How to Implement a Console Command in Magento 2
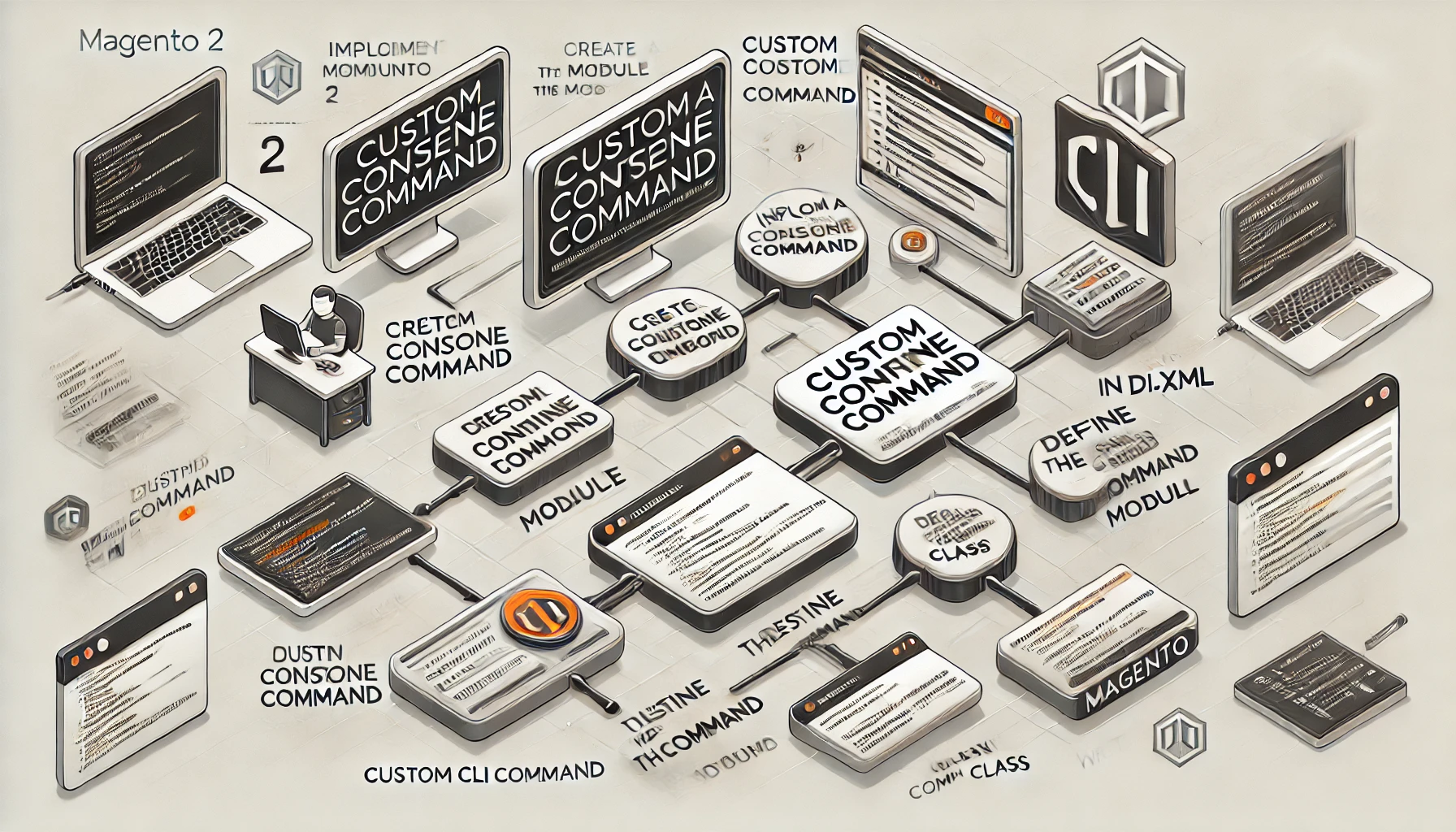
Magento 2 provides a powerful and extensible command-line interface (CLI) for developers and administrators to perform various tasks such as running cron jobs, reindexing, clearing caches, and deploying the store. While Magento includes several built-in commands, you can extend this functionality by creating custom console commands tailored to your specific business or development needs.
