How to Implement a "Buy Now, Pay Later" Solution in Magento 2
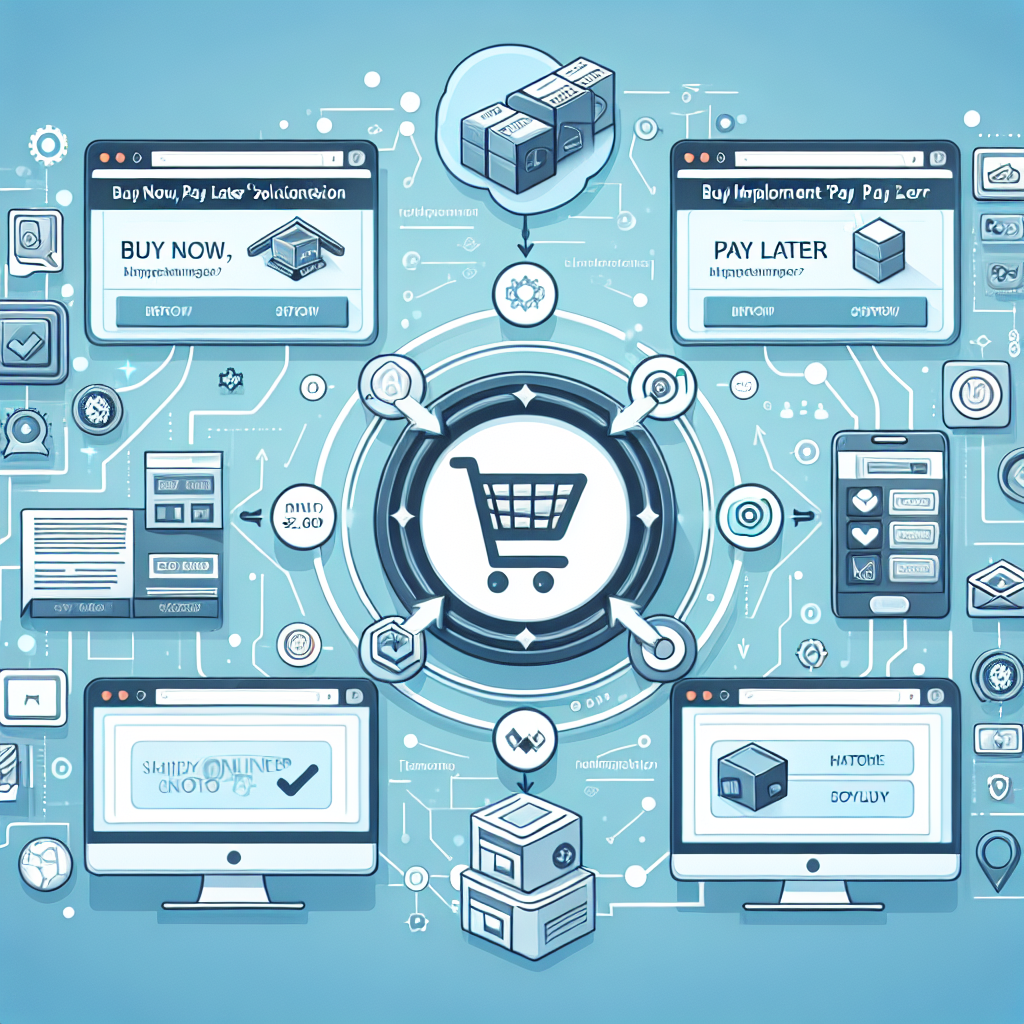
What is "Buy Now, Pay Later" (BNPL) in Magento 2?
"Buy Now, Pay Later" (BNPL) is a payment solution that allows customers to purchase products immediately and pay for them in installments over time. This flexible payment method has become increasingly popular in eCommerce, as it helps increase conversion rates and average order values by reducing financial barriers for shoppers.
In Magento 2, you can implement BNPL either through third-party payment providers (like Afterpay, Klarna, or Affirm) or by creating a custom installment payment solution. Let’s explore both approaches.
Option 1: Integrating Third-Party BNPL Providers
Many BNPL providers offer Magento 2 extensions to simplify integration. Here’s how to set up Klarna as an example:
Step 1: Install the Klarna Extension
You can install the Klarna module via Composer:
composer require klarna/m2-payments
Step 2: Configure Klarna in Magento Admin
Navigate to Stores > Configuration > Sales > Payment Methods > Klarna and enter your merchant credentials.
Step 3: Enable Payment Options
Choose which BNPL options to display (Pay Later, Slice It, Financing). Save the configuration.
Step 4: Customize Checkout Display
Klarna will now appear as a payment option at checkout. You can adjust the widget styling via the extension settings.
Option 2: Creating a Custom BNPL Solution
If you prefer more control, you can build a custom BNPL solution. Here’s a basic implementation approach:
Step 1: Create a Custom Payment Module
Set up a new module with the following structure:
app/code/Vendor/BuyNowPayLater/
├── etc/
│ ├── adminhtml/system.xml
│ ├── config.xml
│ ├── module.xml
│ └── payment.xml
├── Model/
│ ├── Method/
│ │ └── BuyNowPayLater.php
│ └── Ui/
│ └── ConfigProvider.php
└── view/
└── frontend/
├── layout/
├── templates/
└── web/template/payment/buynowpaylater.html
Step 2: Define the Payment Method
Create the payment method class (BuyNowPayLater.php
):
<?php
namespace Vendor\BuyNowPayLater\Model\Method;
use Magento\Payment\Model\Method\AbstractMethod;
class BuyNowPayLater extends AbstractMethod
{
const CODE = 'buynowpaylater';
protected $_code = self::CODE;
protected $_isOffline = true;
protected $_canAuthorize = true;
protected $_canCapture = true;
public function authorize(\Magento\Payment\Model\InfoInterface $payment, $amount)
{
// Custom authorization logic
return $this;
}
}
Step 3: Configure Payment Options
Create payment.xml
to define your BNPL options:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Payment:etc/payment.xsd">
<payment>
<groups>
<group id="buynowpaylater">
<label>Buy Now, Pay Later</label>
</group>
</groups>
</payment>
</config>
Step 4: Add Installment Options
Extend the checkout with custom installment options in your template:
<div class="payment-method" data-bind="css: {'_active': (getCode() == 'buynowpaylater')}">
<div class="payment-method-title field choice">
<input type="radio"
name="payment[method]"
class="radio"
data-bind="attr: {'id': getCode()}, value: getCode(), checked: isChecked, click: selectPaymentMethod" />
<label data-bind="attr: {'for': getCode()}" class="label">
<span data-bind="text: getTitle()"></span>
</label>
</div>
<div class="payment-method-content">
<div class="installment-options">
<h3>Choose Payment Plan:</h3>
<select data-bind="value: installmentOption">
<option value="1">Pay in full now</option>
<option value="4">4 interest-free payments</option>
<option value="6">6 monthly payments (with interest)</option>
</select>
</div>
</div>
</div>
Best Practices for BNPL Implementation
- Clear Disclosure: Always display installment terms and any fees upfront
- Mobile Optimization: Ensure BNPL options work smoothly on mobile devices
- Fraud Prevention: Implement credit checks or order limits for installment payments
- Order Status Handling: Create custom order statuses for installment payments
Conclusion
Adding BNPL to your Magento 2 store can significantly boost conversions and average order values. Whether you choose a third-party solution or build a custom implementation, ensure the payment flow is seamless and transparent for customers.
For stores needing advanced BNPL features, consider exploring Magento 2 extensions from Magefine that offer ready-made solutions with additional functionality like automated payment scheduling and customer credit management.