How to Use Magento 2’s Built-in Testing Frameworks (PHPUnit, MFTF)
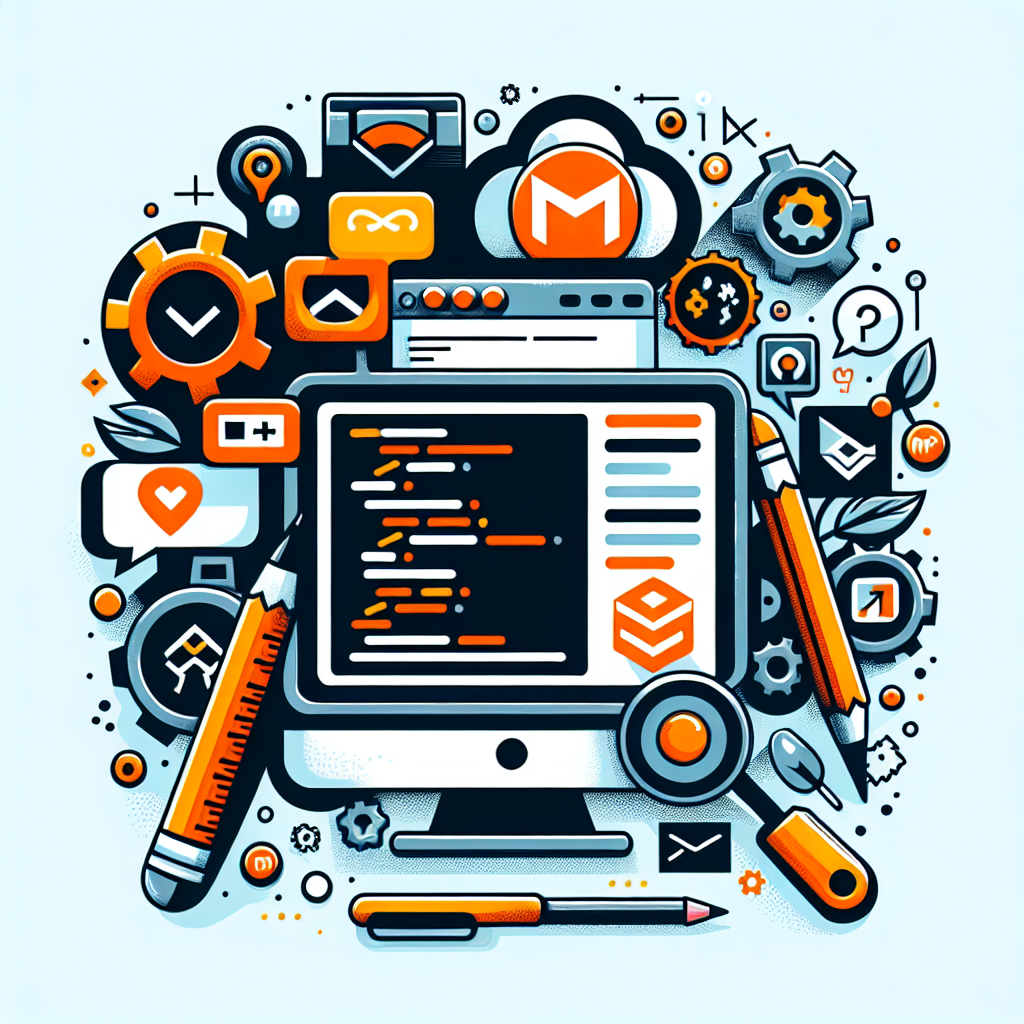
Understanding Magento 2's Testing Frameworks
Testing is a crucial part of any Magento 2 development workflow. Luckily, Magento comes with two powerful built-in testing frameworks: PHPUnit for unit and integration testing, and MFTF (Magento Functional Testing Framework) for functional testing. Let's break down how to use them effectively.