What Is a Console Command in Magento 2?
In Magento 2, a console command is a CLI tool that allows developers or administrators to interact with the Magento system directly through the terminal. These commands provide an efficient way to automate tasks, access data, or trigger custom functionality that would be cumbersome to achieve via the admin panel.
Common Use Cases
- Exporting or importing data.
- Running background tasks or processes.
- Debugging and logging specific information.
- Automating repetitive workflows.
Steps to Implement a Console Command in Magento 2
1. Create the Module Skeleton
Create the necessary directory structure:
app/code/Vendor/Module/
├── Console/
├── etc/
│ ├── module.xml
└── registration.php
2. Register the Module
Create the registration.php
file:
<?php
use Magento\Framework\Component\ComponentRegistrar;
ComponentRegistrar::register(
ComponentRegistrar::MODULE,
'Vendor_Module',
__DIR__
);
3. Define the Module Configuration
Create the module.xml
file:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_Module" setup_version="1.0.0"/>
</config>
4. Create the Console Command Class
Define your custom logic in the CustomCommand.php
file:
<?php
namespace Vendor\Module\Console;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
class CustomCommand extends Command
{
protected function configure()
{
$this->setName('vendor:custom:command')
->setDescription('This is a custom console command in Magento 2');
}
protected function execute(InputInterface $input, OutputInterface $output)
{
$output->writeln("Hello! This is your custom Magento 2 command.");
return Command::SUCCESS;
}
}
5. Register the Command in di.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Framework\Console\CommandList">
<arguments>
<argument name="commands" xsi:type="array">
<item name="vendor_custom_command" xsi:type="object">Vendor\Module\Console\CustomCommand</item>
</argument>
</arguments>
</type>
</config>
6. Enable the Module and Test the Command
Run the following commands:
php bin/magento module:enable Vendor_Module
php bin/magento setup:upgrade
php bin/magento vendor:custom:command
Output:
Hello! This is your custom Magento 2 command.
Practical Example: Exporting Product Data
Modify your command to export product data to a CSV file:
<?php
namespace Vendor\Module\Console;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
use Magento\Catalog\Model\ResourceModel\Product\CollectionFactory;
use Magento\Framework\App\Filesystem\DirectoryList;
class ExportProductsCommand extends Command
{
private $productCollectionFactory;
private $directoryList;
public function __construct(
CollectionFactory $productCollectionFactory,
DirectoryList $directoryList
) {
$this->productCollectionFactory = $productCollectionFactory;
$this->directoryList = $directoryList;
parent::__construct();
}
protected function configure()
{
$this->setName('vendor:export:products')
->setDescription('Export product data to a CSV file');
}
protected function execute(InputInterface $input, OutputInterface $output)
{
$productCollection = $this->productCollectionFactory->create();
$productCollection->addAttributeToSelect(['name', 'sku', 'price']);
$csvFilePath = $this->directoryList->getPath(DirectoryList::VAR_DIR) . '/export/products.csv';
$csvFile = fopen($csvFilePath, 'w');
fputcsv($csvFile, ['Name', 'SKU', 'Price']);
foreach ($productCollection as $product) {
fputcsv($csvFile, [$product->getName(), $product->getSku(), $product->getPrice()]);
}
fclose($csvFile);
$output->writeln("Products exported to: " . $csvFilePath);
return Command::SUCCESS;
}
}
Best Practices for Custom Console Commands
- Use Dependency Injection (DI): Inject services and factories through the constructor.
- Provide Clear Descriptions: Make command names and descriptions meaningful.
- Handle Errors Gracefully: Add error handling for better user experience.
- Optimize Performance: Use pagination or batch processing for large datasets.