Magento 2 and Webhooks: Real-Time Integrations with External Services
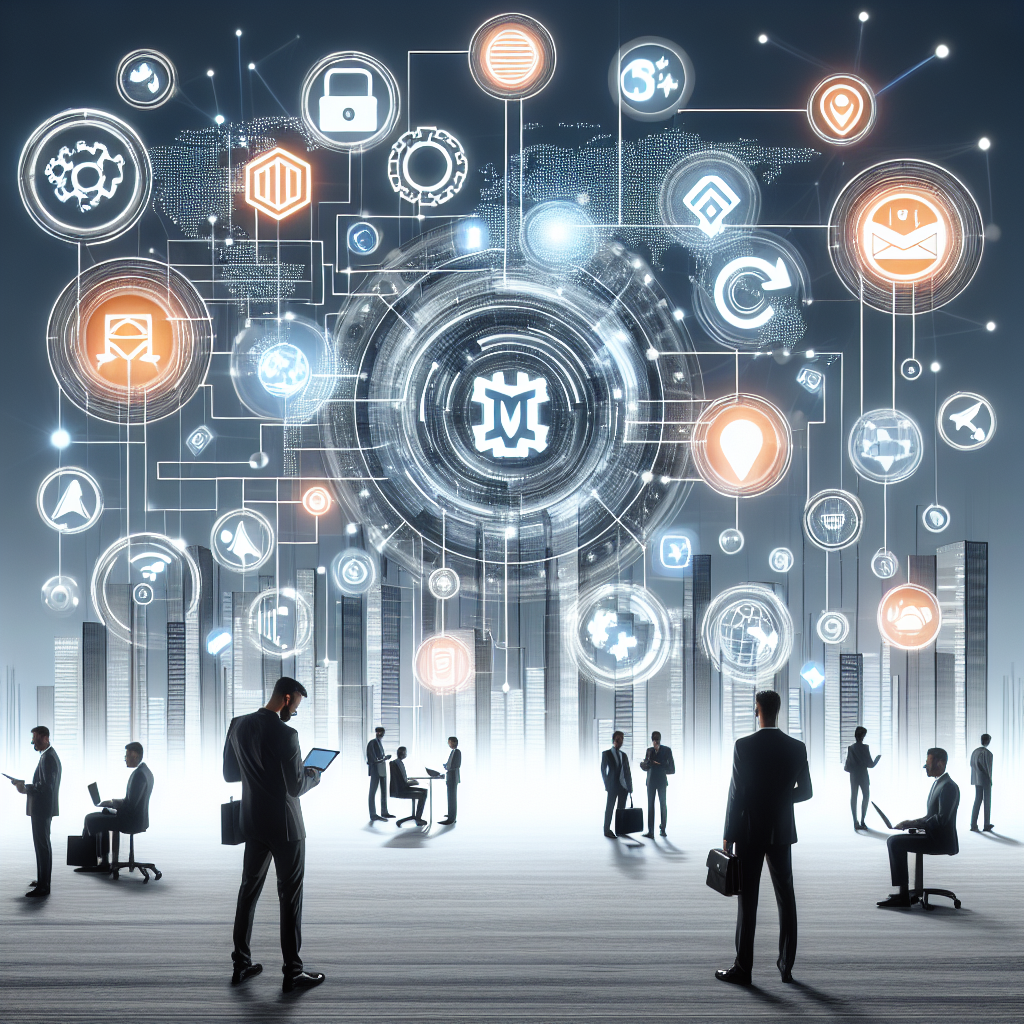
Magento 2 and Webhooks: Real-Time Integrations with External Services
If you're running a Magento 2 store, you've probably heard about webhooks. They're like digital messengers that instantly notify other systems when something happens in your store. No more waiting around for scheduled updates – webhooks keep everything in sync in real-time.
In this post, we'll break down how webhooks work in Magento 2 and show you exactly how to set them up for seamless integrations with payment gateways, CRMs, ERPs, and other external services.
What Exactly Are Webhooks?
Webhooks are automated messages sent from apps when something happens. They're essentially user-defined HTTP callbacks triggered by specific events. When the event occurs, the source app makes an HTTP request to the URL configured for the webhook.
Think of them like phone notifications for your apps. Instead of your apps constantly checking for updates (which is inefficient), they get pinged immediately when there's something new.
Why Use Webhooks with Magento 2?
Here's why webhooks are game-changers for Magento stores:
- Real-time data sync: No more waiting for cron jobs or manual exports
- Reduced server load: More efficient than constant API polling
- Automated workflows: Trigger actions in other systems instantly
- Better customer experiences: Keep all systems updated simultaneously
Common Use Cases for Magento 2 Webhooks
Some practical examples where webhooks shine:
- Notify your CRM when a new customer registers
- Alert your inventory system when products sell out
- Trigger shipping notifications when orders are processed
- Update accounting software with new orders
- Sync customer data with email marketing platforms
How to Implement Webhooks in Magento 2
Let's walk through setting up webhooks in Magento 2. We'll create a simple module that sends order data to an external service whenever a new order is placed.
