How to Implement a Custom Tax Calculation Logic in Magento 2
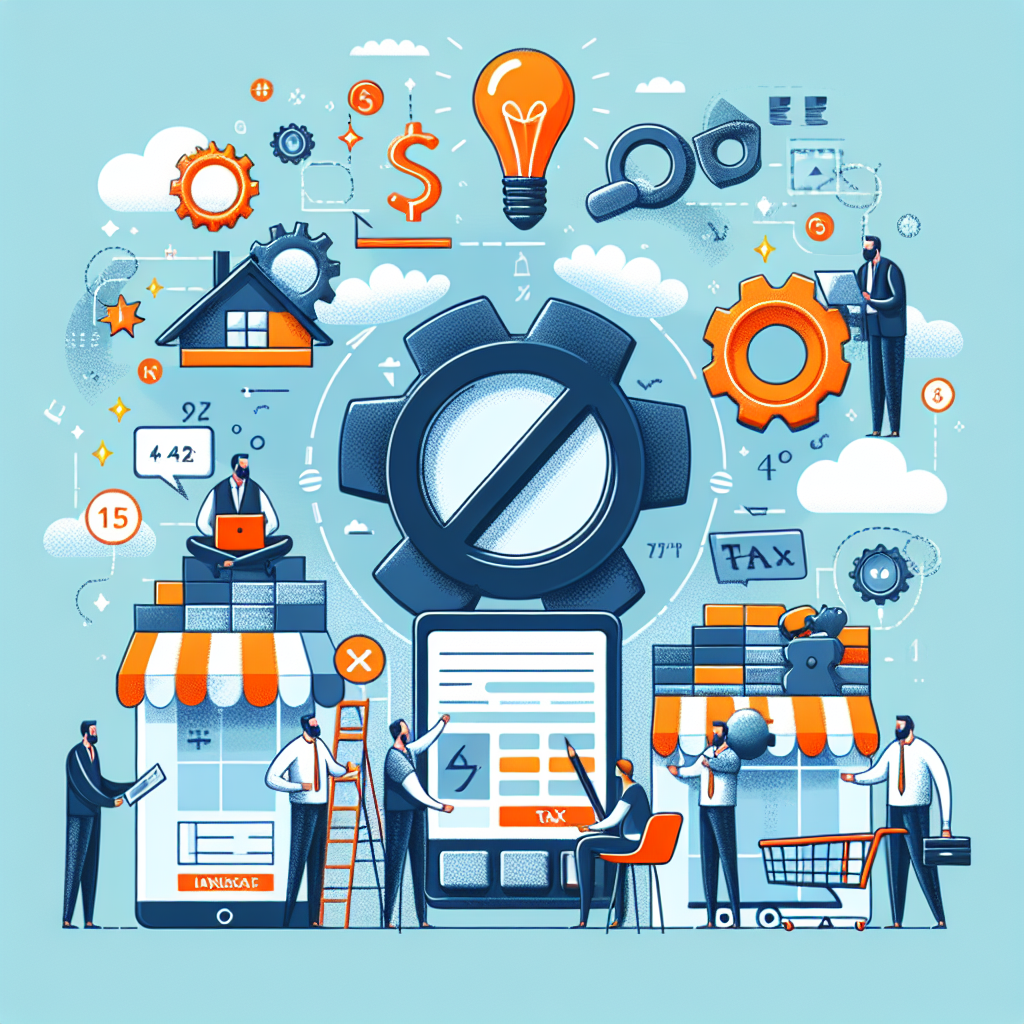
Understanding Magento 2 Tax Calculation
Magento 2 comes with a robust tax calculation system out of the box, but sometimes your business rules require something more tailored. Whether you need to apply special tax rates for specific customer groups, products, or regions, implementing custom tax logic is the way to go.
