YourModule::report" title="Custom Reports" module="YourVendor_YourModule" sortOrder="10" resource="Magento_Backend::content"/>
<add id="YourVendor_YourModule::report_yourreport" title="Your Custom Report" module="YourVendor_YourModule" sortOrder="10" parent="YourVendor_YourModule::report" action="yourmodule/report_yourreport" resource="Magento_Backend::content"/>
</menu>
</config>
Step 5: Create the Controller
Finally, you'll need to create the controller that will handle the request for your custom report. Create the controller file:
app/code/YourVendor/YourModule/Controller/Adminhtml/Report/YourReport/Index.php
And add the following content:
<?php
namespace YourVendor\YourModule\Controller\Adminhtml\Report\YourReport;
class Index extends \Magento\Backend\App\Action
{
protected $resultPageFactory;
public function __construct(
\Magento\Backend\App\Action\Context $context,
\Magento\Framework\View\Result\PageFactory $resultPageFactory
) {
parent::__construct($context);
$this->resultPageFactory = $resultPageFactory;
}
public function execute()
{
$resultPage = $this->resultPageFactory->create();
$resultPage->setActiveMenu('YourVendor_YourModule::report_yourreport');
$resultPage->getConfig()->getTitle()->prepend(__('Your Custom Report'));
return $resultPage;
}
}
Step 6: Test Your Custom Report
Once you've completed all the steps, it's time to test your custom report. Log in to your Magento 2 Admin panel and navigate to the "Custom Reports" section. You should see your custom report listed there. Click on it, and you should see the grid with the data you defined.
If everything looks good, congratulations! You've successfully created a custom report in Magento 2 Admin. If you encounter any issues, double-check your code and make sure all files are in the correct locations.
Conclusion
Creating a custom report in Magento 2 Admin might seem daunting at first, but by following these steps, you can easily tailor your reports to meet your business needs. Whether you're tracking sales, monitoring customer behavior, or analyzing product performance, custom reports can provide the insights you need to make informed decisions. Happy reporting!