Step 1: Create the Basic Module Structure
First, create these files in your module directory (app/code/Vendor/Webhooks):
app/code/Vendor/Webhooks/
├── etc
│ ├── module.xml
│ └── events.xml
├── Model
│ └── Webhook.php
└── registration.php
Step 2: Set Up the Module Configuration
Create the module.xml file:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_Webhooks" setup_version="1.0.0">
<sequence>
<module name="Magento_Sales"/>
</sequence>
</module>
</config>
And the registration.php:
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_Webhooks',
__DIR__
);
Step 3: Configure the Event Observer
In events.xml, we'll listen for the sales_order_place_after event:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="sales_order_place_after">
<observer name="vendor_webhooks_order_place" instance="Vendor\Webhooks\Model\Webhook"/>
</event>
</config>
Step 4: Create the Webhook Logic
Now let's implement the Webhook.php model:
<?php
namespace Vendor\Webhooks\Model;
use Magento\Framework\Event\ObserverInterface;
use Magento\Framework\Event\Observer;
use Magento\Framework\HTTP\Client\Curl;
class Webhook implements ObserverInterface
{
protected $_curl;
public function __construct(
Curl $curl
) {
$this->_curl = $curl;
}
public function execute(Observer $observer)
{
$order = $observer->getEvent()->getOrder();
$webhookUrl = 'https://your-external-service.com/webhook';
$data = [
'event' => 'order_placed',
'order_id' => $order->getIncrementId(),
'customer_email' => $order->getCustomerEmail(),
'total' => $order->getGrandTotal(),
'items' => []
];
foreach ($order->getAllItems() as $item) {
$data['items'][] = [
'sku' => $item->getSku(),
'name' => $item->getName(),
'price' => $item->getPrice(),
'qty' => $item->getQtyOrdered()
];
}
$this->_curl->post($webhookUrl, json_encode($data));
}
}
Step 5: Enable and Test Your Module
Run these commands to enable your module:
php bin/magento module:enable Vendor_Webhooks
php bin/magento setup:upgrade
php bin/magento cache:clean
Now place a test order in your store. You should see the order data being sent to your webhook URL immediately after order placement.
Advanced Webhook Implementation Tips
For production use, you'll want to add some refinements:
1. Add Error Handling
Modify your Webhook.php to handle errors gracefully:
public function execute(Observer $observer)
{
try {
$order = $observer->getEvent()->getOrder();
$webhookUrl = 'https://your-external-service.com/webhook';
// Prepare data...
$this->_curl->setOption(CURLOPT_TIMEOUT, 10);
$this->_curl->setOption(CURLOPT_RETURNTRANSFER, true);
$this->_curl->addHeader("Content-Type", "application/json");
$this->_curl->post($webhookUrl, json_encode($data));
if ($this->_curl->getStatus() != 200) {
// Log error
throw new \Exception('Webhook failed with status: ' . $this->_curl->getStatus());
}
} catch (\Exception $e) {
// Log the error
$this->_logger->error($e->getMessage());
}
}
2. Implement Retry Logic
For temporary failures, implement a retry mechanism:
public function sendWebhookWithRetry($url, $data, $maxRetries = 3)
{
$retryCount = 0;
$success = false;
while (!$success && $retryCount < $maxRetries) {
try {
$this->_curl->post($url, json_encode($data));
if ($this->_curl->getStatus() == 200) {
$success = true;
} else {
$retryCount++;
sleep(pow(2, $retryCount)); // Exponential backoff
}
} catch (\Exception $e) {
$retryCount++;
sleep(pow(2, $retryCount));
}
}
if (!$success) {
// Log final failure
}
return $success;
}
3. Secure Your Webhooks
Add security by implementing:
- Signature verification
- IP whitelisting
- Authentication tokens
Here's how to add a signature header:
$secret = 'your_shared_secret';
$payload = json_encode($data);
$signature = hash_hmac('sha256', $payload, $secret);
$this->_curl->addHeader("X-Webhook-Signature", $signature);
$this->_curl->post($webhookUrl, $payload);
Popular Services That Support Magento 2 Webhooks
Many third-party services offer webhook integrations with Magento 2. Here are some popular ones:
- Payment Processors: Stripe, PayPal, Braintree
- CRMs: Salesforce, HubSpot, Zoho
- ERPs: SAP, NetSuite, Microsoft Dynamics
- Marketing Automation: Mailchimp, Klaviyo, ActiveCampaign
- Shipping: ShipStation, Shippo, EasyPost
Monitoring and Debugging Webhooks
When things go wrong (and they will), here's how to troubleshoot:
- Check Magento logs: var/log/system.log and var/log/exception.log
- Use a webhook testing service: RequestBin or Webhook.site
- Monitor response times: Long delays might indicate performance issues
- Implement logging: Log all webhook requests and responses
Webhooks vs. Magento 2 REST API
While both can integrate systems, they serve different purposes:
Feature | Webhooks | REST API |
---|---|---|
Direction | Push (outbound) | Pull (inbound) |
Timing | Real-time | On-demand |
Complexity | Simple event-based | Complex query capabilities |
Server Load | Low (only when events occur) | High (constant polling) |
In many cases, you'll want to use both together for comprehensive integration.
Performance Considerations
While webhooks are efficient, some best practices will keep your store running smoothly:
- Queue webhook processing: Use message queues for high-volume events
- Limit payload size: Only send essential data
- Implement rate limiting: Prevent overwhelming external services
- Use async processing: Don't make customers wait for webhook completion
Ready-Made Webhook Solutions for Magento 2
If you'd rather not build from scratch, consider these extensions:
- MageFine Webhooks Pro: Comprehensive webhook management with GUI
- Amasty Webhooks: Supports multiple events and custom payloads
- Webkul Webhooks: Simple setup for common integrations
Final Thoughts
Webhooks transform how your Magento 2 store communicates with other systems. By implementing real-time notifications, you eliminate delays, reduce manual work, and create a more responsive ecommerce ecosystem.
Start with simple webhooks for critical events like new orders, then expand to other business processes as you become comfortable with the technology. Remember to implement proper error handling, security, and monitoring from the beginning.
Have you implemented webhooks in your Magento store? What challenges did you face? Share your experiences in the comments!