Before diving into code, let's clarify how Magento handles taxes:
- Tax rules are applied based on customer tax class, product tax class, and tax rates
- The system calculates taxes during checkout and order creation
- All calculations are handled by the
Magento\Tax\Model\Calculation
model
When to Implement Custom Tax Logic
You might need custom tax calculation in scenarios like:
- Applying different tax rates for wholesale vs retail customers
- Special tax exemptions for certain product categories
- Complex regional tax rules (like county-level taxes in some US states)
- Dynamic tax rates based on cart contents or customer attributes
Step 1: Create a Tax Calculation Plugin
The cleanest way to modify tax behavior is by creating a plugin for the calculation model. Here's how:
<?php
namespace YourCompany\Tax\Plugin;
class TaxCalculationPlugin
{
public function aroundCalculate(
\Magento\Tax\Model\Calculation $subject,
callable $proceed,
$rateRequest
) {
// Your custom logic before original calculation
if ($this->shouldApplyCustomTax($rateRequest)) {
return $this->calculateCustomTax($rateRequest);
}
// Call original method if no custom tax applies
return $proceed($rateRequest);
}
private function shouldApplyCustomTax($rateRequest)
{
// Add your conditions here
// Example: Check customer group or product type
return false;
}
private function calculateCustomTax($rateRequest)
{
// Implement your custom calculation
return 8.25; // Example fixed rate
}
}
Step 2: Register Your Plugin
Create or modify your module's etc/di.xml
:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Tax\Model\Calculation">
<plugin name="yourcompany_tax_calculation" type="YourCompany\Tax\Plugin\TaxCalculationPlugin"/>
</type>
</config>
Step 3: Testing Your Implementation
Always test your custom tax logic thoroughly:
- Create test orders with different customer groups
- Verify tax calculations in the admin panel
- Check tax amounts during checkout
- Test with various product combinations
Alternative Approach: Custom Tax Rate Model
For more complex scenarios, you might need to create a custom tax rate model:
<?php
namespace YourCompany\Tax\Model;
class CustomRate extends \Magento\Tax\Model\Calculation\Rate
{
public function getRate($request)
{
$standardRate = parent::getRate($request);
// Apply your modifications
if ($this->isWeekend()) {
return $standardRate * 1.1; // Weekend surcharge
}
return $standardRate;
}
private function isWeekend()
{
return date('N') >= 6;
}
}
Handling Tax Display
Don't forget about tax display settings. You might need to adjust how taxes are shown:
<?php
namespace YourCompany\Tax\Plugin;
class TaxDisplayPlugin
{
public function afterGetPriceDisplayType(
\Magento\Tax\Helper\Data $subject,
$result
) {
// Force including tax in prices for specific customer group
if ($this->isWholesaleCustomer()) {
return \Magento\Tax\Model\Config::DISPLAY_TYPE_INCLUDING_TAX;
}
return $result;
}
}
Performance Considerations
Custom tax logic can impact performance. Keep these tips in mind:
- Cache tax rates when possible
- Minimize database queries in your tax calculations
- Consider using indexes for customer/product tax class lookups
- Profile your tax calculations during peak loads
Common Pitfalls to Avoid
Watch out for these common mistakes:
- Not handling tax rounding properly (Magento uses 4 decimal places internally)
- Forgetting to account for shipping tax calculations
- Not testing with all price display settings (including/excluding tax)
- Overriding core tax models instead of using plugins
Extending with Custom Tax Rules
For ultimate flexibility, consider creating custom tax rule types:
<?php
namespace YourCompany\Tax\Model\Rule;
class VolumeBased extends \Magento\Tax\Model\Calculation\Rule\AbstractRule
{
public function calculate(\Magento\Tax\Service\V1\Data\QuoteDetails $quoteDetails)
{
// Implement volume-based tax rules
// Example: Higher tax rate for orders over $1000
}
}
Final Thoughts
Custom tax logic in Magento 2 gives you the power to handle complex business requirements while maintaining the robustness of the core tax system. Whether you need simple rate adjustments or completely custom tax rules, the plugin system provides a maintainable way to implement your requirements.
Remember to:
- Always extend functionality rather than modifying core files
- Document your custom tax rules clearly
- Test with real-world scenarios before deployment
- Monitor performance after implementation
Need help with your Magento 2 tax implementation? Check out our Magento extensions or hosting solutions to ensure your store runs smoothly with custom tax logic.