Setting Up PHPUnit for Magento 2
PHPUnit is your go-to tool for testing individual components of your Magento store. Here's how to get started:
- First, make sure you have PHPUnit installed. If you're using Composer (which you should be), run:
composer require --dev phpunit/phpunit
- Create your first test class in the
Test/Unit
directory of your module. Here's a basic example:
<?php
namespace Vendor\Module\Test\Unit;
use PHPUnit\Framework\TestCase;
class SimpleTest extends TestCase
{
public function testAddition()
{
$this->assertEquals(4, 2+2);
}
}
- Run your test from the Magento root directory:
vendor/bin/phpunit Vendor/Module/Test/Unit/SimpleTest.php
Writing Integration Tests
Integration tests verify how your code interacts with Magento's core components. Here's a sample integration test:
<?php
namespace Vendor\Module\Test\Integration;
use Magento\TestFramework\TestCase\AbstractController;
class ProductTest extends AbstractController
{
public function testProductPageLoads()
{
$this->dispatch('catalog/product/view/id/1');
$this->assertContains('Simple Product', $this->getResponse()->getBody());
}
}
Getting Started with MFTF
The Magento Functional Testing Framework (MFTF) is designed for end-to-end testing of store functionality. Here's how to set it up:
- Install MFTF dependencies:
composer require --dev magento/magento2-functional-testing-framework
- Generate the initial configuration:
vendor/bin/mftf generate:config
Creating Your First MFTF Test
MFTF tests are written in XML. Here's a simple test that verifies a customer can log in:
<?xml version="1.0" encoding="UTF-8"?>
<tests xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:mftf:Test/etc/testSchema.xsd">
<test name="CustomerLoginTest">
<annotations>
<title value="Customer Login Test"/>
<description value="Verify customer can login"/>
</annotations>
<before>
<createData entity="customer" stepKey="createCustomer"/>
</before>
<amOnPage url="{{StorefrontCustomerSignInPage.url}}" stepKey="goToSignInPage"/>
<fillField selector="{{StorefrontCustomerSignInFormSection.emailField}}"
userInput="$$createCustomer.email$$" stepKey="fillEmail"/>
<fillField selector="{{StorefrontCustomerSignInFormSection.passwordField}}"
userInput="$$createCustomer.password$$" stepKey="fillPassword"/>
<click selector="{{StorefrontCustomerSignInFormSection.signInAccountButton}}"
stepKey="clickSignIn"/>
<see userInput="Welcome, $$createCustomer.firstname$$ $$createCustomer.lastname$$!"
selector="{{StorefrontCustomerDashboardSection.welcomeMessage}}"
stepKey="seeWelcomeMessage"/>
<after>
<deleteData createDataKey="createCustomer" stepKey="deleteCustomer"/>
</after>
</test>
</tests>
Running MFTF Tests
To execute your MFTF tests, use:
vendor/bin/mftf run:test CustomerLoginTest
Best Practices for Magento 2 Testing
- Write tests before or alongside your code (TDD approach)
- Keep tests focused on one specific functionality
- Use meaningful test names and annotations
- Clean up test data after each test
- Run tests frequently during development
Debugging Failed Tests
When tests fail, Magento provides several tools to help diagnose issues:
- For PHPUnit tests, use
--debug
flag - For MFTF, check the
var/log/mftf.log
file - Take screenshots on failure with MFTF by adding
<saveScreenshot stepKey="saveScreenshot"/>
Integrating Tests into Your CI/CD Pipeline
To make testing part of your deployment process, add these commands to your CI configuration:
# Run unit tests
vendor/bin/phpunit
# Run integration tests
vendor/bin/phpunit -c dev/tests/integration/phpunit.xml.dist
# Run MFTF tests
vendor/bin/mftf build:project
vendor/bin/mftf run:group default
Advanced MFTF Techniques
Once you're comfortable with basic MFTF tests, try these advanced features:
- Data entities for reusable test data
- Test suites to group related tests
- Page objects for better maintainability
- Custom actions for complex test scenarios
Common Pitfalls and How to Avoid Them
- Flaky tests: Make sure your tests don't rely on timing or external services
- Slow tests: Use mocked data where possible
- Overly complex tests: Keep tests simple and focused
- Not testing edge cases: Include tests for error conditions
By mastering these testing frameworks, you'll significantly improve the quality and reliability of your Magento 2 store. Happy testing!